Question
How to achieve this function? (Pointed out by red line) When we type anything except integer, the sys would show: Must be an integer The
How to achieve this function? (Pointed out by red line)
When we type anything except integer, the sys would show: "Must be an integer"
The code is also showing below in text.
import random
from sqlite3 import IntegrityError
from tkinter.tix import INTEGER
class TicTacToe:
def __init__(self): # Use as is
""" initializes data fields (board and played)
and prints the banner messages
and prints the initial board on the screen
"""
self.board = [' '] * 9 # A list of 9 strings, one for each cell,
# will contain ' ' or 'X' or 'O'
self.played = set() # Set (of cell num) already played: to keep track of the played cells
print("Welcome to Tic-Tac-Toe!")
print("You play X (first move) and computer plays O.")
print("Computer plays randomly, not strategically.")
self.printBoard()
def printBoard(self) -> None:
""" prints the board on the screen based on the values in the self.board data field """
print() # Print the top blank line
# Print the rows 1-3
# this is the first row
print(self.board[0] + " | " + self.board[1] + " | " + self.board[2])
# first row border
print("--+---+--")
# second row
print(self.board[3] + " | " + self.board[4] + " | " + self.board[5])
# second row border
print("--+---+--")
# third row
print(self.board[6] + " | " + self.board[7] + " | " + self.board[8])
# third row border
print()
def playerNextMove(self) -> None:
""" prompts the player for a valid cell number;
error checks that the input is a valid cell number;
and prints the info and the updated self.board;
"""
# Prompt the player for a cell number using input()
while True: # Using infinite loop
try: # Using try and except to check if the input is a valid cell number
cell = int(input("> Next move for X (state a vaild cell num): ")) # Prompt the player for a cell number using input()
if cell 8: # Check if the input is a valid cell number
raise ValueError # If the input is not a valid cell number, raise a ValueError
if cell in self.played: # Check if the input is a valid cell number
raise ValueError # If the input is not a valid cell number, raise a ValueError
self.board[cell] = 'X' # If the input is a valid cell number, update the board
self.played.add(cell) # Add the input to the played set
self.printBoard() # Print the updated board
break # Break out of the loop
except ValueError: # If the input is not a valid cell number, print an error message
print("Must enter a valid cell number") # Print an error message
continue # Continue the loop
def computerNextMove(self) -> None:
""" computer randomly chooses a valid cell,
and prints the info and the updated self.board
"""
while True: # using infinite Loop
cell = random.randint(0, 8) # Generate a random number between 0 and 8
if cell in self.played: # Check if the input is a valid cell number
continue # If the input is not a valid cell number, continue the loop
self.board[cell] = 'O' # If the input is a valid cell number, update the board
self.played.add(cell) # Add the input to the played set
self.printBoard() # Print the updated board
break # Break out of the loop
def hasWon(self, who: str) -> bool:
""" returns True if who (being passed 'X' or 'O') has won, False otherwise """
# Check rows
if self.board[0] == 'X' and self.board[1] == 'X' and self.board[2] == 'X': # Check row 1
return True # Return True if the row is won
if self.board[3] == 'X' and self.board[4] == 'X' and self.board[5] == 'X': # Check row 2
return True # Return True if the row is won
if self.board[6] == 'X' and self.board[7] == 'X' and self.board[8] == 'X': # Check row 3
return True # Return True if the row is won
if self.board[0] == 'X' and self.board[3] == 'X' and self.board[6] == 'X': # Check column 1
return True # Return True if the column is won
if self.board[1] == 'X' and self.board[4] == 'X' and self.board[7] == 'X': # Check column 2
return True # Return True if the column is won
if self.board[2] == 'X' and self.board[5] == 'X' and self.board[8] == 'X': # Check column 3
return True # Return True if the column is won
if self.board[0] == 'X' and self.board[4] == 'X' and self.board[8] == 'X': # Check diagonal 1
return True # Return True if the diagonal is won
if self.board[2] == 'X' and self.board[4] == 'X' and self.board[6] == 'X': # Check diagonal 2
return True
else:
return False # Return False if the diagonal is not won
def hasLost(self, who: str) -> bool:
""" returns True if computer (playing 'O') has won, False otherwise """
# Check rows
if self.board[0] == 'O' and self.board[1] == 'O' and self.board[2] == 'O': # Check row 1
return True # Return True if the row is lost
if self.board[3] == 'O' and self.board[4] == 'O' and self.board[5] == 'O': # Check row 2
return True # Return True if the row is lost
if self.board[6] == 'O' and self.board[7] == 'O' and self.board[8] == 'O': # Check row 3
return True # Return True if the row is lost
if self.board[0] == 'O' and self.board[3] == 'O' and self.board[6] == 'O': # Check column 1
return True # Return True if the column is lost
if self.board[1] == 'O' and self.board[4] == 'O' and self.board[7] == 'O': # Check column 2
return True # Return True if the column is lost
if self.board[2] == 'O' and self.board[5] == 'O' and self.board[8] == 'O': # Check column 3
return True # Return True if the column is lost
if self.board[0] == 'O' and self.board[4] == 'O' and self.board[8] == 'O': # Check diagonal 1
return True # Return True if the diagonal is lost
if self.board[2] == 'O' and self.board[4] == 'O' and self.board[6] == 'O': # Check diagonal 2
return True
else:
return False # Return False if the diagonal is not lost
def terminate(self, who: str) -> bool:
""" returns True if who (being passed 'X' or 'O') has won or if it's a draw, False otherwise;
it also prints the final messages:
"You won! Thanks for playing." or
"You lost! Thanks for playing." or
"A draw! Thanks for playing."
