Question
How do I update my summary function to include a count of A, B, 100, 0s this is my current code: #globals students = {}
How do I update my summary function to include a count of A, B, 100, 0s
this is my current code:
#globals
students = {} #stores complete information about each student in a dictionary
#key: name value: [score, grade_basis, grade] score = value[0] grade_basis = value[1] grade = value[2]
#functions
def is_valid_graded(grade_basis): #validation bool
return grade_basis == 1 or grade_basis == 0
def is_valid_score(score): #in range 0...100 validation bool
return score >=0 and score
def compute_grade(score): #computes grades based on score and return grade
if score > 80:
grade = 'A'
else:
grade = 'B'
return grade
def compute_passfail(score,grade_basis):
if grade_basis == 1:
return compute_grade(score)
if score >=40:
grade = "Pass"
else:
grade = "Fail"
return grade
def submit():
global students
line = input('Enter name, score and grade basis (1 or 0)>>')
in_list = line.split()
name = in_list[0]
score = float(in_list[1])
if not is_valid_score(score): #validating score here
print('Error! Score must be positive')
return
print(score)
grade_basis = int(in_list[2])
if not is_valid_graded(grade_basis):
print('Error! Grade basis is defined by entering 0 or 1') #validating grade basis here
return
print(grade_basis)
grade = compute_passfail(score,grade_basis)
students[name] = [score,grade_basis,grade] #inserting record into dictionary
print(name,score,grade_basis,grade)
def compute_average_score():
global students
total_score = 0.0
num_inputs = 0
grade_basis_count = 0
for value in students.values():
score = value[0]
grade_basis = value[1]
grade = value[2]
total_score = total_score + score
num_inputs = num_inputs + 1
if grade_basis:
grade_basis_count = grade_basis_count + 1
if num_inputs > 0:
avg = total_score / num_inputs
else:
avg = None
return avg, num_inputs,grade_basis_count
def summary():
global students
average_score, num_inputs,grade_basis_count = compute_average_score()
if average_score is not None:
print(f'Average Score: {average_score:.2f}\tNumber of Inputs: {num_inputs:d}\tGrade Basis Count: {grade_basis_count:d}')
else:
print('No Data!')
def clear_data():
global students
students.clear()
def reset():
clear_data()
def display():
global students
if students:
for key in students.keys():
value = students[key]
score = value[0]
grade_basis = value[1]
grade = value[2]
print(f'{key:12}|{score:12}|{grade_basis:14}|{grade:12}|')
else:
print('No data!')
def search():
global students
key = input('Enter a name to search for?>>')
if key in students.keys():
value = students[key]
score = value[0]
grade_basis = value[1]
grade = value[2]
print(f'{key:12}|{score:12}|{grade_basis:14}|{grade:12}|')
else:
print('No Key Found!')
#main
quit = False
while not quit:
print('1.Submit 2.Summary 3.Reset 4.Display 5.Search 6.Exit')
choice = int(input('Enter choice: '))
if choice == 1:
submit()
elif choice == 2:
summary()
elif choice == 3:
reset()
print('Data Clear. Ready for new series')
elif choice == 4:
display()
elif choice == 5:
search()
elif choice == 6:
quit = True
clear_data()
else:
print('Invalid Choice!')
summary() uses a compute_average function. Summary displays: Average score, number of inputs, average score for inputs on grade basis, count of A, B, 100s, Os. Same restrictions as in previous version (no built-in functions to be used for sum() or len(), and no additional global variables besides the dictionary)Step by Step Solution
There are 3 Steps involved in it
Step: 1
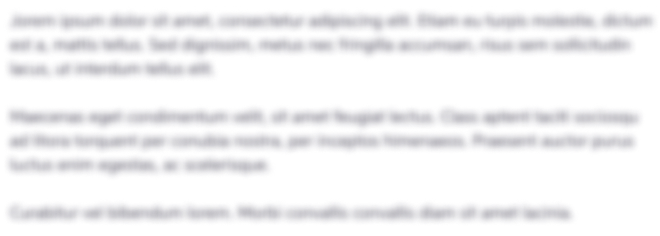
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started