Question
I am getting this error for my program and i do not know how to fix it, I will provide all the programs that are
I am getting this error for my program and i do not know how to fix it, I will provide all the programs that are needed to run it, if anyone can show me how to fix it ill be grateful.
QwirkleHandTest.java:7: error: incompatible types: Tile cannot be converted to int qwirkleHand = TileNode.insertAtHead( qwirkleHand, new TileNode(game1.draw( ), null));
TileNode.Java
public class TileNode{
// instance variables private int data; private TileNode link; public TileNode() {} // constructor public TileNode(int initialData, TileNode initialLink) { data = initialData; link = initialLink; } // the calling object is a reference to the // node before the location where I want to add public void addNodeAfter(int item){ link = new TileNode(item, link); } public int getData( ) { return data; } public TileNode getLink( ){ return link; } public static TileNode[ ] split ( TileNode original, int sValue ) { TileNode cursor = original; TileNode answer[ ] = new TileNode [ 2 ]; TileNode list1=null, list1cursor=null; TileNode list2=null, list2cursor=null; while ( cursor != null ) { if ( cursor.getData() < sValue ) { if ( list1cursor == null) { // list 1 is empty list1 = new TileNode( cursor.getData(), null ); list1cursor = list1; } else { list1cursor.setLink(new TileNode(cursor.getData(), null)); list1cursor = list1cursor.getLink(); } cursor = cursor.link; } // end if data < sValue else { if ( list2cursor == null) { // list 2 is empty list2 = new TileNode( cursor.getData(), null ); list2cursor = list2; } else { list2cursor.setLink(new TileNode(cursor.getData(), null)); list2cursor = list2cursor.getLink(); } cursor = cursor.link; } // end data >= splitValue } // end while answer[0] = list1; answer [1] = list2; return answer; }// end method public static String listToString( TileNode head ) { String answer = ""; TileNode cursor = head; while (cursor != null) { answer = answer + cursor.getData(); if (cursor.getLink() == null) answer = answer + " End of List"; else answer = answer + " --> "; cursor = cursor.getLink(); } return answer; } // call this method using IntNode.listCopy(reference to the head) // it will duplicate the list and return a reference which // is the head of the new list public static TileNode listCopy(TileNode source){ TileNode copyHead; TileNode copyTail; // Handle the special case of the empty list. if (source == null) return null; // Make the first node for the newly created list. copyHead = new TileNode(source.data, null); copyTail = copyHead; // Make the rest of the nodes for the newly created list. while (source.link != null){ source = source.link; copyTail.addNodeAfter(source.data); copyTail = copyTail.link; } // Return the head reference for the new list. return copyHead; } public static int listLength(TileNode head){ int answer = 0; for (TileNode cursor = head; cursor != null; cursor = cursor.link) answer++; return answer; } // search for a particular data value in the list // return a reference to the node where the value // was found // if the value isn't in the list, return null public static TileNode listSearch(TileNode head, int target){ TileNode cursor; for (cursor = head; cursor != null; cursor = cursor.link) if (target == cursor.data) return cursor; return null; } // this method is called using the node // that is before the one we want to remove public void removeNodeAfter( ){ link = link.link; } // mutator public void setData(int newData){ data = newData; } public void setLink(TileNode newLink){ link = newLink; } //added portion for the lab public static void printList(TileNode head){ if(head == null) return; TileNode node = head; while(true) { System.out.println(node.data); if(node.link != null) node=node.link; else break; } } public static TileNode insertAtHead(TileNode head, TileNode tile){ TileNode node = new TileNode(); node.data = tile.data; node.link = head; return node; } public static TileNode reverse(TileNode original){ TileNode prev = null; TileNode cur = original; TileNode link = null; while(cur != null) { link = cur.link; cur.link = prev; prev = cur; cur = link; } original = prev; return original; } }
QwirkleHandTester.Java
class QwirkleHandTes{ public static void main(String[] args) { TileNode qwirkleHand = null; QwirkleBag game1 = new QwirkleBag(); //Draw 6 tiles out of the bag, and add them to the player's hand. for(int t = 1; t <= 6; t++) qwirkleHand = TileNode.insertAtHead( qwirkleHand, new TileNode(game1.draw( ), null)); //Where the error is occuring //Display the tiles in the player's hand. TileNode.printList(qwirkleHand); } }
QwirkleBag.Java
public class QwirkleBag extends Object implements Cloneable {
