Question
How would I code a Presentation class with what I already have? The presentation class should ask for the employee number, last name, first name,
How would I code a Presentation class with what I already have? The presentation class should ask for the employee number, last name, first name, email address and social security number. The presentation classs main method should consist almost entirely of method calls. Error checking should be done on all user-entered fields using a Validation class. Once the fields have been validated, those values should be passed to business class using the set methods. The application class should also ask if the employee is hourly or salary. Based on that answer; determine which calcPay method to call in order to calculate pay. If the employee is hourly, the application should prompt the user for the number of hours worked. That value should be passed to the hourly subclass by calling the set method. If the employee is salary, the application should prompt the user for a yearly salary. That value should be passed to the salary subclass by calling the set method. The application class should display all of the users information by calling an overridden toString() method along with their formatted pay by calling the appropriate methods in the business classes. The user should be prompted as to whether they would like to calculate pay for another employee. If the answer is Y in either upper or lower case, the application should start again at the beginning.
Employee.java
public abstract class Employee {
//Declaring instance variables
private int empNo;
private String firstname;
private String lastname;
private String email;
private String socialSecurityNumber;
public Employee() {
}
//Parameterised constructor
public Employee(int empNo,String firstname, String lastname,String email,String socialSecurityNumber) {
this.empNo=empNo;
this.firstname = firstname;
this.lastname = lastname;
this.email=email;
this.socialSecurityNumber = socialSecurityNumber;
}
//Setters and Getters.
public String getFirstname() {
return firstname;
}
public void setFirstname(String firstname) {
this.firstname = firstname;
}
public String getLastname() {
return lastname;
}
public void setLastname(String lastname) {
this.lastname = lastname;
}
public String getSocialSecurityNumber() {
return socialSecurityNumber;
}
public void setSocialSecurityNumber(String socialSecurityNumber) {
this.socialSecurityNumber = socialSecurityNumber;
}
public String getEmail() {
return email;
}
public void setEmail(String email) {
this.email = email;
}
public abstract double calcPay(double n);
@Override
public String toString() {
System.out.println("Firstname :"+getFirstname());
System.out.println("Lastname :"+getLastname());
System.out.println("Email :"+email);
System.out.println("Social Security No :"+getSocialSecurityNumber());
return " ";
}
}
___________________
HourlyEmployee.java
public class HourlyEmployee extends Employee {
//Declaring instance variables
private final double HOURLYWAGE=15.75;
private int hoursworked;
//Parameterised constructor
public HourlyEmployee(int empNo,String firstname, String lastname,String email, String ssn,
double hourlyWage, int hoursworked) {
super(empNo,firstname,lastname,email,ssn);
this.hoursworked=hoursworked;
}
//Setters and Getters.
public int getHoursworked() {
return hoursworked;
}
public void setHoursworked(int hoursworked) {
if(hoursworked>0 && hoursworked<=168)
{
this.hoursworked = hoursworked;
}
}
//toString() method will display the contents of the Object.
@Override
public String toString() {
System.out.print("Hourly Employee:");
super.toString();
System.out.println("Hours Worked :"+getHoursworked());
System.out.println("Earnings :"+calcPay(hoursworked));
return " ";
}
@Override
public double calcPay(double n) {
return n*HOURLYWAGE;
}
}
__________________
SalariedEmployee.java
public class SalariedEmployee extends Employee {
// Declaring instance variables
private double ysalary;
// Parameterised constructor
public SalariedEmployee(int empNo,String firstname, String lastname, String email,
String ssn, double ysalary) {
super(empNo,firstname, lastname, email, ssn);
this.ysalary = ysalary;
}
// Setters and Getters.
public double getSalary() {
return ysalary;
}
public void setSalary(double salary) {
if (salary > 0) {
this.ysalary = salary;
}
}
// toString() method will display the contents of the Object.
@Override
public String toString() {
System.out.print("Salaried Employee:");
super.toString();
System.out.println("Salary: : " + getSalary());
System.out.println("Earnings: " + calcPay(ysalary));
return " ";
}
@Override
public double calcPay(double n) {
return n / 12;
}
}
__________________
Step by Step Solution
There are 3 Steps involved in it
Step: 1
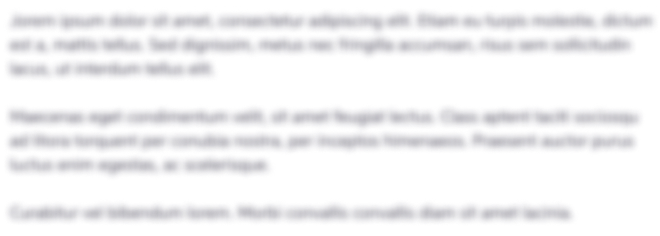
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started