Question
//I am having a hard time figuring out REQ 2 and how to do it without using the java.util.Random function, also for REQ3 when the
//I am having a hard time figuring out REQ 2 and how to do it without using the java.util.Random function, also for REQ3 when the answer is wrong it does not repeat and ask the same question again.
/* This file includes:
* 1. Solution to P3
* 2. Questions for P4. Comments starting with REQ represent the questions.
*
* Features:
* - We have from 1 to 3 players
* - We have many questions. Each player may be asked more than one question.
* - User can play many rounds of the game.
*
* Focus:
* - Arrays and Methods
*
* Aim:
* - Organize code and avoid code redundancy by the use of methods
*/
public class Main {
static Game game;
//Two arrays for questions and answers (both are global, i.e., accessible by all code in the class).
//REQ1: Replace array values with real questions/answers
static String[] questions = {"What is the Capital of India?", "What Chemical Elemant is K?", "What year did WWII End?", "How Many Provinces in Canada?", "First Prime Minister of Canada", "Name the world's largest ocean?", "What Sport is popular in Canada", "How many Plamets in our Solar System?", "Which planet is closest to the sun?"};
static String[] answers = {"New Delhi", "Potassium", "1945", "10", "John MacDonald", "Pacific", "Hockey", "8", "Mercury"};
public static void main(String[] args) {
String ans;
do{
//Reset the game
game = new Game();
//Get number of players (from 1 to 3)
int numPlayers = game.askForInt("How many players", 1, 3);
//Add up to 3 players to the game
for (int i = 0; i < numPlayers; i++) {
String name = game.askForText("What is player " + i + " name?");
game.addPlayer(name);
}
//REQ2: Call a method to shuffle questions and answers. For that, you need to create a method with the header: void shuffleQuestions();
shuffleQuestions();
//REQ3: - Calculate the maximum number of questions each player could be asked (equals to the number of available questions divided by numPlayers). Store this value in a variable maxQuestions
// - Ask the user how many questions should be given to each player. The value read from the user should not exceed MaxQuestion. Store this value in a variable numQuestions.
// - Ask each player the next unanswered question (e.g., player 0 gets the first question. If it is answered correctly, then player1 gets the next question in the array, otherwise player1 gets the same question again, and so on).
// Assume that an incorrectly answered question will keep popping up until it is correctly answered.
// Hint: you need to create a for loop that repeats the below code block numQuestions times.
// Hint: you need to have a variable that keeps track of the next question to be offered.
int maxQuestions=questions.length/numPlayers;
//Get number of players (from 1 to maxQuestions)
int numQuestions = game.askForInt("How many questions to each player", 1, maxQuestions);
int questionToAsk=0;
for(int j=0;j
{
for (int i = 0; i < numPlayers; i++) {
game.setCurrentPlayer(questionToAsk);//draw rectangle around player 0, and currentPlayer = player0
String answer = game.askForText(questions[i]);
if(answers[i].equals(answer))
{
game.correct(); //display "Correct", increment score, change frame color to green
questionToAsk++;
}
else
game.incorrect(); //display "incorrect", change frame color of player to red
}
}
//Do you want to play again? make sure you get valid input
ans = game.askForText("Play again? (Y/N)");
while(ans != null && !ans.toUpperCase().equals("Y") && !ans.toUpperCase().equals("N"))
ans = game.askForText("Invalid input. Play again? (Y/N)");
}while(ans.toUpperCase().equals("Y")); //play again if the user answers "Y" or "y"
System.exit(1); //This statement terminates the program
}
//Game class
import java.awt.BasicStroke;
import java.awt.Color;
import java.awt.Font;
import java.awt.FontMetrics;
import java.awt.Graphics2D;
import java.util.ArrayList;
public class Game {
public static final int WIDTH = 800, HEIGHT = 500, MAX_PLAYERS = 3, PLAYER_Y = 50;
protected ArrayList
private GameFrame frame = new GameFrame(this, "COSC 111 Jeopardy", WIDTH, HEIGHT);
private BDialog dialog = new BDialog(frame);
private Player currentPlayer;
private Color responseColor = Color.white;
public Game(){
frame.setLocationRelativeTo(null);
dialog.setLocationRelativeTo(frame);
dialog.setSize(WIDTH-10, 100);
dialog.setLocation(frame.getX()+5, frame.getY() + HEIGHT - 105);
frame.setVisible(true);
}
public void print(String msg){
frame.repaint();
dialog.showMessageDialog(msg);
}
public String askForText(String msg){
frame.repaint();
String response = dialog.showInputDialog(msg);
return (response==null || response.length()==0)? "" : response.trim();
}
public int askForInt(String msg, int min, int max){
msg += "(" + min + " to " + max + ")";
boolean found = false, msgModified = false;
int num = 0;
while(!found){
try{
num = Integer.parseInt(askForText(msg));
if(num
throw new Exception();
found = true;
}catch(Exception e){
if(!msgModified) msg = "Invlid input. " + msg;
msgModified = true;
}
}
return num;
}
public void addPlayer(String name){
Player player = new Player(name);
int playerWidth = player.getImg().getWidth();
int distanceBetweenPlayers = (WIDTH - MAX_PLAYERS * playerWidth) / (MAX_PLAYERS + 1);
int x = (players.size()+1) * distanceBetweenPlayers + players.size() * playerWidth;
player.setX(x);
player.setY(PLAYER_Y);
players.add(player);
frame.repaint();
}
public void clearPlayers(){
players.clear();
}
public void paint(Graphics2D g2){
//draw players
for (int i = 0; i < players.size(); i++)
players.get(i).paint(g2);
//draw frame around selected player
if(currentPlayer != null){
int px = currentPlayer.getX()-4;
int py = currentPlayer.getY()-4;
int pw = currentPlayer.getImg().getWidth()+8;
int ph = currentPlayer.getImg().getHeight()+8;
g2.setStroke(new BasicStroke(8));
g2.setColor(responseColor);
g2.drawRect(px, py, pw, ph);
}
//who is turn it is
if(currentPlayer != null){
g2.setColor(Color.yellow);
String name = currentPlayer.getName() + "'s turn";
Font font = new Font("Arial", Font.BOLD, 28);
g2.setFont(font);
FontMetrics metrics = g2.getFontMetrics(font);
int txtWidth = metrics.stringWidth(name);
g2.drawString(name, (WIDTH-txtWidth)/2, 35);
}
}
public void setCurrentPlayer(int pl) {
currentPlayer = players.get(pl);
responseColor = new Color(255, 160, 255);
}
public void correct() {
responseColor = Color.green;
frame.repaint();
if(currentPlayer != null)
currentPlayer.incrementScore();
print("Correct (press Enter to continue).");
}
public void incorrect() {
responseColor = Color.red;
frame.repaint();
print("Sorry, that is incorrect answer (press Enter to continue).");
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
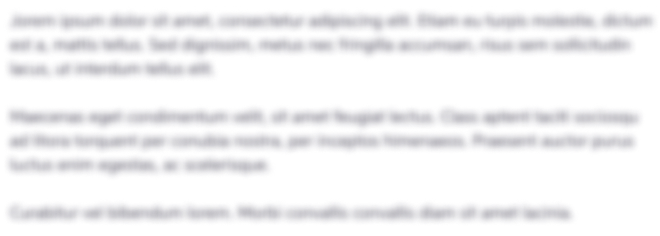
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started