Answered step by step
Verified Expert Solution
Question
1 Approved Answer
I am having issues with my inventory class maybe in the member fields or maybe how im setting up one of the methods... i am
I am having issues with my inventory class maybe in the member fields or maybe how im setting up one of the methods... i am including what i have for my inventory class as well as my item class class Item
TODO: Create an Item class
The class should contain data members:
A string called name
An int called cost.
This class should have a default constructor
that sets name to and cost to
It should also have an overloaded constructor
that accepts parameters:
A string
An int.
Write gettersaccessors for each data member.
They should be called GetName and GetCost.
Write settersmutators for each data member.
They should be called SetName and SetCost.
define attributes
string name;
int cost;
public:
default construcor
Item
set default values to for name and for cost
name ;
cost ;
overloaded constuctor
Itemstring name, int cost
set paramaters to this
thisname name;
thiscost cost;
method to get name
string GetName
return name
return name;
method to get cost
int GetCost
return cost
return cost;
method to set name with name parameter
void SetNamestring name
set parameter to this
thisname name;
method to set cost with cost parameter
void SetCostint cost
set parameter to this
thiscost cost;
;class Inventory
TODO: Define three member fields
An int called mMaxSize
An vector of Items called mItems
An int called mGold
int mMaxSize;
int mGold;
vectormItems;
public:
TODO: Write a default constructor
By default, it should:
Assigns the mMaxSize to
Assign mItems to a new vector of Items with mMaxSize as the size
Set mGold to
Inventory
mMaxSize ;
mGold ;
vectormItems mMaxSize ;
TODO: Create a Getter and a Setter for the mGold field named "GetGold" and "SetGold"
int GetGold
return mGold;
int SetGoldint gold
mGold gold;
TODO: Write a method called AddItem
The method should return a bool returns
a bool and takes an Item parameter.
This method should iterate through the
mItems vector, looking for any vector element
that is default.
If a default Item is found, it should assign
that vector element to the Item passed in and
return true. Otherwise it should return false.
bool AddItemItem newItem
bool result;
for int i ; i mMaxSize; i
if mItemsi Item
mItemsi newItem;
result true;
else
result false;
return result;
TODO: Write a method called RemoveItem
This method should return a bool and takes
a string parameter.
This method should iterate through the
mItems vector, looking for an Item that
has the same name as the parameter.
If it finds a match it should set that
element of the mItems vector to a default
item and return true. Otherwise return false.
bool RemoveItemstring itemName
bool result;
for int i ; i mMaxSize; i
if mItemsi Item && mItemsiGetName itemName
mItemsi Item;
result true;
else
result false;
return result;
TODO: Write a method called GetItem
This method should return an Item and takes
a string parameter.
This method should iterate through the mItems
vector, looking for an Item that has the same
name as the parameter.
If it finds a match it should return that element
of the mItems vector. Otherwise return a default item.
Item GetItemstring itemName
for int i ; i mMaxSize; i
if mItemsi Item && mItemsiGetName itemName
return mItemsi;
TODO: Uncomment the following code:
void DisplayInventoryint x int y
Console::SetCursorPositionxy;
Console::WriteItem Name";
Console::SetCursorPositionx y;
Console::WriteItem Cost";
for int i ; i mMaxSize; i
if mItemsiGetNameempty
Console::SetCursorPositionxy;
Console::WritemItemsiGetName;
Console::SetCursorPositionx y;
Console::Writestd::tostringmItemsiGetCost;
y ;
Console::SetCursorPositionxy;
Step by Step Solution
There are 3 Steps involved in it
Step: 1
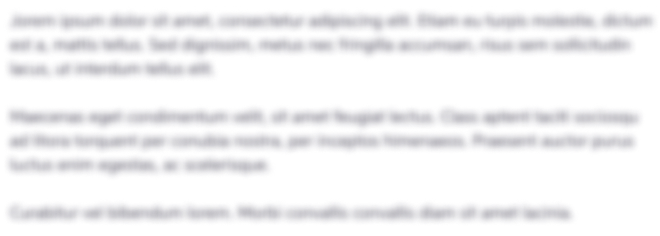
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started