Question
How would I write/implement code into the testing of my code way below? My testing code is directly below this but it's unfinished because I'm
How would I write/implement code into the testing of my code way below? My testing code is directly below this but it's unfinished because I'm a little lost on what to do. It's a caesar cipher, so what I need to do is show my output and finish my testing cases.
--------------------------------
what I have for testing below
--------------------------------
import java.io.File; import java.io.FileInputStream; import java.io.FileOutputStream; import java.io.InputStream; import java.io.IOException; import java.io.OutputStream; import java.util.Scanner; /** This program encrypts a file, using the Caesar cipher. */ public class CipherTest { public static void main(String[] args) { Scanner in = new Scanner(System.in); try { System.out.print("Input file: "); String inFile = in.next(); System.out.print("Output file: "); String outFile = in.next(); System.out.print("Encryption key: "); int key = in.nextInt(); InputStream inStream = new FileInputStream(inFile); OutputStream outStream = new FileOutputStream(outFile); Cipher cipher = new Cipher(key); cipher.encryptStream(inStream, outStream); inStream.close(); outStream.close(); } catch (IOException exception) { System.out.println("Error processing file: " + exception); } } }
---------------
My code below
---------------
import java.io.InputStream;
import java.io.OutputStream;
import java.io.IOException;
/**
* This class encrypts files using the substitution ciphers
*/
public class Cipher {
private String secret;
/**
* Constructs a cipher object with a given secret key.
*
* @param aKey
* the encryption key
*/
public Cipher(String secret) {
this.secret = secret.toUpperCase();
}
/**
* Encrypts the contents of a stream.
*
* @param in
* the input stream
* @param out
* the output stream
*/
public void encryptStream(InputStream in, OutputStream out)
throws IOException {
boolean done = false;
while (!done) {
int next = in.read();
if (next == -1)
done = true;
else {
char b = (char) next;
char c = encrypt(b);
out.write(c);
}
}
}
/**
* Decrypts the contents of a stream.
*
* @param in
* the input stream
* @param out
* the output stream
*/
public void decryptStream(InputStream in, OutputStream out)
throws IOException {
boolean done = false;
while (!done) {
int next = in.read();
if (next == -1)
done = true;
else {
char b = (char) next;
char c = encrypt(b);
out.write(c);
}
}
}
/**
* Encrypts a char.
*
* @param b
* the char to encrypt
* @return the encrypted char
*/
public char encrypt(char c) {
if (Character.isUpperCase(c))
return Character.toUpperCase(secret.charAt(c - 'A'));
if (Character.isLowerCase(c))
return Character.toLowerCase(secret.charAt(c - 'a'));
return c;
}
/**
* Decrypts a char.
*
* @param b
* the char to encrypt
* @return the Decrypted char
*/
public char decrypt(char c) {
if (Character.isUpperCase(c))
return (char) ('A' + secret.indexOf(Character.toUpperCase(c)));
if (Character.isLowerCase(c))
return (char) ('a' + secret.indexOf(Character.toUpperCase(c)));
return c;
}
public static void main(String args[]) throws IOException {
Cipher cipher = new Cipher("FEATHRZYXWVUSQPONMLKJIGDCB");
cipher.encryptStream(System.in, System.out);
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
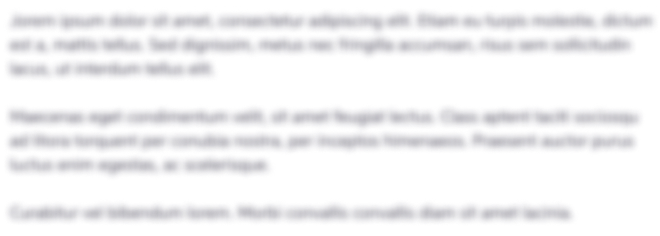
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started