Question
I am having problems designing a java program using NetBeans. The program has to be a GUI one with add note, exit note and exit
I am having problems designing a java program using NetBeans. The program has to be a GUI one with add note, exit note and exit program buttons. It should have a drop-down menu of courses which I can select a course and add notes during lectures, then read it back as a text file. The notes should be stored in folders by date and time. I should be able to search for key words using a search button.
So far, I have created the GUI with the various button, but the issue I am having is how to add notes, store them in folders, search etc. I am posting the codes for what I have done so far. If you could go through it and help me, I would gladly appreciate. I use NetBeans.
==========================================================Code.
/* * To change this license header, choose License Headers in Project Properties. * To change this template file, choose Tools | Templates * and open the template in the editor. */ package coursework;
import java.awt.BorderLayout; import java.awt.Color; import java.awt.Font; import java.awt.PopupMenu; import java.awt.event.ActionEvent; import java.awt.event.ActionListener; import java.awt.event.KeyEvent; import java.awt.event.KeyListener; import java.io.File; import java.text.SimpleDateFormat; import java.util.ArrayList; import java.util.Calendar; import javax.swing.BorderFactory; import javax.swing.BoxLayout; import javax.swing.Icon; import javax.swing.ImageIcon; import javax.swing.JButton; import javax.swing.JComboBox; import javax.swing.JFrame; import javax.swing.JLabel; import javax.swing.JMenu; import javax.swing.JMenuBar; import javax.swing.JMenuItem; import javax.swing.JOptionPane; import javax.swing.JPanel; import javax.swing.JScrollPane; import javax.swing.JSeparator; import javax.swing.JTextArea; import javax.swing.JToolBar; import javax.swing.ScrollPaneConstants;
/** * @author Gepon */ public class Coursework extends JFrame implements ActionListener, KeyListener { JPanel pnl = new JPanel (new BorderLayout()); JTextArea txtNewNote = new JTextArea(30,20); JTextArea txtDisplayNotes = new JTextArea(); ArrayList
@Override public void actionPerformed(ActionEvent ae) { if ("NewNote".equals(ae.getActionCommand())) { addNote(txtNewNote.getText()); txtNewNote.setText(""); } if ("close".equals(ae.getActionCommand())) { txtNewNote.setText(""); } if ("Exit".equals(ae.getActionCommand())) { System.exit(0); } if ("Course".equals(ae.getActionCommand())) { crse = courseList.getSelectedItem().toString (); System.out.println(crse); } }
@Override public void keyTyped(KeyEvent e) { System.out.println("keyTyped Not coded yet."); //To change body of generated methods, choose Tools | Templates. }
@Override public void keyPressed(KeyEvent e) { System.out.println("keyPressed Not coded yet."); //To change body of generated methods, choose Tools | Templates. }
@Override public void keyReleased(KeyEvent e) { System.out.println("keyReleased Not coded yet."); //To change body of generated methods, choose Tools | Templates. }
/** * */ public void model() { course.add("COMP1752"); course.add("COMP1753"); crse = course.get(0); note.add("Arrays are of fixed length and are inflexible."); note.add("ArrayList can be added to and items can be deleted."); }
private void view() { Font fnt = new Font("Georgia", Font.PLAIN, 18); setExtendedState(JFrame.MAXIMIZED_BOTH); setTitle("Coursework - Gepon"); setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); // new MenuBar created JMenuBar menuBar = new JMenuBar(); menuBar.setForeground(Color.BLUE); // image and note objects created in MenuBar ImageIcon menuImage = new ImageIcon("icons/menu.png"); JMenu note = new JMenu(); note = new JMenu("Note"); note.setToolTipText("Note tasks"); note.setIcon(menuImage); note.setFont(fnt); note.add(makeMenuItem("New", "NewNote", "Create a new note. ", fnt)); note.addSeparator(); note.add(makeMenuItem("Close", "Close", "Clear the current