Question
How to make a snake game using DrJava(with the template provided below)? /* BasicGameTemplate * Desc: Template for a basic arcade game * @author *
How to make a snake game using DrJava(with the template provided below)?
/* BasicGameTemplate * Desc: Template for a basic arcade game * @author * @version Jan 2022 */ import javax.swing.*; import java.awt.*; import java.awt.event.*;
public class BasicGameTemplate{ // Game Window properties static JFrame gameWindow; static GraphicsPanel canvas; static final int WIDTH = 1280; static final int HEIGHT = 1024; // key listener static MyKeyListener keyListener = new MyKeyListener(); // mouse listeners static MyMouseListener mouseListener = new MyMouseListener(); static MyMouseMotionListener mouseMotionListener = new MyMouseMotionListener(); //-------------------------------------------------------------------------- // declare the properties of all game objects here //-------------------------------------------------------------------------- //------------------------------------------------------------------------------ public static void main(String[] args){ gameWindow = new JFrame("Game Window"); gameWindow.setSize(WIDTH,HEIGHT); gameWindow.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); canvas = new GraphicsPanel(); canvas.addMouseListener(mouseListener); canvas.addMouseMotionListener(mouseMotionListener); canvas.addKeyListener(keyListener); gameWindow.add(canvas); gameWindow.setVisible(true); runGameLoop(); } // main method end //------------------------------------------------------------------------------ public static void runGameLoop(){ while (true) { gameWindow.repaint(); try {Thread.sleep(20);} catch(Exception e){} //------------------------------------------------------------------ // implement the game functionality here //------------------------------------------------------------------ } } // runGameLoop method end //------------------------------------------------------------------------------ static class GraphicsPanel extends JPanel{ public GraphicsPanel(){ setFocusable(true); requestFocusInWindow(); } public void paintComponent(Graphics g){ super.paintComponent(g); //required //------------------------------------------------------------------ // do all your drawings here //------------------------------------------------------------------ } // paintComponent method end } // GraphicsPanel class end //------------------------------------------------------------------------------ static class MyKeyListener implements KeyListener{ public void keyPressed(KeyEvent e){ int key = e.getKeyCode(); } public void keyReleased(KeyEvent e){ int key = e.getKeyCode(); } public void keyTyped(KeyEvent e){ char keyChar = e.getKeyChar(); } } // MyKeyListener class end //------------------------------------------------------------------------------ static class MyMouseListener implements MouseListener{ public void mouseClicked(MouseEvent e){ int mouseX = e.getX(); int mouseY = e.getY(); } public void mousePressed(MouseEvent e){ } public void mouseReleased(MouseEvent e){ } public void mouseEntered(MouseEvent e){ } public void mouseExited(MouseEvent e){ } } // MyMouseListener class end //------------------------------------------------------------------------------ static class MyMouseMotionListener implements MouseMotionListener{ public void mouseMoved(MouseEvent e){ } public void mouseDragged(MouseEvent e){ } } // MyMouseMotionListener class end } // BasicGameTemplate class end
Template code:
Step by Step Solution
There are 3 Steps involved in it
Step: 1
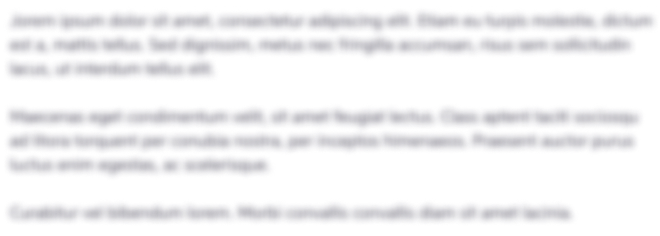
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started