Question
How to write an implementation of a deque using doubly linked list? I have the API and the requirements attached I am also attaching the
How to write an implementation of a deque using doubly linked list?
I have the API and the requirements attached
I am also attaching the code I've written for reference, but it is getting NullPointerException for the removeLast Method.
import java.util.Iterator; import java.util.NoSuchElementException;
import stdlib.StdOut; import stdlib.StdRandom;
// A data type to represent a double-ended queue (aka deque), implemented using a doubly-linked // list as the underlying data structure. public class LinkedDeque
// Returns true if this deque is empty, and false otherwise. public boolean isEmpty() { return first == null; }
// Returns the number of items in this deque. public int size() { return n; }
// Adds item to the front of this deque. public void addFirst(Item item) { if (item == null) { throw new NullPointerException("item is null"); } Node oldfirst = first; first = new Node(); first.item = item; first.next = oldfirst; first.prev = null; if (last == null) { last = first; } n++; }
// Adds item to the back of this deque. public void addLast(Item item) { if (item == null) { throw new NullPointerException("item is null"); } Node oldlast = last; last = new Node(); last.item = item; last.prev = oldlast; last.next = null; if (first == null) { first = last; } n++; }
// Returns the item at the front of this deque. public Item peekFirst() { if(isEmpty()) { throw new NoSuchElementException("Deque is empty"); } return first.item; }
// Removes and returns the item at the front of this deque. public Item removeFirst() { Item item = first.item; if (isEmpty()) { throw new NoSuchElementException("Deque is empty"); } if (first.next == null) { first = null; last = null; } else { first = first.next; first.prev = null; } n--; return item; }
// Returns the item at the back of this deque. public Item peekLast() { if(isEmpty()) { throw new NoSuchElementException("Deque is empty"); } return last.item; }
// Removes and returns the item at the back of this deque. public Item removeLast() { Item item = last.item; if (isEmpty()) { throw new NoSuchElementException("Deque is empty"); } if (first.next == null) { first = null; last = null; } else { last = last.prev; last.next = null; } n--; return item; }
// Returns an iterator to iterate over the items in this deque from front to back. public Iterator
// Returns a string representation of this deque. public String toString() { StringBuilder sb = new StringBuilder(); for (Item item : this) { sb.append(item); sb.append(", "); } return n > 0 ? "[" + sb.substring(0, sb.length() - 2) + "]" : "[]"; }
// A deque iterator. private class DequeIterator implements Iterator
// Constructs an iterator. public DequeIterator() { current = first; }
// Returns true if there are more items to iterate, and false otherwise. public boolean hasNext() { return current != null; }
// Returns the next item. public Item next() { if (!hasNext()) { throw new NoSuchElementException("Iterator is empty"); } Item item = current.item; current = current.next; return item; }
// Unsupported method. public void remove() { throw new UnsupportedOperationException("remove() is not supported"); } }
// A data type to represent a doubly-linked list. Each node in the list stores a generic item // and references to the next and previous nodes in the list. private class Node { private Item item; // the item private Node next; // the next node private Node prev; // the previous node }
// Unit tests the data type. [DO NOT EDIT] public static void main(String[] args) { LinkedDeque
Step by Step Solution
There are 3 Steps involved in it
Step: 1
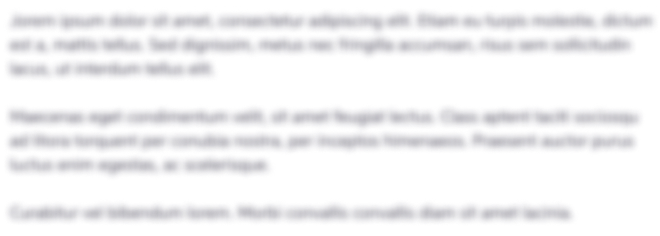
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started