Question
//I am having this error , can you guys help me to fix it,.. please,,,,,,,,,,,,.........................// //Severity Code Description Project File Line Suppression State Error C2664
//I am having this error , can you guys help me to fix it,.. please,,,,,,,,,,,,.........................//
//Severity Code Description Project File Line Suppression State
Error C2664 'void bSearchTreeType
//---------------------------------Ch19_Ex9.cpp-----------------------------//
//68 43 10 56 77 82 61 82 33 56 72 66 99 88 12 6 7 21 -999
#include
#include "binarySearchTree.h"
#include "orderedLinkedList.h"
using namespace std;
int main()
{
bSearchTreeType
orderedLinkedList
int num;
cout << "Enter numbers ending with -999" << endl;
cin >> num;
while (num != -999)
{
treeRoot.insert(num);
cin >> num;
}
cout << endl << "Tree nodes in inorder: ";
treeRoot.inorderTraversal();
cout << endl;
cout << "Tree Height: " << treeRoot.treeHeight()<< endl;
treeRoot.createList(newList);
cout << "newList: ";
newList.print();
cout << endl;
system("pause");
return 0;
}
//--------------------------------------linkedList.h......................................///
#ifndef H_LinkedListType
#define H_LinkedListType
#include
#include
using namespace std;
//Definition of the node
template
class linkedListIterator
{
public:
linkedListIterator();
//Default constructor
//Postcondition: current = nullptr;
linkedListIterator(const nodeType
//Constructor with a parameter.
//Postcondition: current = ptr;
Type operator*();
//Function to overload the dereferencing operator *.
//Postcondition: Returns the info contained in the node.
linkedListIterator
//Overload the pre-increment operator.
//Postcondition: The iterator is advanced to the next
// node.
bool operator==(const linkedListIterator
//Overload the equality operator.
//Postcondition: Returns true if this iterator is equal to
// the iterator specified by right,
// otherwise it returns the value false.
bool operator!=(const linkedListIterator
//Overload the not equal to operator.
//Postcondition: Returns true if this iterator is not
// equal to the iterator specified by
// right; otherwise it returns the value
// false.
private:
nodeType
//node in the linked list
};
template
linkedListIterator
{
current = nullptr;
}
template
linkedListIterator
linkedListIterator(const nodeType
{
current = ptr;
}
template
Type linkedListIterator
{
return current->info;
}
template
linkedListIterator
{
current = current->link;
return *this;
}
template
bool linkedListIterator
(const linkedListIterator
{
return (current == right.current);
}
template
bool linkedListIterator
(const linkedListIterator
{
return (current != right.current);
}
//***************** class linkedListType ****************
template
class linkedListType
{
public:
const linkedListType
(const linkedListType
//Overload the assignment operator.
void initializeList();
//Initialize the list to an empty state.
//Postcondition: first = nullptr, last = nullptr, count = 0;
bool isEmptyList() const;
//Function to determine whether the list is empty.
//Postcondition: Returns true if the list is empty,
// otherwise it returns false.
void print() const;
//Function to output the data contained in each node.
//Postcondition: none
int length() const;
//Function to return the number of nodes in the list.
//Postcondition: The value of count is returned.
void destroyList();
//Function to delete all the nodes from the list.
//Postcondition: first = nullptr, last = nullptr, count = 0;
Type front() const;
//Function to return the first element of the list.
//Precondition: The list must exist and must not be
// empty.
//Postcondition: If the list is empty, the program
// terminates; otherwise, the first
// element of the list is returned.
Type back() const;
//Function to return the last element of the list.
//Precondition: The list must exist and must not be
// empty.
//Postcondition: If the list is empty, the program
// terminates; otherwise, the last
// element of the list is returned.
virtual bool search(const Type& searchItem) const = 0;
//Function to determine whether searchItem is in the list.
//Postcondition: Returns true if searchItem is in the
// list, otherwise the value false is
// returned.
virtual void insertFirst(const Type& newItem) = 0;
//Function to insert newItem at the beginning of the list.
//Postcondition: first points to the new list, newItem is
// inserted at the beginning of the list,
// last points to the last node in the list,
// and count is incremented by 1.
virtual void insertLast(const Type& newItem) = 0;
//Function to insert newItem at the end of the list.
//Postcondition: first points to the new list, newItem
// is inserted at the end of the list,
// last points to the last node in the list,
// and count is incremented by 1.
virtual void deleteNode(const Type& deleteItem) = 0;
//Function to delete deleteItem from the list.
//Postcondition: If found, the node containing
// deleteItem is deleted from the list.
// first points to the first node, last
// points to the last node of the updated
// list, and count is decremented by 1.
linkedListIterator
//Function to return an iterator at the begining of the
//linked list.
//Postcondition: Returns an iterator such that current is
// set to first.
linkedListIterator
//Function to return an iterator one element past the
//last element of the linked list.
//Postcondition: Returns an iterator such that current is
// set to nullptr.
linkedListType();
//default constructor
//Initializes the list to an empty state.
