Answered step by step
Verified Expert Solution
Question
1 Approved Answer
how to write this unit tests for all classes? @Slf4j @Service public class ServiceCallsImpl implements ServiceCalls { private final CacheManager cacheManager; private final JwtTokenGetterClient jwtTokenGetterClient;
how to write this unit tests for all classes?
@Slf4j @Service public class ServiceCallsImpl implements ServiceCalls { private final CacheManager cacheManager; private final JwtTokenGetterClient jwtTokenGetterClient; private final UserAuthService userAuthService; private final JwtTokenService jwtTokenService; private final PermissionClient permissionClient; private final PerformanceUtils perf = new PerformanceUtils(false); @Value("${okta.clientId}") private String clientId; @Value("${okta.oktaServer}") private String oktaServer; @Autowired public ServiceCallsImpl(CacheManager cacheManager, JwtTokenGetterClient jwtTokenGetterClient, UserAuthService userAuthService, JwtTokenService jwtTokenService, PermissionClient permissionClient) { // public ServiceCallsImpl(FF4j ff4j, CacheManager cacheManager, JwtTokenGetterClient jwtTokenGetterClient, UserAuthService userAuthService, JwtTokenService jwtTokenService, PermissionClient permissionClient) { // this.ff4j = ff4j; this.cacheManager = cacheManager; this.jwtTokenGetterClient = jwtTokenGetterClient; this.userAuthService = userAuthService; this.jwtTokenService = jwtTokenService; this.permissionClient = permissionClient; // if (logger.isDebugEnabled()) { // perf.enabled(true); // } } /** * Gets validate * * @return new ValidateEntity */ public ValidateEntity getValidate(String ruleName, String operation) { ValidateProto.Validate validate = permissionClient.getValidate(getBearerToken(), ruleName, operation); return validate != null ? createValidateEntityFromProto(validate) : null; } /** * {@inheritDoc} */ public String getBearerToken() { // First try to get the token passed in from the user if there was one perf.init().start(); String userToken = userAuthService.getAuthorizationToken(); perf.end().elapsed(this.getClass().getName(), "got bearer token from user service"); if (null == userToken || userToken.isBlank()) { // Try getting it from cache userToken = checkCachedOktaToken(DateTime.now().plusMinutes(10)); } return null != userToken ? "Bearer " + userToken : null; } /** * {@inheritDoc} */ public String checkCachedOktaToken(DateTime dateTime) { // Try getting it from cache String userToken = PdrmCacheUtil.getOktaTokenFromCache(cacheManager, SprinklrConstants.OKTA_TOKEN_CACHE); perf.init().start(); if (null != userToken && !userToken.isBlank()) { DateTime checkDate = dateTime; if (null == checkDate) { // Default to now plus 10 minutes if no date is passed checkDate = DateTime.now().plusMinutes(10); } // Check to see if it's expired. Checks for 10 minutes early so the token doesn't expire if (isJwtExpired(userToken, checkDate)) { // If expired then get a new one and add it to cache userToken = getNewOktaToken(); } else { // logger.debug("Got token from cache and it is not expired"); } } else { // Didn't get a token from cache so get a new one and add it to cache userToken = getNewOktaToken(); } perf.end().elapsed(this.getClass().getName(), "checked okta token expiration"); return userToken; } /** * {@inheritDoc} */ public void getNewOktaJWKSlist() { ResponseEntity response; try { perf.init().start(); response = jwtTokenGetterClient.getJwks(oktaServer, clientId); if (null == response || !response.getStatusCode().equals(HttpStatus.OK)) { //TODO email notificaiton to admin that this failed to look into // logger.debug("Could not get public keys - response code: {} - response message: {}", null != response ? response.getStatusCode() : null, null != response ? response.getBody() : null); } else { JSONObject responseObject = new JSONObject(response.getBody()); JSONArray keys = responseObject.getJSONArray("keys"); if (null != keys && keys.length() > 0) { List oktaKeyEntities = new ArrayList<>(); for (int i = 0; i < keys.length(); i++) { oktaKeyEntities.add(new