Answered step by step
Verified Expert Solution
Question
1 Approved Answer
I am having difficulty completing this project, in the way, I am supposed to. There are many ways to do this code, I would appreciate
I am having difficulty completing this project, in the way, I am supposed to. There are many ways to do this code, I would appreciate it if someone could help me complete this code in this way. Below are the instructions and my attempt at completing this code, as well as an example of how it's supposed to work:
my attempt:
HELP!!
board_positions.txt In the canvas directions I will have provided a file with 101 numbers inside of it. Download it and put it into your lab folder with the name board_positions.txt on when Inside of this file I have all of the resulting positions of the game board, as in the number you end you land on that space. Important Note All the line numbers are 1 off, so that they line up nicely with the array positions. Line 1 corresponds to space 0 (not on the board) Line 2 corresponds to space 1 (the first space on the board) and so on and so forth. For example On line 1 of the file, I have a 0 indicating the starting space that is not displayed on the game board. In line 2 of the file, representing the 1 spot, I have the value 38. This indicates that when you land on space 1 there is a ladder that will take you to space 38. You can see this ladder on the game board above. On line 5 of the file, representing the 4th spot, I have the value 14. This indicates that there is a ladder here that will take you to the 14th spot. You can see the ladder on the game board above. On non special spots, such as lines 3 and 4, landing on spots 2 and 3 don't take you anywhere, so if you land on 2, you end up on 2 and so on for all normal spots. On line 17 of the file, representing space 16, I have the value 6, indicating that there is a chute here that takes you to space 6 if you land on 16. You can see the chute on the game board above. Step 1: Reading in the Positions Create an array called board_positions that can hold 101 integers. Scan every value from board_positions.txt into this array using a for loop. Step 1: Reading in the Positions Create an array called board_positions that can hold 101 integers. Scan every value from board_positions.txt into this array using a for loop. Display the array backwards, from elements 100 to 1 (don't display element 0) with each element on a single line. Use the following print statement in the loop (We will be changing it soon) printf("%3d ", board_positions[i]); This will right justify all the numbers to have 3 spaces for each number, making them lined up to the right. The output should look something like: 100 99 78 96 ... Many rows omitted for brevity ... 14 3 2 38 Step 2 Fancying up the Board Display Right now we have all the information we need to use the game board, but it doesn't convey much information to the user in a nice way. This step is all about fixing that. First figure out how to go to a new line after every ten numbers instead of printing each number on a new line. (I just use an if statement in my for loop.) The output should now look like this: 100 99 78 97 96 75 94 92 73 83 91 81 89 88 24 86 85 84 90 100 82 72 79 78 77 76 75 74 73 91 69 68 67 66 65 60 63 19 61 70 60 59 58 57 53 55 54 53 52 67 50 11 48 26 46 45 44 43 33 42 32 41 31 40 39 38 37 44 35 34 30 29 84 27 17 26 6 25 15 24 14 22 12 20 19 23 13 3 18 42 11 38 10 31 8 7 6 5 14 2 This is much closer to the actual game board! Now of course some of the rows are backwards and some are forwards in the actual picture, but we aren't going to worry about that. This order is good enough. Step 2.5 Adding Colors! more clear where the chutes and ladders are though, There is one more thing I want to add to make COLORS! It isn't particularly straightforward to make the terminal print colors in C, so I'll just show you how to do it. To print a red number: printf("\x1B[31m %3d \x1B[0m", board_positions[i]); To print a green number: printf("\x1B[32m 83d \x1B[0m", board_positions[i]); Figure out a way (some simple if statements) to print red numbers on spaces where there is a chute, and green numbers on spaces where there is a ladder. After that, your output should look like: 100 90 100 70 60 50 40 