Question
How to use Binary Heap to create Huffman tree in Huffman Encode This is my binary heap class: public class BinaryHeap { // implements a
How to use Binary Heap to create Huffman tree in Huffman Encode
This is my binary heap class:
public class BinaryHeap {
// implements a binary heap where the heap rule is the value in the parent
// node is less than
// or equal to the values in the child nodes
// the implementation uses parallel arrays to store the priorities and the
// trees
int priority[];
HuffmanTree trees[];
int size;
public BinaryHeap(int s) {
priority = new int[s + 1];
trees = new HuffmanTree[s + 1];
size = 0;
}
public void removeMin() {
// PRE: size != 0
// removes the priority and tree at the root of the heap
// PRE: size != 0;
// removes the priority and the tree at the root of the heap
int parent;
int child;
int x = priority[size];
HuffmanTree z = trees[size];
size--;
child = 2;
while(child <= size) {
parent = child / 2;
if(child < size && priority[child+1] < priority[child])
child++;
if(x < priority[child]) break;
priority[parent] = priority[child];
trees[parent] = trees[child];
child = 2 * child;
}
priority[child/2] = x;
trees[child/2] = z;
}
public int getMinPriority() {
// PRE: size != 0
// return the priority in the root of the heap
return priority[1];
}
public HuffmanTree getMinTree() {
// PRE: size != 0
// return the tree in the root of the heap
return trees[1];
}
public boolean full() {
// return true if the heap is full otherwise return false
return size == priority.length - 1;
}
public void insert(int p, HuffmanTree t) {
// insert the priority p and the associated tree t into the heap
// PRE !full()
int parent;
int child;
size++;
child = size;
parent = child/2;
priority[0] = p;
trees[0] = t;
while (priority[parent] > p) {
priority[child] = priority[parent];
trees[child] = trees[parent];
child = parent;
parent = child/2;
}
priority[child] = p;
trees[child] = t;
}
public int getSize() {
// return the number of values (priority , tree) pairs in the heap
return size;
}
}
I need help in HuffmanEncode class. I finished the code but when i run the code. It will shows "Exception in thread "main" java.lang.ArrayIndexOutOfBoundsException: 129".
public class HuffmanEncode {
int totalCharacters;
public HuffmanEncode(String in, String out) {
// Implements the Huffman encoding algorithm
// Add private methods and instance variables as needed
BinaryHeap heap = new BinaryHeap(128);
int[] characterArray = new int[128];
characterArray = readFile(in, characterArray);
// putting everything from array into priority queue
for (int i = 0; i < characterArray.length; ++i) {
if (characterArray[i] > 0) {
HuffmanTree temp = new HuffmanTree((char) i);
Item item = new Item(temp, characterArray[i]);
heap.insert(item.frequency, temp);
}
}
// popping two things from priority queue, merging them, then pushing that
// tree back onto the priority queue
while (heap.getSize() > 1) {
char d = (char) 128;
int left = heap.getMinPriority();
int right = heap.getMinPriority();
HuffmanTree n = heap.getMinTree();
Item item1 = new Item(n, left);
Item item2 = new Item(n, right);
HuffmanTree newTree = new HuffmanTree(item1.tree, item2.tree, d);
int newNum = item1.frequency + item2.frequency;
Item item = new Item(newTree, newNum);
heap.insert(item.frequency, newTree);
}
HuffmanTree tree = heap.getMinTree();
String[] encodingsArray = new String[128];
Iterator
while (iter.hasNext()) {
String s = iter.next();
char c = s.charAt(0);
encodingsArray[(int) c] = s.substring(1, s.length());
}
HuffmanOutputStream outFile = new HuffmanOutputStream(out, tree.toString(), totalCharacters);
writeFile(in, outFile, encodingsArray);
}
private class Item implements Comparable {
HuffmanTree tree;
int frequency;
public Item(HuffmanTree t, int n) {
tree = t;
frequency = n;
}
public int compareTo(Object x) {
return frequency - (((Item) x).frequency);
}
}
/**
*
* method to read in the characters from a file and find their frequencies
*
* @param file
* @param array
* @return
*/
private int[] readFile(String file, int[] array) {
try {
FileReader fin = new FileReader(file);
BufferedReader reader = new BufferedReader(fin);
int character = reader.read();
// while not at end of file add one to that character in the array
while (character != -1) {
array[character]++;
totalCharacters++;
character = reader.read();
}
} catch (IOException e) {
System.out.println("IOException");
}
return array;
}
/**
*
* write the bits to file
*
* @param in
* @param out
* @param array
*/
private void writeFile(String in, HuffmanOutputStream out, String[] array) {
try {
FileReader fin = new FileReader(in);
BufferedReader reader = new BufferedReader(fin);
int character = reader.read();
while (character != -1) {
for (int i = 0; i < array[character].length(); i++) {
String s = array[character];
out.writeBit((char) (s.charAt(i) - '0'));
}
character = reader.read();
}
fin.close();
reader.close();
out.close();
} catch (IOException e) {
System.out.print("IOException");
}
}
public static void main(String args[]) {
// args[0] is the name of the file whose contents should be compressed
// args[1] is the name of the output file that will hold the compressed
// content of the input file
new HuffmanEncode(args[0], args[1]);
// do not add anything here
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
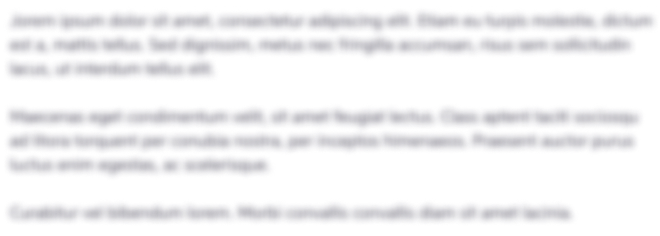
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started