Question
I am having a bit of trouble getting this Java program to work. If anyone can solve this so I have a guideline on how
I am having a bit of trouble getting this Java program to work. If anyone can solve this so I have a guideline on how to break something like this down it would be helpful. The Check
Input file should be in a separate class to check input validation. Thank you!
Lecture notes, Copy Paste:
/** This class is a Player that uses a Die object */ public class Player { /** A die that the player may roll */ private Die d; /** The number of points the player has */ private int points; /** Constructor = create a new 6 sided die */ public Player( ) { d = new Die( 6 ); points = 0; } /** Player takes turn if 1 is rolled then points * reset to 0, otherwise add die value to points */ public void takeTurn( ) { int p = d.roll( ); if( p == 1 ) { points = 0; } else { points += p; } } /** Retreives the points value * @return players points */ public int getPoints( ) { return points; } }
Implementation the main portion of your program, where your objects may interact.
public class Game { public static void main ( String[] args ) { Player p1 = new Player( ); Player p2 = new Player( ); for( int i = 0; i p2.getPoints( ) ) { System.out.println( Player 1 Wins ); } else if ( p2.getPoints( ) > p1.getPoints( ) ) { System.out.println( Player 2 Wins ); } else { System.out.println( Tied ); } } }
Check Input File (make a separate Class in project) //call methods in here to check validity of input
import java.util.Scanner;
/**
* Static functions used to check console input for validity.
*
* Use: Place CheckInput class in the same project folder as your code.
* Call CheckInput functions from your code using "CheckInput."
*
* Example: int num = CheckInput.getInt();
*
* @author Shannon Cleary 2018
*/
public class CheckInput {
/**
* Checks if the inputted value is an integer.
* @return the valid input.
*/
public static int getInt() {
Scanner in = new Scanner( System.in );
int input = 0;
boolean valid = false;
while( !valid ) {
if( in.hasNextInt() ) {
input = in.nextInt();
valid = true;
} else {
in.next(); //clear invalid string
System.out.println( "Invalid Input." );
}
}
return input;
}
/**
* Checks if the inputted value is an integer and
* within the specified range (ex: 1-10)
* @param low lower bound of the range.
* @param high upper bound of the range.
* @return the valid input.
*/
public static int getIntRange( int low, int high ) {
Scanner in = new Scanner( System.in );
int input = 0;
boolean valid = false;
while( !valid ) {
if( in.hasNextInt() ) {
input = in.nextInt();
if( input = low ) {
valid = true;
} else {
System.out.println( "Invalid Range." );
}
} else {
in.next(); //clear invalid string
System.out.println( "Invalid Input." );
}
}
return input;
}
/**
* Checks if the inputted value is a non-negative integer.
* @return the valid input.
*/
public static int getPositiveInt( ) {
Scanner in = new Scanner( System.in );
int input = 0;
boolean valid = false;
while( !valid ) {
if( in.hasNextInt() ) {
input = in.nextInt();
if( input >= 0 ) {
valid = true;
} else {
System.out.println( "Invalid Range." );
}
} else {
in.next(); //clear invalid string
System.out.println( "Invalid Input." );
}
}
return input;
}
/**
* Checks if the inputted value is a double.
* @return the valid input.
*/
public static double getDouble() {
Scanner in = new Scanner( System.in );
double input = 0;
boolean valid = false;
while( !valid ) {
if( in.hasNextDouble() ) {
input = in.nextDouble();
valid = true;
} else {
in.next(); //clear invalid string
System.out.println( "Invalid Input." );
}
}
return input;
}
/**
* Takes in a string from the user.
* @return the inputted String.
*/
public static String getString() {
Scanner in = new Scanner( System.in );
String input = in.nextLine();
return input;
}
/**
* Takes in a yeso from the user.
* @return 1 if yes, 0 if no.
*/
public static int getYesNo(){
boolean valid = false;
while( !valid ) {
String s = getString();
if( s.equalsIgnoreCase("yes") || s.equalsIgnoreCase("y") ) {
return 1;
} else if( s.equalsIgnoreCase("no") || s.equalsIgnoreCase("n") ) {
return 0;
} else {
System.out.println( "Invalid Input." );
}
}
return 0;
}
}
Yacht-z - Create a dice game that uses the Die class that was given in the lecture notes Create a new class called Die in a file called Die.java', add the code from the lecture notes, and then add an equals and a compareTo method. In the same project folder, create a second class called Player in a file called Player java' (the code for this class is not the same as the lecture notes). Then, again in the same project folder, create a third class for your main. The player rolls a set of 3 dice. If two of the dice are the same then the player receives 1 point for a pair. If all of the dice are the same, then the player receives 3 points for 3-of-a-kind (note if a player gets a 3-of-a-kind they shouldn't also get points for having a pair). If the dice values are in a series, then the player receives 2 points (ex. 1-2-3, 3-2-1,2-1-3, or any other order of these values would be considered a series) Create an array of three dice objects and a variable for the player's points in your Player class In the constructor, loop through and call the constructor for each of the dice to initialize them Create a sort method that arranges the dice in increasing sorted order that uses the compareTo method you wrote in the Die class. Add methods to check for each of the three different win types that use the Die's compareTo and equals methods, return true if they met the win condition. Add a toString method for your player class that will be used to display the dice roll (ex. D1-2, D2-4 D3-6). Add a get method for the point value. Create a method that called takeTurn that rolls the dice, prints their values using the toString0 function you wrote (you can use System.out.println(this) to call it from within takeTurn), and determines if the player met any of the win conditions, if so, then accumulate those points in their point total and display it Ask the player if they want to continue playing after every roll, display their final points when they decide to quit. Perform error checking and use methods appropriately to create your program Example Output Rolling Dice...D1-, D2-4,D3-4 You got 3 of a kind! Score6 points. Play again? (/N) Rolling Dice...D1-1, D2-3,D3-6 Awww. Too Bad. Score6 points. Play again? (/N) Game Over. Final Score-6 points Yacht-z Rolling Dice...D1-2,D2-3,D3-5 AWNw.TooBad. Score0 points. Play again? (/N) X Invalid input. Play again? (/N) Y Rolling Dice D1-2,D2-3, D3-4 You got a series of 3! Score 2 points. Play again? (/N) Rolling Dice. . .D1-1,D2-1,D3-3 You got a pair! Score 3 points. Play again? (Y/N)YStep by Step Solution
There are 3 Steps involved in it
Step: 1
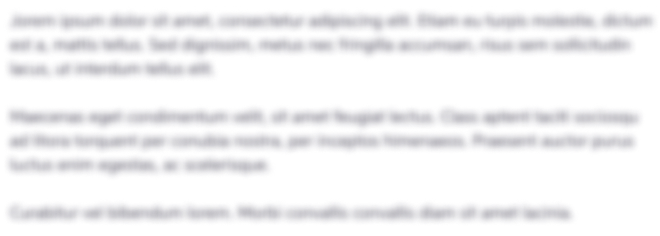
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started