Question
I am currently working in Visual Studios 2019 C++. These are the instructions that follow: Create an array variable to store string values. Make this
I am currently working in Visual Studios 2019 C++. These are the instructions that follow:
Create an array variable to store string values. Make this array variable so it can store up to 50 values in it. When declaring that array variable, use a constant int to allocate the size.
Create an input file variable to read in the class name data. Create an output file variable to store output
Use a loop of your choice to read in an unknown number of values from your input file and store that data into the array variable you created in step 1 (unknown number values: this means the number of data items stored in the file size is not static. It can change each time the program executes)
Use getline to read in string values from your input file so you may capture values with spaces in them
After reading in the class names from the input file and storing them into your array variable, output them to both the screen and an output file in the original order and again in reverse order
Use functions to do the following:
Check to see if the file was found
Read in the class names
Output the class names to the screen in the original order
Output the class names in reverse order
Close the files
This is my following code:
#include
using namespace std;
int main() {
ifstream iFile;
iFile.open("input.txt");
if (!iFile.is_open()) { cout << "Input file not found" << endl; exit(1);
}
string name[50]; int pageNum = 0;
while (!iFile.eof()) { getline(iFile, name[pageNum]);
pageNum++; }
for (int a = 0; a < pageNum; a++) { cout << "Student Name: " << name[a] << endl; }
cout << endl << endl;
for (int a = pageNum-1; a >=0; a--) { cout << "Student Name: " << name[a] << endl; }
iFile.close();
}
I was wondering how to implement these into functions without using global variables.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
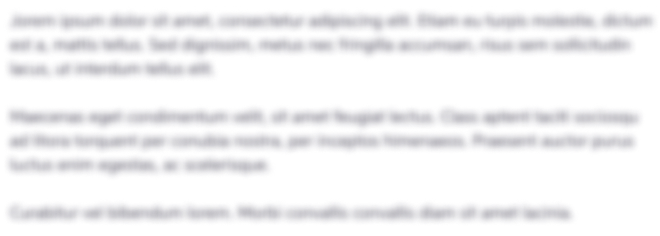
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started