PublicJWKeyEntity(keys.getJSONObject(i).get("kid").toString(), keys.getJSONObject(i).get("n").toString(), keys.getJSONObject(i).get("e").toString())); } if (!oktaKeyEntities.isEmpty()) { PdrmCacheUtil.populateCache(cacheManager, SprinklrConstants.OKTA_PUBLIC_KEYS_CACHE, oktaKeyEntities); } } else { // logger.debug("Could not get public keys"); //TODO email notificaiton to admin that this failed to look into } } perf.end().elapsed(this.getClass().getName(), "got new Okta public keys from service"); } catch (Exception ex) { //TODO email notificaiton to admin that this failed to look into // logger.debug("Exception getting public keys: " + ex); } } /** * Gets a new token from Okta * * @return new token */ private String getNewOktaToken() { String token = null; perf.init().start(); TokenEntity tokenEntity = jwtTokenService.getToken(); if (null != tokenEntity && null != tokenEntity.getToken() && !tokenEntity.getToken().isBlank()) { token = tokenEntity.getToken(); // logger.debug("Got a new token from Okta"); // Add the new token to cache PdrmCacheUtil.populateCache(cacheManager, SprinklrConstants.OKTA_TOKEN_CACHE, token); } perf.end().elapsed(this.getClass().getName(), "got new Okta token from service"); return token; } /** * Checks to see if the specified token is expired. It compares to the date passed in. * * @param token token to check * @param dateTime date to check the token against * @return true if expired, otherwise false */ public static Boolean isJwtExpired(String token, DateTime dateTime) { try { SignedJWT signedJWT = SignedJWT.parse(token); JWTClaimsSet claimsSet = signedJWT.getJWTClaimsSet(); Date expirationDate = claimsSet.getExpirationTime(); // logger.debug("token expirationDate is: {}", expirationDate); // logger.debug("time-to-check that was passed in is: {}", dateTime.toDate()); if (null != expirationDate && (expirationDate.before(dateTime.toDate()) || expirationDate.equals(dateTime.toDate()))) { return true; } } catch (Exception e) { // logger.debug("Error checking expiration date for token " + token); // logger.debug(Throwables.getStackTraceAsString(e)); } return false; } }
public class FeignConfiguration { @Bean Logger.Level feignLoggerLevel() { return Logger.Level.FULL; } @Bean public FeignErrorDecoder pdrmErrorDecoder() { return new FeignErrorDecoder(); } @Autowired private ObjectFactorymessageConverters; @Bean @Primary @Scope(SCOPE_PROTOTYPE) Encoder feignFormEncoder() { return new FormEncoder(new SpringEncoder(this.messageConverters)); } }
@Slf4j public class FeignErrorDecoder implements ErrorDecoder { private final ErrorDecoder defaultErrorDecoder = new Default(); private static final Logger LOGGER = LoggerFactory.getLogger(WebClientUtils.class); @Override public Exception decode(String methodKey, Response response) { if (response.status() >= 300 && response.status() <= 399) { LOGGER.debug("Feign got a redirection response of {}", response.status()); LOGGER.debug("Feign response: {}", response); } if (response.status() >= 400 && response.status() <= 499) { LOGGER.debug("Feign got a client error response of {}", response.status()); LOGGER.debug("Feign got a client error methodKey {}", methodKey); LOGGER.debug("Feign response: {}", response); LOGGER.debug("Request: " + response.request()); LOGGER.debug("Request URL: " + response.request().url()); } if (response.status() >= 500 && response.status() <= 599) { LOGGER.debug("Feign got a system error response of {}", response.status()); LOGGER.debug("Feign response: {}", response); } return defaultErrorDecoder.decode(methodKey, response); } }
@Slf4j @Component public class ValidateImpl implements Validate { private final PerformanceUtils perf = new PerformanceUtils(false); private final ServiceCalls serviceCalls; @Autowired public ValidateImpl(ServiceCalls serviceCalls) { this.serviceCalls = serviceCalls; // if (logger.isDebugEnabled()) { // perf.enabled(true); // } } /** * {@inheritDoc} */ public ValidateEntity get(String ruleName, String operation) { return serviceCalls.getValidate(ruleName, operation); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
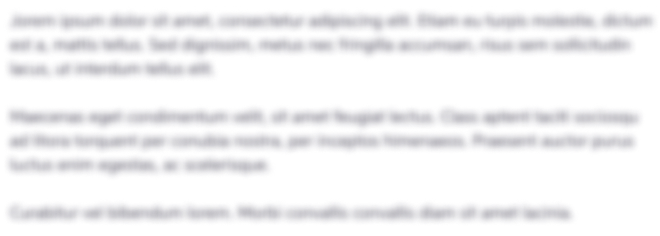
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started