Question
I am coding in c++ a program to calculate for the landing position of a projectile with a given velocity and angle. We are to
I am coding in c++ a program to calculate for the landing position of a projectile with a given velocity and angle. We are to show the user the landing position only, and save to a document the y position of the object over 30 increments, where one incrememt is the (time of travel / 30)
Here is what my code looks like:
// Projectile Motion
#include
#include
#include
#define pi 3.14159
#define g -9.81
using namespace std;
int main()
{
double landingPoint;
double angle;
double initialVelocity;
double xo = 0.0;
double yo = 0.0;
double vox;
double voy;
double time;
double interval;
double timeStamp;
cout << "Enter the angle at which the projectile is being fired at (in degrees with respect to the positive x-axis): ";
cin >> angle;
while (angle <= 0 || angle >= 90)
{
cout << "Angle must be between 0 and 90."
<< " Re-enter the angle. ";
cin >> angle;
}
cout << "Enter the initial velocity at which the projectile is being fired at (in meters per second): ";
cin >> initialVelocity;
while (initialVelocity <= 0)
{
cout << "Initial velocity must be greater than 0."
<< " Re-enter the initial velocity. ";
cin >> initialVelocity;
}
vox = initialVelocity*cos(angle*pi / 180);
voy = initialVelocity*sin(angle*pi / 180);
time = 2 * voy / g;
interval = time / 30;
timeStamp = 1;
landingPoint = vox * time;
cout << "The projectile will land at x = " << landingPoint << " meters ";
ofstream outFile;
{
outFile.open("loosresults.txt");
outFile << "The y position of the object at time intervals of t/30. ";
for (double interval = 1; interval <= 30; interval++)
for (double timeStamp = 1; timeStamp <= 30; timeStamp++)
outFile << "Time stamp: " << timeStamp << "\t Height: " << (voy*interval) - (.5*g*(interval*interval)) << " ";
}
system("pause");
Here is what my data looks like:
The y position of the object at time intervals of t/30.
Time stamp: 1 Height: -2.41006
Time stamp: 2 Height: -2.41006
Time stamp: 3 Height: -2.41006
Time stamp: 4 Height: -2.41006
Time stamp: 5 Height: -2.41006
Time stamp: 6 Height: -2.41006
Time stamp: 7 Height: -2.41006
Time stamp: 8 Height: -2.41006
Time stamp: 9 Height: -2.41006
Time stamp: 10 Height: -2.41006
Time stamp: 11 Height: -2.41006
Time stamp: 12 Height: -2.41006
Time stamp: 13 Height: -2.41006
Time stamp: 14 Height: -2.41006
Time stamp: 15 Height: -2.41006
Time stamp: 16 Height: -2.41006
Time stamp: 17 Height: -2.41006
Time stamp: 18 Height: -2.41006
Time stamp: 19 Height: -2.41006
Time stamp: 20 Height: -2.41006
Time stamp: 21 Height: -2.41006
Time stamp: 22 Height: -2.41006
Time stamp: 23 Height: -2.41006
Time stamp: 24 Height: -2.41006
Time stamp: 25 Height: -2.41006
Time stamp: 26 Height: -2.41006
Time stamp: 27 Height: -2.41006
Time stamp: 28 Height: -2.41006
Time stamp: 29 Height: -2.41006
Time stamp: 30 Height: -2.41006
Time stamp: 1 Height: -14.6301
Time stamp: 2 Height: -14.6301
Time stamp: 3 Height: -14.6301
Time stamp: 4 Height: -14.6301
Time stamp: 5 Height: -14.6301
Time stamp: 6 Height: -14.6301
Time stamp: 7 Height: -14.6301
Step by Step Solution
There are 3 Steps involved in it
Step: 1
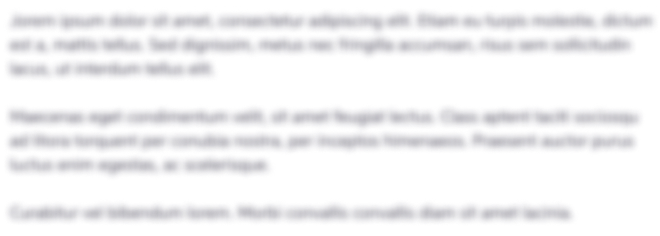
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started