Question
How do you write the code in Java for class named EmployeeManagerFileImpl implements EmployeeManager ? I also need to write a Test Harness that Tests
How do you write the code in Java for class named EmployeeManagerFileImpl implements EmployeeManager ?
I also need to write a Test Harness that Tests the program
/** * */ class named EmployeeManagerFileImpl implements EmployeeManager {
EmployeeManagerFileImpl should use a CSV File to store and read the data. method: createEmployee Should meet the requirements of the Interface update the Employee.Id to have the index value add the Employee to the file. method: updateEmployee Should meet the requirements of the Interface update the employee in the File method: readEmployee Should meet the requirements of the Interface method: readAllEmployees Should meet the requirements of the Interface A method deleteEmployee Should meet the requirements of the Interface
} /** Create a TestHarness
Test Harness Example
Create a TestHarness Simar to the example in Figure 6.7.1
Use the same format at the Test Program in EmployeeManager Interface Part 1.
This time Test both the EmployeeManagerArrayListImpl and EmployeeManagerFileImpl
After each statement there should be a statement that verifies the change
Example:
Write a test program that creates an Employee -- firstName: John, lastName: Wayne, payPerHour: 100.0, EmployeeType: CONTRACTOR Call the EmployeeManager.createEmployee
Employee employeeFile = new Employee(0,"John", "Wayne", 100.0, EmployeeType.CONTRACTOR)); Employee employeeArrayList = new Employee(0,"John", "Wayne", 100.0, EmployeeType.CONTRACTOR)); EmployeeManager employeeManagerArrayList = new EmployeeManagerArrayListImpl(); EmployeeManager employeeManagerFile = new EmployeeManagerFile(); employeeManagerFile.createEmployee(employeeFile); employeeFile = employeeManagerFile.readEmployee(1); System.out.printf("%s, expecting 1, got %s ",1 == employeeFile.getId(), employeeFile.getId()); System.out.printf("%s, expecting John, got %s ","John".equals(employeeFile.getFirstName()), employeeFile.getFirstName()); System.out.printf("%s, expecting Wayne, got %s ","Wayne".equals(employeeFile.getLastName()), employeeFile.getLastName()); System.out.printf("%s, expecting 100, got %s ",100 == employeeFile.getPayPerHour(), employeeFile.getPayPerHour()); System.out.printf("%s, expecting CONTRACTOR, got %s ", EmployeeType.CONTRACTOR.equals(employeeFile.getEmployeeType()), employeeFile.getEmployeeType()); employeeManagerArrayList.createEmployee(employeeArrayList); employeeArrayList = employeeManagerArrayList.readEmployee(1); System.out.printf("%s, expecting 1, got %s ",1 == employeeArrayList.getId(), employeeArrayList.getId()); System.out.printf("%s, expecting John, got %s ","John".equals(employeeArrayList.getFirstName()), employeeArrayList.getFirstName()); System.out.printf("%s, expecting Wayne, got %s ","Wayne".equals(employeeArrayList.getLastName()), employeeArrayList.getLastName()); System.out.printf("%s, expecting 100, got %s ",100 == employeeArrayList.getPayPerHour(), employeeArrayList.getPayPerHour()); System.out.printf("%s, expecting CONTRACTOR, got %s ", EmployeeType.CONTRACTOR.equals(employeeArrayList.getEmployeeType()), employeeArrayList.getEmployeeType());
//Employee.java
package examplesweek2; /** * * @author * */
public class Employee { private int id; private double paymentPerHour; private String firstName; private String lastName; private EmployeeType employeeType;
//Constructor Employee for all private fields public Employee(int id, double paymentPerHour, String firstName, String lastName, EmployeeType employeeType) { super(); this.id = id; this.paymentPerHour = paymentPerHour; this.firstName = firstName; this.lastName = lastName; this.employeeType = employeeType; }
public int getId() { return id; } public void setId(int id) { this.id = id; }
