Question
How to fix my code so that the GUI looks like in the photos? import javafx.application.*; import javafx.event.*; import javafx.scene.*; import javafx.scene.control.*; import javafx.scene.control.Alert.*; import
How to fix my code so that the GUI looks like in the photos?
import javafx.application.*; import javafx.event.*; import javafx.scene.*; import javafx.scene.control.*; import javafx.scene.control.Alert.*; import javafx.scene.layout.*; import javafx.scene.text.*; import javafx.stage.*; import javafx.stage.FileChooser.*; import javafx.geometry.*; import javafx.collections.*; import java.net.*; import java.io.*; import java.util.*;
public class OrderClient extends Application{
//window attributes private Stage stage; private Scene scene; private VBox root = null;
//dropdown list /*public static final ObservableList state = FXCollections.observableArrayList( "AL", "AK", "AZ", "AR", "CA", "CO", "CT", "DE", "DC", "FL", "GA", "HI","ID", "IL", "IN", "IA", "KS", "KY", "LA", "ME", "MD", "MA", "MI", "MN", "MS", "MO", "MT", "NE", "NV", "NH", "NJ", "NM", "NY", "NC", "ND", "OH", "OK", "OR", "PA", "RI", "SC", "SD", "TN", "TX", "UT", "VT", "VA", "WA", "WV", "WI", "WY"); state.addListener (new ListChangeListener() { //override the method here }*/ // Components - TOP // The TOP will itself be GridPane - we will use Row1 and Row2 of this Grid // These are for Row1 private Label lblServerIP = new Label("Server: "); private TextField tfServerIP = newTextField(); private Label lblName = new Label(" Name: "); private TextField tfName = new TextField(); private Label lblAddress = new Label(" Address: "); private Label lblStreet = new Label(" Street: "); private TextField tfStreet = new TextField(); private Label lblCity = new Label(" City: "); private TextField tfCity = new TextField(); private Label lblZipcode = new Label(" Zipcode: "); private TextField tfZipcode = new TextField(); private Label lblEmail = new Label(" Email: "); private TextField tfEmail = new TextField(); private Label lblItemNum = new Label(" Item Num: "); private TextField tfItemNum = new TextField(); private Label lblQuantity = new Label(" Quantity: "); private TextField tfQuantity = new TextField(); private Button btnNew = new Button("New Order"); private Button btnNum = new Button("Number of Current Orders"); private Button btnExit = new Button("Disconnect"); // IO attributes private PrintWriter pwt = null; private Scanner scn = null; private ObjectOutputStream out = null;
//general SOCKET attributes public static final int SERVER_PORT = 32001; private Socket socket = null; // IO attributes private DataOutputStream dos = null; // Stream TO server private DataInputStream dis = null; // Stream FROM server private ObjectOutputStream oos = null; private ObjectInputStream ois = null;
public static void main(String [] args) { launch(args); } public void start(Stage _stage) { stage = _stage; stage.setTitle("Place an Order"); stage.setOnCloseRequest( new EventHandler() { public void handle(WindowEvent evt) { System.exit(0); } }); stage.setResizable(false); root = new VBox(8); // ROW1 - FlowPane FlowPane fpRow1 = new FlowPane(8,8); fpRow1.setAlignment(Pos.CENTER); fpRow1.getChildren().addAll(btnNew, btnNum, btnExit); root.getChildren().add(fpRow1); //FlowPane FlowPane fpRow2 = new FlowPane(8,8); tfName.setPrefColumnCount(20); fpRow2.getChildren().addAll(lblName, tfName); root.getChildren().add(fpRow2); //FlowPane FlowPane fpRow3 = new FlowPane(8,8); fpRow3.getChildren().addAll(lblAddress); root.getChildren().add(fpRow3); //FlowPane FlowPane fpRow4 = new FlowPane(8,8); tfStreet.setPrefColumnCount(20); tfCity.setPrefColumnCount(8); fpRow4.getChildren().addAll(lblStreet, tfStreet, lblCity, tfCity); root.getChildren().add(fpRow4); //FlowPane FlowPane fpRow5 = new FlowPane(8,8); tfZipcode.setPrefColumnCount(8); fpRow5.getChildren().addAll(lblZipcode, tfZipcode); root.getChildren().add(fpRow5); //FlowPane FlowPane fpRow6 = new FlowPane(8,8); tfEmail.setPrefColumnCount(20); fpRow6.getChildren().addAll(lblEmail, tfEmail); root.getChildren().add(fpRow6); // FlowPane