"""
if self.hasWon(who): # Check if the player has won
print("You won! Thanks for playing.") # Print the message
return True # Return True if the player has won
if self.hasLost(who):
print("You lost! Thanks for playing.")
return True
if len(self.played) == 9: # Check if the game is a draw
print("A draw! Thanks for playing.") # Print the message
return True # Return True if the game is a draw
return False # Return False if the game is not a draw or won
if __name__ == "__main__": # Use as is
ttt = TicTacToe() # initialize a game
while True:
ttt.playerNextMove() # X starts first
if (ttt.terminate('X')): break # if X won or a draw, print message and terminate
ttt.computerNextMove() # computer plays O
if (ttt.terminate('O')): break # if O won or a draw, print message and terminate
Welcome to Tic-Tac-Toe! You play X (first move) and computer plays 0. Computer plays randomly, not strategically. 0 | 1 | 2 --+---+-- 1 3 | 4 | 5 --+---+-- 6 | 7 | 8 > Next move for X (state a valid cell num): The move is stated by the number of a valid cell. If the number is not valid (already taken, or not between 0 and 8) or if it is not a number, the game should display the error message (as shown) and repeat asking for the next move. > Next move for x (state a valid cell num) Must be an integer > Next move for X (state a valid cell num): 13 Must enter a valid cell number > Next move for X (state a valid cell num): 4 You chose cell 4 0 | 1 | 2 +---+- --+---+-- 3 | 4 | 5 --+---+-- 6 | 7 | 8 Computer chose cell 5 xo 0 | 1 | 2 --+---+-- | 4 | 5 --+---+-- 6 | 7 | 8 > Next move for X (state a valid cell num): 1 # End of printBoardo 6 7 def playerNextMove(self) -> None: prompts the player for a valid cell number; error checks that the input is a valid cell number; and prints the info and the updated self.board; 9 1 2 3 4 5 6 7 3 # Prompt the player for a cell number using input() while True: # Using infinite loop try: # Using try and except to check if the input is a valid cell number cell = int(input("> Next move for X (state a vaild cell num): ")) # Prompt the player for a cell number using input() if cell 8: # Check if the input is a valid cell number raise ValueError # If the input is not a valid cell number, raise a ValueError if cell in self.played: # Check if the input is a valid cell number raise ValueError # If the input is not a valid cell number, raise a ValueError self.board[cell] = 'X' # If the input is a valid cell number, update the board self.played.add(cell) # Add the input to the played set self.printBoard() # Print the updated board break # Break out of the loop except ValueError: # If the input is not a valid cell number, print an error message print("Must enter a valid cell number") # Print an error message continue # Continue the loop 3 1 2 3 4 5 6 7 8 def computer NextMove(self) -> None: computer randomly chooses a alid cell, and prints the info and the updated self.board HER 3 1 2 3 4 5 6 7 3 while True: # using infinite Loop cell = random.randint(0, 8) # Generate a random number between 0 and 8 if cell in self.played: # Check if the input is a valid cell number continue # If the input is not a valid cell number, continue the loop self.board[cell] = '0' # If the input is a valid cell number, update the board self.played.add(cell) # Add the input to the played set self.printBoard() # Print the updated board break # Break out of the loopStep by Step Solution
There are 3 Steps involved in it
Step: 1
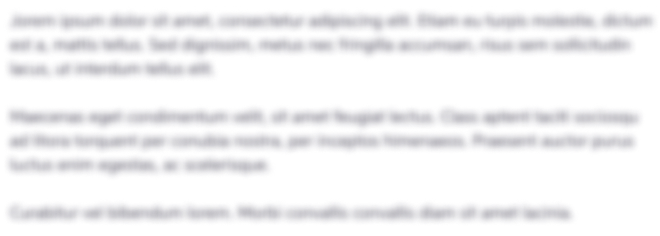
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started