public double getPaymentPerHour() { return paymentPerHour; }
public void setPaymentPerHour(double paymentPerHour) { this.paymentPerHour = paymentPerHour; }
public String getFirstName() { return firstName; }
public void setFirstName(String firstName) { this.firstName = firstName; }
public String getLastName() { return lastName; }
public void setLastName(String lastName) { this.lastName = lastName; }
public EmployeeType getEmployeeType() { return employeeType; }
public void setEmployeeType(EmployeeType employeeType) { this.employeeType = employeeType; }
public double calculateWeeklySalary() { return paymentPerHour * 40; }
@Override public String toString() { return "Employee [id=" + id + ", paymentPerHour=" + paymentPerHour + ", firstName=" + firstName + ", lastName=" + lastName + ", employeeType=" + employeeType + "]"; } //EmployeeManager.java
package examplesweek2;
import java.util.List; /** * * @author * */
//EmployeeManagerArrayListImpl.java
package examplesweek2;
import java.util.ArrayList; import java.util.List; /** * * @author * */
public class EmployeeManagerArrayListImpl implements EmployeeManager {
/** EmployeeManagerArrayListImpl should use an ArrayList to store the data method: createEmployee Should meet the requirements of the Interface add the Employee to the ArrayList. update the Employee.Id to have the index value in the ArrayList method: updateEmployee Should meet the requirements of the Interface update the employee in the ArrayList method: readEmployee Should meet the requirements of the Interface method: readAllEmployees Should meet the requirements of the Interface A method deleteEmployee Should meet the requirements of the Interface */ private List
public Employee updateEmployee(Employee employee) { //update Employee in ArrayList if(employee.getId() == 0) { System.out.println("To add an Employee the Id must not be zero");; return null; } for (int i = 0; i < employeeList.size(); i++) { Employee emp = employeeList.get(i); if (employee.getId() == emp.getId()) { employeeList.set(i, employee); } } return employee; } @Override public Employee readEmployee(int employeeId) { for (int i = 0; i < employeeList.size(); i++) { Employee emp = employeeList.get(i); if (employeeId == emp.getId()) { return emp; } } return null; } public List
public void deleteEmployee(int employeeid) { Employee employee = readEmployee(employeeid); employeeList.remove(employee); } }
public interface EmployeeManager {
public Employee createEmployee(Employee employee); public Employee updateEmployee(Employee employee); public Employee readEmployee(int id); public List
}
// EmployeeType.java
package examplesweek2; /** * * @author * */
public enum EmployeeType {
//2 values CONTRACTOR, FULL_TIME CONTRACTOR, FULL_TIME }
//Employee Test Program
package inclass.week.two;
import java.util.List;
public class EmployeeTestProgram {
public static void main(String[] args) { Employee employee = new Employee(0, 100, "John", "Wayne", EmployeeType.CONTRACTOR); EmployeeManager employeeManager = new EmployeeManagerArrayListImpl(); employeeManager.createEmployee(employee); Employee readEmployee = employeeManager.readEmployee(employee.getId()); printEmployee(readEmployee); Employee nullEmployee = employeeManager.readEmployee(2); if (nullEmployee == null) { System.out.println("Employee.id 2: not found"); } readEmployee.setFirstName("Bruce"); readEmployee.setPaymentPerHour(200.0); readEmployee.setEmployeeType(EmployeeType.FULL_TIME); Employee updatedEmployee = employeeManager.updateEmployee(readEmployee); printEmployee(updatedEmployee); Employee employee2 = new Employee(0, 1000.0, "Selina", "Kyle", EmployeeType.CONTRACTOR); employeeManager.createEmployee(employee2); List
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
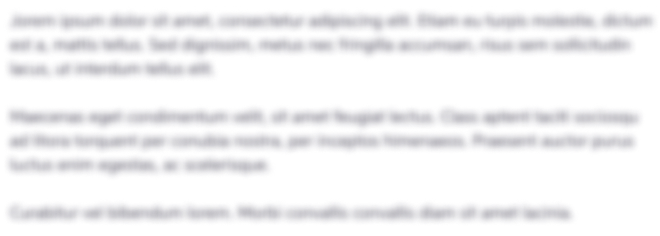
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started