FlowPane fpRow7 = new FlowPane(8,8); tfItemNum.setPrefColumnCount(5); fpRow7.getChildren().addAll(lblItemNum, tfItemNum); root.getChildren().add(fpRow7); // ROW7 - FlowPane FlowPane fpRow8 = new FlowPane(8,8); tfQuantity.setPrefColumnCount(5); fpRow8.getChildren().addAll(lblQuantity, tfQuantity); root.getChildren().add(fpRow8); scene = new Scene(root, 500, 280); stage.setScene(scene); stage.setX(100); stage.setY(100); stage.show(); } /** * actionPerformed to process the action listener for each button. * @param ActionEvent ae - the button that has been pressed */ public void handle(ActionEvent ae) { String label = ((Button)ae.getSource()).getText(); switch(label){ case "New Order": doOrder(); break; case "Number of Current Orders": doNum(); break; case "Disconnect": doDisconnect(); break; case "Connect": doConnect(); break; } } /** * doConnect connects the client with the server using sockets. */ private void doConnect(){ try{ socket = new Socket(tfServerIP.getText(), SERVER_PORT); scn = new Scanner(new InputStreamReader(socket.getInputStream())); pwt = new PrintWriter(new OutputStreamWriter(socket.getOutputStream())); } catch(IOException ioe){ } btnExit.setText("Disconnect"); }
/** * doDisconnect disconnects the client from the server. */ private void doDisconnect(){ pwt.println("doDisconnect"); pwt.flush(); try{ socket.close(); scn.close(); pwt.close(); } catch(IOException ioe) { } btnExit.setText("Connect"); } /** * doOrder creates a new order object. */ private void doOrder(){ pwt.println("doOrder"); pwt.flush(); //btnNew.setEnabled(false); try{ out = new ObjectOutputStream(socket.getOutputStream()); } catch(IOException ioe){ //OptionPane.showMessageDialog(null, "Cannot Create ObjectOutputStream: " + ioe + " ", "File Error", JOptionPane.ERROR_MESSAGE); return; } // Create order object based on text fields //Order order = null; try{ Order order = new Order(tfName.getText(), tfStreet.getText() + tfCity.getText() + tfZipcode.getText(), tfEmail.getText(), Integer.parseInt(tfItemNum.getText()), Integer.parseInt(tfQuantity.getText()), 01L); } catch(Exception e){ //OptionPane.showMessageDialog(null, "Text fields are not completed correctly: " + e + " ", "Text Error", JOptionPane.ERROR_MESSAGE); return; } try{ // Write order to the server. out.writeObject(order); out.flush(); } catch(IOException ioe){ //OptionPane.showMessageDialog(null, "Cannot Send File: " + ioe + " ", "File Error", JOptionPane.ERROR_MESSAGE); return; } // Wait for server to say it is all good. String reply = scn.nextLine(); if(reply.equals("OK")){ return; } } /** * doNum lists the number of orders currently in the list. */ private void doNum(){ pwt.println("doNum"); pwt.flush(); String reply = scn.nextLine(); }
}
Order class:
import java.io.Serializable;
public class Order implements Serializable { //attributes private static final long serialVersionUID = 01L; private String name; private String address; private String email; private int itemNo; private int quantity; //constructor public Order(String _name, String _address, String _email, int _itemNo, int _quantity){ this.name = _name; this.address = _address; this.email = _email; this.itemNo = _itemNo; this.quantity = _quantity; } //getters public String getName() { return name;} public String getAddress() { return address;} public String getEmail() { return email;} public int getItem() { return itemNo;} public int getQuantity() { return quantity;} //toString method public String toString() { return "Customer's name: " + getName() + " " + "Customer's address: " + getAddress() + " " + "EMail: " + getEmail() + " " + "Item number: " + getItem() + " " + "Quantity: " + getQuantity(); } //toCSV method public String toCSV() { return "\"" + getName() + "\",\"" + getAddress() + "\",\"" + getEmail() + "\"," + getItem() + "," + getQuantity() + " "; } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
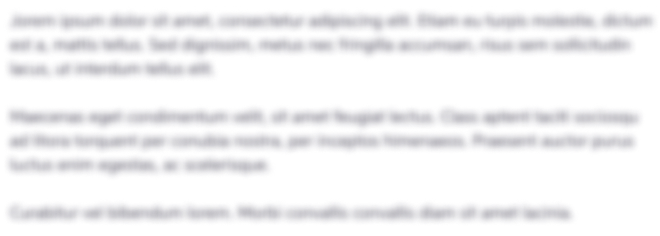
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started