Question
How to complete this problem in python. can you send the code which I can copy and paste, please? thank you. In this assignment, we
How to complete this problem in python. can you send the code which I can copy and paste, please? thank you.
In this assignment, we will sort cards again, but this time we will be using quicksort and the suit matters. The cards should be sorted by rank and any cards with the same rank should then be sorted by suit. You can use a helper function to compare cards, or, if you'd like, you can implement the method __lt__(self, other) and then directly compare cards (e.g. card1 < card2 will call card1.__lt__(card2)). For more information on defining built-in operators, see https://docs.python.org/3/library/operator.html. The ranking of suits, from low to high, should be Clubs, Diamonds, Spades, Hearts. The suits will be stored as strings starting with capitol letters, as written above. This means that the 2 of Clubs should come before the 2 of Diamonds in the sorted result. Unlike problem 2, Aces are always high. You are required to use quicksort in this problem. I don't mind which version of pivot selection or partitioning you use. You will not get full credit for a solution which runs in O(n^2) time. If it will help you, you may redefine the constants JACK, QUEEN, KING, and ACE. However, they must exist as I will use them in my test case. Example code: cards = [ Card(2), Card(KING), Card(8), Card(ACE), Card(5), ] sort_cards(cards) => [2 of Spades, 5 of Spades, 8 of Spades, King of Spades, Ace of Spades] suited_cards = [ Card(ACE, 'Hearts'), Card(QUEEN, 'Spades'), Card(8, 'Clubs'), Card(ACE, 'Clubs'), Card(QUEEN, 'Diamonds'), ] sort_cards(suited_cards) => [8 of Clubs, Queen of Diamonds, Queen of Spades, Ace of Clubs, Ace of Hearts] aces = [ Card(ACE, 'Hearts'), Card(ACE, 'Diamonds'), Card(ACE, 'Clubs'), Card(ACE, 'Spades'), ] sort_cards(suited_cards) => [Ace of Clubs, Ace of Diamonds, Ace of Spades, Ace of Hearts]
class Card: def __init__(self, rank, suit = 'Spades'): """Initialize the card with an optional suit (default: Spades).""" self.__rank = rank self.__setSuit(suit) def __setSuit(self, suit): if suit not in {'Hearts', 'Spades', 'Diamonds', 'Clubs'}: raise ValueError(f'Improper suit passed to Card(): {suit}') self.__suit == suit def getSuit(self): """Return the suit.""" return self.__suit def getRank(self): """Return the rank.""" return self.__rank def printRank(self): """Return a printable version of the rank.""" names = {JACK: 'Jack', QUEEN: 'Queen', KING: 'King', ACE: 'Ace'} return names.get(self.__rank, self.__rank) def __repr__(self): """Return the printable version of the card.""" return f'{self.printRank()} of {self.__suit}' def __eq__(self, other): """Check equality between cards by attributes.""" return self.__rank == other.__rank and self.__suit == other.__suit
JACK = 11 QUEEN = 12 KING = 13 ACE = 1
def quicksort_cards(cards): """Sort a hand of cards using quicksort.""" # YOUR CODE HERE
Step by Step Solution
There are 3 Steps involved in it
Step: 1
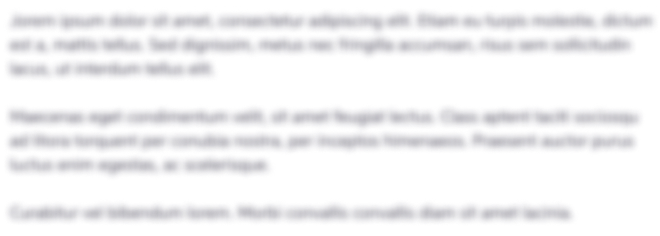
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started