Question
I am given an input file, P1input.txt and I have to write code to find the min and max, as well as prime and perfect
I am given an input file, P1input.txt and I have to write code to find the min and max, as well as prime and perfect numbers from the input file. P1input.txt contains a hundred integers. Why doesn't my code compile properly to show me all the numbers? It just stops and displays usage: C:\> java Project1 P1input.txt 1 30
import java.io.*; // BufferedReader import java.util.*; // Scanner to read from a text file
public class Project1 { public static void main (String args[]) throws Exception // we NEED this 'throws' clause { // ALWAYS TEST FIRST TO VERIFY USER PUT REQUIRED CMD ARGS if (args.length < 3) { System.out.println(" usage: C:\\> java Project1 P1input.txt 1 30 "); // i.e. C:\> java Project1.txt P1input.txt 1 30 System.exit(0); }
String infileName = args[0]; // no need to convert, just copy into infileName int lo = Integer.parseInt( args[1] ); // conver to int then store into lo int hi = Integer.parseInt( args[2] ); // conver to int then store into hi
// STEP #1: OPEN THE INPUT FILE AND COMPUTE THE MIN AND MAX. NO OUTPUT STATMENTS ALLOWED Scanner infile = new Scanner( new File(infileName) ); int min,max; min=max=infile.nextInt(); // WE ASSUME INPUT FILE HAS AT LEAST ONE VALUE while ( infile.hasNextInt() ) { // YOUR CODE HERE FIND THE MIN AND MAX VALUES OF THE FILE // USING THE LEAST POSSIBLE NUMBER OF COMPARISONS // ASSIGN CORRECT VALUES INTO min & max INTHIS LOOP. // MY CODE BELOW WILL FORMAT THEM TO THE SCREEN // DO NOT WRITE ANY OUTPUT TO THE SCREEN int num = infile.nextInt();
if(num < min) min = num;
if(num > max) max = num; }
System.out.format("min: %d max: %d ",min,max); // DO NOT REMOVE OR MODIFY IN ANY WAY
// STEP #2: DO NOT MODIFY THE REST OF MAIN. USE THIS CODE AS IS // WE ARE TESTING EVERY NUMBER BETWEEN LO AND HI INCLUSIVE FOR // BEING PRIME AND/OR BEING PERFECT for (int i=lo ; i<=hi ; ++i) { System.out.print( i ); if ( isPrime(i) ) System.out.print( " prime "); if ( isPerfect(i) ) System.out.print( " perfect "); System.out.println(); } } // END MAIN
// *************** YOU FILL IN THE METHODS BELOW **********************
// RETURNs true if and only if the number passed in is perfect static boolean isPerfect( int n ) { int sum = 0; for (int i =1; i <= n/2; i++) { if(n % i == 0) sum += i; }
if (sum == n) return true;
else return false; // (just to make it compile) YOU CHANGE AS NEEDED } // RETURNs true if and only if the number passed in is prime static boolean isPrime( int n ) { for (int f = 2; f <= n/2; f++) { if (n % f == 0) return false;
} return true; // (just to make it compile) YOU CHANGE AS NEEDED } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
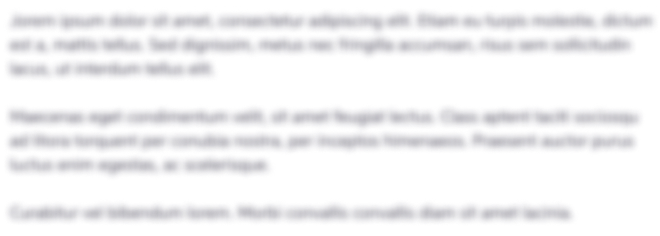
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started