Question
How would I finish this algorithm off, to where the player or computer hits 100, in the dice game and it says game over. The
How would I finish this algorithm off, to where the player or computer hits 100, in the dice game and it says
"game over". The classes built out of the GUI are working fine in my gameTester class, only when my GUI is used do I not get winner "gameover" when the player or computer breaks one hundred. Anyway, I know the fix is in the highlighted code. I just am not sure how I should write it. How would I finish off this algorithm to do that?
Note: the fix should be in my highlighted code section, no other code outside the GUIDriver can be changed.
Thank-you
//-------------------------------------------------------------------
// If game is not over, calls the beAPig method for current Player
// Calls playGame method and updates the GUI components accordingly
//-------------------------------------------------------------------
public void processButtonPress(ActionEvent event)
{
if(!g.gameOver())
{
if(piggy == event.getSource())
{
g.getCurrentPlayer().beAPig(true);
}
else
{
g.getCurrentPlayer().beAPig(false);
}
if(g.playGame() ==true)
{
}
Full pertenent code below:
// Demonstrates JavaFX pushButton and Die Images
//*************************************************************************
public class GUIDriver extends Application
{
private Text sumText, humanScore, computerScore, currentPlayerIs;
private ImageView die1View, die2View;
private Game g;
private Button piggy, pass, proceed;
//-----------------------------------------------------------------------
// Constructor: creates ImageView, Flowpane etc.
// Initializing sumText, images pig1, pig2
//-----------------------------------------------------------------------
public void start(Stage primaryStage)
{
//--------------------------------------------------------------------
// declaring variables
//--------------------------------------------------------------------
g = new Game();
sumText = new Text ("Sum is: 0");
humanScore = new Text(g.getHuman().toString());
computerScore = new Text(g.getComputer().toString());
Image pig1 = g.getPigDice().getDie1Image();
Image pig2 = g.getPigDice().getDie2Image();
die1View = new ImageView(pig1);
die2View = new ImageView(pig2);
//--------------------------------------------------------------------
// creates the piggy button, if piggy is pressed it does an action
//--------------------------------------------------------------------
piggy = new Button ("Roll like a pig!");
piggy.setOnAction(this::processButtonPress);
//---------------------------------------------------------------------
// creates the pass button, if pass is pressed it does an action
//---------------------------------------------------------------------
pass = new Button ("Pass like a pansy!");
pass.setOnAction(this::processButtonPress);
//----------------------------------------------------------------------
// creates the proceed button, if proceed is pressed it does an action
//----------------------------------------------------------------------
proceed = new Button ("Computer");
proceed.setOnAction(this::processButtonPress);
//----------------------------------------------------------------------
// creates Flowpane, position, and color
//---------------------------------------------------------------------------
FlowPane pane = new FlowPane(die1View, die2View, piggy, pass, proceed, sumText, humanScore, computerScore);
pane.setPrefWrapLength(100);
pane.setStyle("-fx-background-color: lightgreen");
pane.setAlignment(javafx.geometry.Pos.CENTER);
pane.setHgap(10);
pane.setVgap(20);
//---------------------------------------------------------------
// Setting scene and title
//---------------------------------------------------------------
Scene scene = new Scene(pane, 800, 600);;
primaryStage.setTitle("Pigger Game");
primaryStage.setScene(scene);
primaryStage.show();
}
//-------------------------------------------------------------------
// If game is not over, calls the beAPig method for current Player
// Calls playGame method and updates the GUI components accordingly
//-------------------------------------------------------------------
public void processButtonPress(ActionEvent event)
{
if(!g.gameOver())
{
if(piggy == event.getSource())
{
g.getCurrentPlayer().beAPig(true);
}
else
{
g.getCurrentPlayer().beAPig(false);
}
if(g.playGame() ==true)
{
}
die1View.setImage(g.getPigDice().getDie1Image());
die2View.setImage(g.getPigDice().getDie2Image());
sumText.setText("Sum is: " + g.getPigDice().toString());
humanScore.setText(g.getHuman().toString());
computerScore.setText(g.getComputer().toString());
currentPlayerIs.setText(g.getCurrentPlayer().getName());
}
}
}
---------------------------------------------------------------------------------------------------------------------
Game class
---------------------------------------------------------------------------------------------------------------------
//******************************************************************************************
public class Game
{
//---------------------------------------------------------------------------------------
// Class level variables
//---------------------------------------------------------------------------------------
private Player p1, p2, current;
private PigDice d;
private boolean gameOver;
private final int WINNING_SCORE;
//---------------------------------------------------------------------------------------
//creates the two players and a pigDice, initializes the current player to start the game
//and sets game over condition to false.