note. ", fnt)); menuBar.add(note); //image and course objects, items , created and added in menubar ImageIcon courseImage = new ImageIcon("icons/course1.png"); // created Image course // this will add each course to the combobox for ( String crse : course) { courseList.addItem(crse); } courseList.setFont(fnt); courseList.setMaximumSize(courseList.getPreferredSize()); courseList.addActionListener(this); courseList.setActionCommand("Course"); //courseList.setIcon(courseImage); menuBar.add(courseList); // exit button created and added to the menubar ImageIcon exitImage; exitImage = new ImageIcon("icons/exit.png"); JButton exitButton = new JButton("Exit", exitImage); // Exit Button created // and Image added to the BUTTON exitButton.setActionCommand("Exit"); exitButton.addActionListener(this); menuBar.add(exitButton); // menuBar added to this Frame directly with THIS command this.setJMenuBar(menuBar); //========================================================================= JToolBar toolBar = new JToolBar(); // Setting up the ButtonBar JButton button = null; button = makeButton("Create", "NewNote", "Create a new note.", "New"); toolBar.add(button); button = makeButton("closed door", "Close", "Close this note.", "Close"); toolBar.add(button); toolBar.addSeparator(); button = makeButton("exit", "Exit", "Exit from this program.", "Exit"); toolBar.add(button); add(toolBar, BorderLayout.NORTH); //========================================================================= JPanel pnlWest = new JPanel(); pnlWest.setLayout(new BoxLayout(pnlWest, BoxLayout.Y_AXIS)); pnlWest.setBorder(BorderFactory.createLineBorder(Color.BLACK)); txtNewNote.setFont(fnt); pnlWest.add(txtNewNote); JButton btnAddNote = new JButton ("Add note"); btnAddNote.setActionCommand("NewNote"); btnAddNote.addActionListener(this); pnlWest.add(btnAddNote); add(pnlWest, BorderLayout.WEST); //============================================================================ JPanel cen = new JPanel(); cen.setLayout(new BoxLayout (cen, BoxLayout.Y_AXIS)); cen.setBorder(BorderFactory.createLineBorder(Color.black)); txtDisplayNotes.setFont(fnt); cen.add(txtDisplayNotes); scroller.setVerticalScrollBarPolicy(ScrollPaneConstants.VERTICAL_SCROLLBAR_ALWAYS); scroller.setHorizontalScrollBarPolicy(ScrollPaneConstants.HORIZONTAL_SCROLLBAR_NEVER); scroller.setBounds(3,3,400,400); txtDisplayNotes.setLineWrap(true); cen.add(scroller); add(cen, BorderLayout.CENTER); setVisible(true); }
private void controller() { addAllNotes(); addNewNotes(); }
protected JMenuItem makeMenuItem(String txt, String actionCommand, String toolTipText, Font fnt ) { JMenuItem mnuItem = new JMenuItem (); mnuItem.setText(txt); mnuItem.setActionCommand(actionCommand); mnuItem.setToolTipText(toolTipText); mnuItem.setFont(fnt); mnuItem.addActionListener(this); return mnuItem; }
private JButton makeButton(String imageName, String actionCommand, String toolTipText, String altText) { // create and initialize the button. JButton button = new JButton(); button.setToolTipText (toolTipText); button.setActionCommand(actionCommand); button.addActionListener(this); // look for the image. String imgLocation = System.getProperty("user.dir") + "\\icons\\" + imageName + ".png"; File fyle = new File(imgLocation); if (fyle.exists() && !fyle.isDirectory()) { // image found Icon img; img = new ImageIcon(imgLocation); button.setIcon(img); }else { // image not found button.setText(altText); System.err.println("Resource not found: " + imgLocation); } return button; } private void addNote ( String text) { note.add(txtNewNote.getText()); addAllNotes(); } private void addAllNotes() { String allNotes = " "; for (String n: note ) { allNotes += n + " "; } txtDisplayNotes.setText(allNotes); }
private void addNewNotes() { System.out.println("addNewNotes Not coded yet."); //To change body of generated methods, choose Tools | Templates. }
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
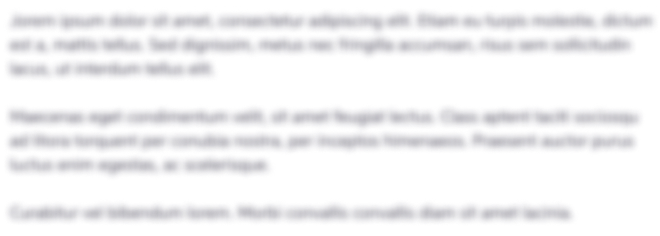
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started