Answered step by step
Verified Expert Solution
Question
1 Approved Answer
I am currently doing a Run-Length Encoding project for my intro to Java class. The program is supposed to ask the user for a String
I am currently doing a Run-Length Encoding project for my intro to Java class. The program is supposed to ask the user for a String to be both encoded and decoded. I believe most of the code in my methods is correct, but I am having trouble 1) figuring out how all of these methods are supposed to work together to gain the end result and 2) figuring out how to get the return values to work where I need them (specifically in my switch statements, I keep getting error messages when I try to compile it).
import java.util.Scanner; public class RLE { public static void main(String[] args) { String inputString = ""; //INITIALIZES INPUTSTRING Menu(); } public static void Menu() { System.out.println("What would you like to do?"); //MENU OPTIONS System.out.println("1. Input string to be encoded"); System.out.println("2. View encoded string"); System.out.println("3. View decoded string"); System.out.println("4. Exit program"); System.out.print("Option: "); Scanner scnr = new Scanner(System.in); int MenuInput = scnr.nextInt(); System.out.println(""); String inputString; switch (MenuInput) { case 1: Scanner scanner = new Scanner(System.in); System.out.print("Enter message: "); inputString = scanner.nextLine(); //PASSES INPUT STRING TO FIND ENCODE LENGTH METHOD AND DECODE RLE METHOD Menu(); break; case 2: System.out.println("The encoded data is: " /* + encoded string*/); break; case 3: System.out.println("The decoded data is: "/* + decoded string*/); break; case 4: System.out.println("Program terminated"); //EXITS PROGRAM System.exit(0); break; default: System.out.println("Error! Invalid input. Please enter an integer from 1-4."); //ERROR CATCHINF System.out.println(""); Menu(); break; } } /////////////////////////////////////////////////////////////////////////////////////////// public static int numOfDigits(int num) { //METHOD WORKS int index = 0; int numOfDigits; String numString = Integer.toString(num); //CONVERTS INT NUM TO STRING NUM while (index < numString.length()) { //LOOP TO COUNT ITERATE THROUGH STRING index++; } numOfDigits = index; //GETS AMOUNT OF CHARACTERS IN STRING return numOfDigits; } ////////////////////////////////////////////////////////////////////////////////////////////////////////////// public static char[] toCharArray(int charCount, char strChar) { //??????????????????????? if(charCount == 1) //no need to store count if its 1 { char[] charArray = new char[1]; charArray[0] = strChar; return charArray; } int digits = numOfDigits(charCount); char[] charArray = new char[digits + 1]; //1 extra for the char to be stored in end String s = "" + charCount; for(int i = 0; i < s.length(); i++) charArray[i] = s.charAt(i); charArray[digits] = strChar; return charArray; } ///////////////////////////////////////////////////////////////////////////////////////// public static int findEncodeLength(String inputString) { //WORKS boolean[] isSameChar = new boolean[Character.MAX_VALUE]; for (int i = 0; i < inputString.length(); i++) { isSameChar[inputString.charAt(i)] = true; } int findEncodeLength = 0; for (int i = 0; i < isSameChar.length; i++) { if (isSameChar[i] == true) { findEncodeLength++; } } return findEncodeLength; } ////////////////////////////////////////////////////////////////////////////////// public static int findDecodeLength(String rleString) { //NOT WORKING CORRECTLY, DEBUG int findDecodeLength = 0; for (int i = 0; i < rleString.length(); i++) { if (Character.isDigit(rleString.charAt(i)) && !Character.isDigit(rleString.charAt(i++))) { i--; int asciiValue = Integer.valueOf(rleString.charAt(i)); int numericValue = Character.getNumericValue(asciiValue); findDecodeLength = findDecodeLength + numericValue; } if ((Character.isDigit(rleString.charAt(i)) && (Character.isDigit(rleString.charAt(i++))))) { i--; int asciiValue = Integer.valueOf(rleString.charAt(i)); int asciiValue2 = Integer.valueOf(rleString.charAt(i++)); i--; int numericValue = (Character.getNumericValue(asciiValue)) * 10; int numericValue2 = Character.getNumericValue(asciiValue2); int totalValue = numericValue + numericValue2; findDecodeLength = findDecodeLength + totalValue; //list.add(totalValue); } if (!Character.isDigit(rleString.charAt(i))) { findDecodeLength++; } } return findDecodeLength; } ////////////////////////////////////////////////////////////////////////////////// public static char[] decodeRLE(String rleString) { //WORKS StringBuilder sb = new StringBuilder(); char[] rleChars = rleString.toCharArray(); int i = 0; while (i < rleChars.length) { int repeat = 0; while ((i < rleChars.length) && Character.isDigit(rleChars[i])) { repeat = repeat * 10 + rleChars[i++] - '0'; } StringBuilder s = new StringBuilder(); while ((i < rleChars.length) && !Character.isDigit(rleChars[i])) { s.append(rleChars[i++]); } if (repeat > 0) { for (int j = 0; j < repeat; j++) { sb.append(s.toString()); } } else { sb.append(s.toString()); } } String decodeRLEstring = sb.toString(); char[] decodeRLE = decodeRLEstring.toCharArray(); return decodeRLE; } ///////////////////////////////////////////////////////////////////////////////////// public static char[][] encodeRLE(String inputString) { int length = findEncodeLength(inputString); char[][] encodeRLEarray = new char[length][]; char current = inputString.charAt(0); int count = 1; int index = 0; for(int i = 1; i < inputString.length(); i++) { char ch = inputString.charAt(i); if(ch != current) { encodeRLEarray[index++] = toCharArray(count, current); current = ch; count = 1; } else count++; } encodeRLEarray[index++] = toCharArray(count, current); //store the last character processed return encodeRLEarray; } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
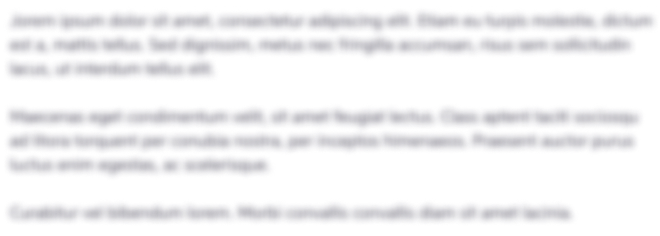
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started