// instance variables
private Tile[ ] data; private int manyItems;
// constructor : behavior #1
public QwirkleBag( ) { final int INITIAL_CAPACITY = 108;
manyItems = 108;
data = new Tile[INITIAL_CAPACITY]; for (int i = 0; i < INITIAL_CAPACITY; i++) data[i] = new Tile( i % 6 + 1, i % 36 / 6 + 1 ); } public String toString() { String answer = ""; for (int i = 0; i < manyItems; i++) answer = answer + String.format("%3d",i+1) + ": " + data[i].toString() + " "; return answer; }
// This method was added so that the entire contents of the // data array could be displayed. // // The behavior is different from toString because toString // only includes tiles up to manyItems public void printAll(int numPerLine, boolean printCompleteArray) { int lastIndex = (printCompleteArray ? data.length : manyItems); for (int index = 0; index < lastIndex; index++) System.out.print( data[index] + " " + ((index+1)%numPerLine == 0 ? " " : "")); } // end method // add one item : behavior #2
public void add(Tile element) { if (manyItems == data.length) { // Ensure twice as much space as we need. ensureCapacity((manyItems + 1)*2); }
data[manyItems] = element.clone(); manyItems++; }
// Clone an QwirkleBag object. public QwirkleBag clone( ) { QwirkleBag answer; try { answer = (QwirkleBag) super.clone( ); } catch (CloneNotSupportedException e) { throw new RuntimeException ("This class does not implement Cloneable"); } answer.data = data.clone( ); return answer; }
public int countOccurrences(Tile target) { int count = 0; for (int index = 0; index < manyItems; index++) if (target.equals(data[index])) count++;
return count; } public void ensureCapacity(int minimumCapacity) { Tile biggerArray[ ]; // declaration if (data.length < minimumCapacity) { biggerArray = new Tile[minimumCapacity]; // allocate space
for (int index = 0; index < manyItems; index++ ) biggerArray[index] = data[index]; data = biggerArray; } }
public int getCapacity( ) { return data.length; } // This method was added so that a Tile at a specific position // (index) in the data array can be retrieved from the bag. public Tile tileAt( int pos ) { if (pos >= manyItems) return null; return data[pos]; }
public boolean remove(Tile target) { int index; // must declare before the loop
for (index = 0; (index < manyItems) && (!target.equals(data[index])); index++) ; if (index == manyItems) // target was not found return false; else { manyItems--; data[index] = data[manyItems]; return true; } } public int size( ) { return manyItems; } // end size public void trimToSize( ) { Tile trimmedArray[ ]; if (data.length != manyItems) { trimmedArray = new Tile[manyItems]; for (int index = 0; index < manyItems; index++) trimmedArray[index] = data[index]; data = trimmedArray; } } // end trimToSize public Tile draw( ) { if (manyItems == 0) return null; int pick = (int) (Math.random() * manyItems); Tile chosen = data[pick]; data[pick] = data[manyItems-1]; manyItems--; return chosen; }// end draw } // end class
Tile.Java
public class Tile implements Cloneable {
// instance variables private int color; private int shape; // Behavior #1: constructor public Tile ( int c, int s ) {
// if invalid parameter values, default to 1 if (c >= 1 && c <= 6) color = c; else color = 1;
if (s >= 1 && s <= 6) shape = s; else shape = 1; } // end constructor // Behavior #2 : accessor for color public int getColor( ) { return color; } // Behavior #3 : accessor for shape public int getShape( ) { return shape; } // Behavior #4 : mutator for color public void setColor( int c ) {
// doesn't change color if c is invalid if ( c >= 1 && c <= 6 ) color = c; } // Behavior #5 : mutator for shape public void setShape( int s ) {
// doesn't change shape if s is invalid if ( s >= 1 && s <= 6 ) shape = s; }
// Behavior #6 : String representation of a Tile public String toString( ) { String colorNames[] = {"orange", "green", "yellow", "red", "blue", "purple"}; String shapeNames[] = {"four-pointed star", "clover", "eight-pointed star", "diamond", "square", "circle"}; if (color < 1 || color > 6 || shape < 1 || shape > 6) return "unknown"; return colorNames[color-1] + " " + shapeNames[shape-1]; } // Behavior # 7 : compare two tiles public boolean equals (Tile other) { return shape == other.shape && color == other.color; } // Behavior #8 : clone a tile public Tile clone( ) { Tile answer; try { answer = (Tile) super.clone(); } catch (CloneNotSupportedException e) { throw new RuntimeException ("This class doesn't support Cloneable."); } return answer; } } // end Tile Class
Step by Step Solution
There are 3 Steps involved in it
Step: 1
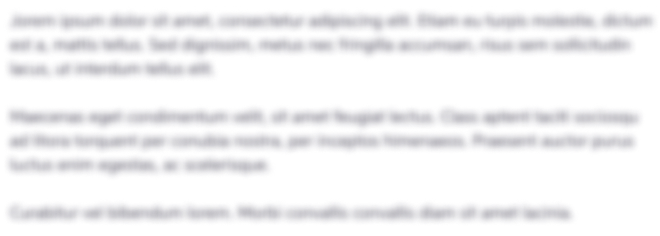
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started