30 20 10 99 89 79 69 59 11 39 29 19 31 78 88 78 68 58 48 38 84 18 8 97 24 77 67 57 26 37 27 17 7 96 86 76 66 53 46 44 26 6 6 75 85 75 65 55 45 35 25 15 5 94 84 74 60 54 44 34 24 14 14 73 83 73 63 53 43 33 23 13 3 92 91 82 81 72 91 19 61 52 67 42 41 32 31 22 42 12 11 2 38 Cool! It is now more clear where the chutes and ladders are! Step 3: Adding A Player Essentially all we need to know about a player is what index they are in on they board. All players start at index 0 and move forward by a random amount between 1-6 each turn. The player must land on exactly 100 in order to win. Landing on somewhere past 100 makes you not move at all. When the player lands on the space with the value 100 the game is over. Each turn: Display the board with the player Display the game board (the for loop we finished in step 2.5). Also add some code that displays the player on the index they are on. You can use the following print statement: printf("\x1B[35m A \x1B[0m"); This gives the player a nice magenta color. For example were the player's position to be 25 it would look like: 100 90 100 70 60 50 40 30 20 10 99 89 79 69 59 11 39 29 19 31 78 88 78 68 58 48 38 84 18 8 97 24 77 67 57 26 37 27 17 7 96 86 76 66 53 46 44 26 6 6. 75 85 75 65 55 45 35 A 15 5 94 84 74 60 54 44 34 24 14 14 73 83 73 63 53 43 33 23 13 3 92 82 72 19 52 42 32 22 12 2 91 81 91 61 67 41 31 42 11 38 Have the player make their turn Tell the player where they are on the board (In case they couldn't tell from the board display.) Next tell the user to press enter in order to roll. Since this is a totally luck based game, they don't need to enter anything important. We can wait for the user to press the enter key with the following code: char input; scanf("%c", &input); We aren't going to use the input in any way, scanf just requires that we have a variable there. Next roll a random number between 1 and 6 (Make sure you set up random generation in the right place in your file). Tell the user what they rolled. Move the player Because you aren't allowed to go over 100, next check for values over 100 before you move the player. If the value is over 100, you don't do anything. Step 4 Add an Opponent In order to add a second player to our game, the main thing we need to do is keep track of a second index for our second player that also starts at 0, declare this near where you declared the first players index variable. Update the board display code Next, update your code that displays the game board to also display the second player. On spaces where the second player is, use the following print statement: printf("\x1B[34m B \x1B[0m"); On spaces where both players are there use the following: printf("\x1B[35m A\x1B[34m B \x1B[0m"); Have the second player take their turn: In your main game loop, add the code for the second player to take their turn after the first player has gone. Basically the code for the second player is exactly the same as the first, except that if you want a "computer controlled" player, don't wait for an enter to be pressed. Also don't let the second player roll if the first player has already won in that turn. Speaking of winning, you are going to want to update the main condition of the game loop so that either player winning will stop the game. After the game is over print out which player won. Here are some screenshots from the new stuff: 92 91 82 81 72 91 19 61 52 67 42 41 32 31 42 11 38 w No 100 99 78 97 96 75 73 90 89 88 24 86 85 83 100 79 78 77 76 75 74 73 70 69 68 67 66 65 60 63 60 59 58 57 53 55 54 53 50 11 48 26 46 45 44 43 40 39 38 37 44 35 34 33 30 29 84 27 26 25 20 19 18 17 6 15 10 31 8 7 6 5 You are at space 0 Press enter to roll> You rolled a 1 You moved to 1 You took a ladder to 38 ! The AI is at space 0 The AI rolled a 3 The AI moved to 3 100 99 78 97 96 75 73 90 89 88 24 86 85 83 100 79 78 77 76 75 73 70 69 68 67 66 65 60 63 60 59 58 57 53 55 54 53 50 11 48 26 46 45 44 43 40 39 . 37 44 35 34 33 30 29 84 27 26 25 20 19 18 17 6 10 31 8 7 6 . You are at space 38 Press enter to roll> 92 82 72 19 52 42 91 81 91 61 67 41 31 42 11 38 : NEN 1 //chutes and Ladders 2 #includeStep by Step Solution
There are 3 Steps involved in it
Step: 1
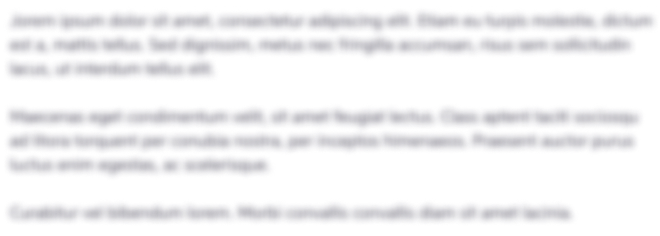
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started