Question
I am getting complier errors from the code I posted a screenshot at the bottom, edit the mistakes found in the screenshot Source code for
I am getting complier errors from the code I posted a screenshot at the bottom, edit the mistakes found in the screenshot
Source code for main.cpp:
#include
int main() {
int numStudents = 5;
const string studentData[5] = { "A1,John,Smith,John1989@gm ail.com,20,30,35,40,SECURITY", "A2,Suzan,Erickson,Erickson_1990@mailcom,19,50,30,40,NETWORK", "A3,Jack,Napoli,The_lawyer99yahoo.com,19,20,40,33,SOFTWARE", "A4,Erin,Black,Erin.black@comcast.net,22,50,58,40,SECURITY", "A5,Sam,Burns,sbur117@wgu.edu,29,10,20,30,SOFTWARE", };
Roster* ros = new Roster(numStudents); for (int i = 0; i parseThenAdd(studentData[i]); }
cout printAll(); cout
ros->printInvalidEmails(); cout printAverageDaysInCourse(ros->getStudent(i)->getStudentID()); } cout
ros->printByDegreeProgram(DegreeProgram::SOFTWARE); cout remove("A3"); cout
cout printAll(); cout
cout remove("A3"); cout
system("pause");
return 0; }
Source code for degree.h:
#pragma once #include
//Define an enumerated data type DegreeProgram, data type values SECURITY, NETWORK and SOFTWARE enum DegreeProgram {SECURITY,NETWORK,SOFTWARE};
static const std::string DegreeProgramStrings[] = { "SECURITY", "NETWORK", "SOFTWARE" };
Source code for roster.cpp:
#include
Roster::Roster() { this->maxSize = 0; this->lastIndex = -1; this->classRosterArray = nullptr; }
Roster::Roster(int maxSize) { this->maxSize = maxSize; this->lastIndex = -1; this->classRosterArray = new Student * [maxSize]; }
void Roster::parseThenAdd(string row) { int parseArr[Student::tableSize]; DegreeProgram degreeprogram;
if (lastIndex classRosterArray[lastIndex] = new Student();
//Parse through the student data strong deliminated by comma //read student ID int long rhs = row.find(","); classRosterArray[lastIndex]->setID(row.substr(0, rhs));
//read firstName int long lhs = rhs + 1; rhs = row.find(",", lhs); classRosterArray[lastIndex]->setFirst(row.substr(lhs, rhs - lhs));
//read lastName lhs = rhs + 1; rhs = row.find(",", lhs); classRosterArray[lastIndex]->setLast(row.substr(lhs, rhs - lhs));
//read emailAddress lhs = rhs + 1; rhs = row.find(",", lhs); classRosterArray[lastIndex]->setEmail(row.substr(lhs, rhs - lhs));
//read age lhs = rhs + 1; rhs = row.find(",", lhs); int x = stoi(row.substr(lhs, rhs - lhs)); classRosterArray[lastIndex]->setAge(x);
//read daysInCourse1 lhs = rhs + 1; rhs = row.find(",", lhs); parseArr[0]= stoi(row.substr(lhs, rhs - lhs));
//read daysInCourse2 lhs = rhs + 1; rhs = row.find(",", lhs); parseArr[1] = stoi(row.substr(lhs, rhs - lhs));
//read daysInCourse3 lhs = rhs + 1; rhs = row.find(",", lhs); parseArr[2] = stoi(row.substr(lhs, rhs - lhs));
//set the days left in course classRosterArray[lastIndex]->setDaysToComplete(parseArr);
//read degree program lhs = rhs + 1; if (row[lhs] == 'S') { if (row[lhs + 1] == 'E') classRosterArray[lastIndex]->setDegreeProgram(SECURITY); else if (row[lhs + 1] == 'O') classRosterArray[lastIndex]->setDegreeProgram(SOFTWARE); else { cerr setDegreeProgram(NETWORK); else { cerr
void Roster::add(string studentID, string firstName, string lastName, string emailAddress, int age, int daysInCourse1, int daysInCourse2, int daysInCourse3, DegreeProgram degreeprogam) { int arrDaysToComplete[Student::tableSize];
arrDaysToComplete[0] = daysInCourse1; arrDaysToComplete[1] = daysInCourse2; arrDaysToComplete[2] = daysInCourse3;
classRosterArray[lastIndex] = new Student(studentID, firstName, lastName, emailAddress, age, arrDaysToComplete, degreeprogam); }
Student* Roster::getStudent(int index) { return classRosterArray[index]; }
void Roster::printAll() { for (int i = 0; i lastIndex; i++) (this->classRosterArray)[i]->print(); }
bool Roster::remove(string ID) { bool found = false; for (int i = 0; i classRosterArray[i]->getStudentID() == ID) { found = true; delete this->classRosterArray[i]; this->classRosterArray[i] = this->classRosterArray[lastIndex]; lastIndex--; } } if (!found) { cout
void Roster::printAverageDaysInCourse(string sID) { bool found = false; for (int i = 0; i classRosterArray[i]->getStudentID() == sID) { found = true; int* ptr = classRosterArray[i]->getDaysToComplete(); cout