//---------------------------------------------------------------------------------------
public Game()
{
p1 = new Human("Player");
p2 = new Computer("Computer");
d = new PigDice();
WINNING_SCORE = 100;
gameOver = false;
current = p1;
}
//---------------------------------------------------------------------------------------
// algorithm: if the game is not over, has the current player take its turn.
// If the current players pigness value is false after the turn, switches the
// current player. Returns true if the currentPlayer changes, false otherwise.
//---------------------------------------------------------------------------------------
public boolean playGame()
{
boolean result = false;
if(!gameOver)
{
current.takeTurn(d);
if(!current.getPigness())
{
if(current == p1)
{
current = p2;
result = true;
}
else
{
current = p1;
result = true;
}
}
}
return result;
}
//---------------------------------------------------------------------------------------
// if either player's current round points + accumulated game points is
// greater or equal to the winning score,
// sets the value of game over to true (false otherwise) and returns the result.
//---------------------------------------------------------------------------------------
public boolean gameOver()
{
if(current.getGamePoints() + current.getRoundPoints() >= WINNING_SCORE)
{
current.gamePoints = (current.getGamePoints() + current.getRoundPoints());
gameOver = true;
}
return gameOver;
}
//---------------------------------------------------------------------------------------
// return current
//---------------------------------------------------------------------------------------
public Player getCurrentPlayer()
{
return current;
}
//---------------------------------------------------------------------------------------
// getter Human player
//---------------------------------------------------------------------------------------
public Player getHuman()
{
return p1;
}
//---------------------------------------------------------------------------------------
// getter Computer player
//---------------------------------------------------------------------------------------
public Player getComputer()
{
return p2;
}
//--------------------------------------------------------------------------------------
// getter PigDice
//--------------------------------------------------------------------------------------
public PigDice getPigDice()
{
return d;
}
//--------------------------------------------------------------------------------------
// ToString method
//--------------------------------------------------------------------------------------
public String toString()
{
return "";
}
}
-------------------------------------------------------------------------------------------------------------------------------------------------------
Player Class
-------------------------------------------------------------------------------------------------------------------------------------------------------
public abstract class Player
{
//---------------------------------------------------------------------------------
// declaring protected variables
//---------------------------------------------------------------------------------
protected String name;
protected int roundPoints, gamePoints;
protected boolean pigness;
//---------------------------------------------------------------------------------
// constructor for name
//---------------------------------------------------------------------------------
public Player(String name)
{
this.name = name;
roundPoints = 0;
gamePoints = 0;
pigness = true;
}
//---------------------------------------------------------------------------------
// getter name
//---------------------------------------------------------------------------------
public String getName()
{
return name;
}
//---------------------------------------------------------------------------------
// getter RoundPoints
//---------------------------------------------------------------------------------
public int getRoundPoints()
{
return roundPoints;
}
//---------------------------------------------------------------------------------
// getter GamePoints
//---------------------------------------------------------------------------------
public int getGamePoints()
{
return gamePoints;
}
//---------------------------------------------------------------------------------
// getter boolean Pigness
//---------------------------------------------------------------------------------
public boolean getPigness()
{
return pigness;
}
//---------------------------------------------------------------------------------
// if the players pigness is true rolls the PigDice and sets the Players roundPoints, gamePoints
// and pigness according to the roll results, otherwise adds the roundPoints to the gamePoints
// and zeroes out the roundPoints.
//---------------------------------------------------------------------------------
public void takeTurn(PigDice p)
{
if(pigness)
{
int points = p.roll();
switch (points)
{
case 2:
{
gamePoints = 0;
}
case 3:
{
roundPoints = 0;
pigness = false;
break;
}
default:
{
roundPoints += points;
pigness = true;
}
}
}
else
{
gamePoints = gamePoints + roundPoints;
roundPoints = 0;
pigness = false;
}
}
//---------------------------------------------------------------------------------
// toString method returns player points results
//---------------------------------------------------------------------------------
public abstract boolean beAPig(boolean isPig);
@Override
public String toString()
{
return "Player{" +
"name = " + name +
", roundPoints =" + roundPoints +
", gamePoints =" + gamePoints +
", pigness =" + pigness + "}";
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
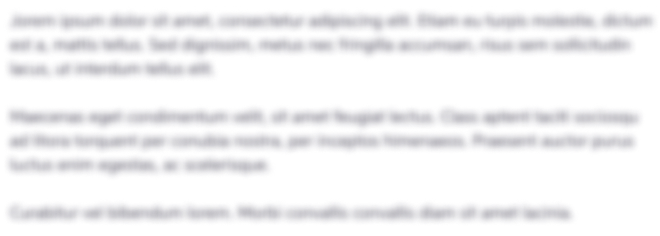
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started