void Roster::printInvalidEmails() { cout getEmailAddress(); if ((email.find("@") == string::npos || email.find(".") == string::npos) || (email.find(" ") != string::npos)) cout
void Roster::printByDegreeProgram(DegreeProgram degreeprogram) { cout classRosterArray[i]->getDegreeProgram() == degreeprogram) this->classRosterArray[i]->print(); } }
Roster::~Roster() { for (int i = 0; i classRosterArray[i]; } delete this; }
Source code for roster.h:
#pragma once #include "student.h" #include
class Roster { public: int lastIndex; int maxSize = 5; Student** classRosterArray; //array of pointers to hold data
//default constructor Roster(); Roster(int maxSize);
void parseThenAdd(string row); void add( string studentID, string firstName, string lastName, string emailAddress, int age, int daysInCourse1, int daysInCourse2, int daysInCourse3, DegreeProgram degreeprogam );
Student* getStudent(int index); void printAll(); bool remove(string studentID); void printAverageDaysInCourse(string studentID); void printInvalidEmails(); void printByDegreeProgram(DegreeProgram degreeprogram); ~Roster(); };
Source code for student.cpp:
#include "student.h" #include
/*** Student class functions ***/ Student::Student() //Empty constructor { this->studentID = ""; this->firstName = ""; this->lastName = ""; this->emailAddress = ""; this->age = 0; this->arrDaysToComplete = new int[tableSize]; for (int i = 0; i arrDaysToComplete[i] = 0; this->dprog = SOFTWARE;
}
Student::Student(string studentID, string firstName, string lastName, string emailAddress, int age, int arrDaysToComplete[], DegreeProgram dprog) { this->studentID = studentID; this->firstName = firstName; this->lastName = lastName; this->emailAddress = emailAddress; this->age = age; this->arrDaysToComplete = new int[tableSize]; for (int i = 0; i arrDaysToComplete[i] = arrDaysToComplete[i]; this->dprog = dprog; }
//Accessor functions string Student::getStudentID() { return studentID; }
string Student::getFirstName() { return firstName; }
string Student::getLastName() { return lastName; }
string Student::getEmailAddress() { return emailAddress; }
int Student::getAge() { return age; }
int* Student::getDaysToComplete() { return arrDaysToComplete; //pointer to an array }
DegreeProgram Student::getDegreeProgram() { return dprog; }
//Mutator functions
void Student::setID(string studentID) { this->studentID = studentID; }
void Student::setFirst(string firstName) { this->firstName = firstName; }
void Student::setLast(string lastName) { this->lastName = lastName; }
void Student::setEmail(string emailAddress) { this->emailAddress = emailAddress; }
void Student::setAge(int age) { this->age = age; }
void Student::setDaysToComplete(int arrDaysToComplete[]) { for (int i = 0; i arrDaysToComplete[i] = arrDaysToComplete[i]; } }
void Student::setDegreeProgram(DegreeProgram dprog) { this->dprog = dprog; }
void Student::print() { cout
//Destructor Student::~Student() { if (arrDaysToComplete != nullptr) { delete[] arrDaysToComplete; arrDaysToComplete = nullptr; } }
Source code for student.h:
#pragma once #include "degree.h" #include
/*** Student class definition ***/ class Student { public: const static int tableSize = 3;
private: /eeds to be privat public or protected??~ string studentID; string firstName; string lastName; string emailAddress; int age; int * arrDaysToComplete; DegreeProgram dprog; public: //default constructor Student(); //full constructor Student( string studentID, string firstName, string lastName, string emailAddress, int age, int arrDaysToComplete[], DegreeProgram dprog );
//accessors for each instance string getStudentID(); string getFirstName(); string getLastName(); string getEmailAddress(); int getAge(); int* getDaysToComplete(); DegreeProgram getDegreeProgram();
//mutators void setID(string studentID); void setFirst(string firstName); void setLast(string lastName); void setEmail(string emailAddress); void setAge(int age); void setDaysToComplete(int arrDaysToComplete[]); void setDegreeProgram(DegreeProgram dprog); void print();
//desturctor ~Student();
};
Step by Step Solution
There are 3 Steps involved in it
Step: 1
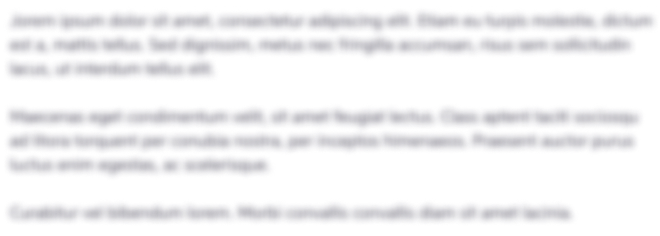
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started