//Postcondition: first = nullptr, last = nullptr, count = 0;
linkedListType(const linkedListType
//copy constructor
~linkedListType();
//destructor
//Deletes all the nodes from the list.
//Postcondition: The list object is destroyed.
protected:
int count; //variable to store the number of
//elements in the list
nodeType
nodeType
private:
void copyList(const linkedListType
//Function to make a copy of otherList.
//Postcondition: A copy of otherList is created and
// assigned to this list.
};
template
bool linkedListType
{
return(first == nullptr);
}
template
linkedListType
{
first = nullptr;
last = nullptr;
count = 0;
}
template
void linkedListType
{
nodeType
//occupied by the node
while (first != nullptr) //while there are nodes in the list
{
temp = first; //set temp to the current node
first = first->link; //advance first to the next node
delete temp; //deallocate the memory occupied by temp
}
last = nullptr; //initialize last to nullptr; first has already
//been set to nullptr by the while loop
count = 0;
}
template
void linkedListType
{
destroyList(); //if the list has any nodes, delete them
}
template
void linkedListType
{
nodeType
current = first; //set current so that it points to
//the first node
while (current != nullptr) //while more data to print
{
cout << current->info << " ";
current = current->link;
}
}//end print
template
int linkedListType
{
return count;
} //end length
template
Type linkedListType
{
assert(first != nullptr);
return first->info; //return the info of the first node
}//end front
template
Type linkedListType
{
assert(last != nullptr);
return last->info; //return the info of the last node
}//end back
template
linkedListIterator
{
linkedListIterator
return temp;
}
template
linkedListIterator
{
linkedListIterator
return temp;
}
template
void linkedListType
(const linkedListType
{
nodeType
nodeType
if (first != nullptr) //if the list is nonempty, make it empty
destroyList();
if (otherList.first == nullptr) //otherList is empty
{
first = nullptr;
last = nullptr;
count = 0;
}
else
{
current = otherList.first; //current points to the
//list to be copied
count = otherList.count;
//copy the first node
first = new nodeType
first->info = current->info; //copy the info
first->link = nullptr; //set the link field of
//the node to nullptr
last = first; //make last point to the
//first node
current = current->link; //make current point to
//the next node
//copy the remaining list
while (current != nullptr)
{
newNode = new nodeType
newNode->info = current->info; //copy the info
newNode->link = nullptr; //set the link of
//newNode to nullptr
last->link = newNode; //attach newNode after last
last = newNode; //make last point to
//the actual last node
current = current->link; //make current point
//to the next node
}//end while
}//end else
}//end copyList
template
linkedListType
{
destroyList();
}//end destructor
template
linkedListType
(const linkedListType
{
first = nullptr;
copyList(otherList);
}//end copy constructor
//overload the assignment operator
template
const linkedListType
(const linkedListType
{
if (this != &otherList) //avoid self-copy
{
copyList(otherList);
}//end else
return *this;
}
#endif
//-----------------------------------------orderedlinklist.h-----------------------------------//
#ifndef H_orderedListType
#define H_orderedListType
#include "linkedList.h"
using namespace std;
template
class orderedLinkedList : public linkedListType
{
public:
bool search(const Type& searchItem) const;
//Function to determine whether searchItem is in the list.
//Postcondition: Returns true if searchItem is in the list,
// otherwise the value false is returned.
void insert(const Type& newItem);
//Function to insert newItem in the list.
//Postcondition: first points to the new list, newItem
// is inserted at the proper place in the
// list, and count is incremented by 1.
void insertFirst(const Type& newItem);
//Function to insert newItem in the list.
//Because the resulting list must be sorted, newItem is
//inserted at the proper in the list.
//This function uses the function insert to insert newItem.
//Postcondition: first points to the new list, newItem is
// inserted at the proper in the list,
// and count is incremented by 1.
void insertLast(const Type& newItem);
//Function to insert newItem in the list.
//Because the resulting list must be sorted, newItem is
//inserted at the proper in the list.
//This function uses the function insert to insert newItem.
//Postcondition: first points to the new list, newItem is
// inserted at the proper in the list,
// and count is incremented by 1.
void deleteNode(const Type& deleteItem);
//Function to delete deleteItem from the list.
//Postcondition: If found, the node containing
// deleteItem is deleted from the list;
// first points to the first node of the
// new list, and count is decremented by 1.
// If deleteItem is not in the list, an
// appropriate message is printed.
};
template
bool orderedLinkedList
{
bool found = false;
nodeType
current = first; //start the search at the first node
while (current != nullptr && !found)
if (current->info >= searchItem)
found = true;
else
current = current->link;
if (found)
found = (current->info == searchItem); //test for equality
return found;
}//end search
template
void orderedLinkedList
{
nodeType
nodeType
nodeType
bool found;
newNode = new nodeType
newNode->info = newItem; //store newItem in the node
newNode->link = nullptr; //set the link field of the node
//to nullptr
if (first == nullptr) //Case 1
{
first = newNode;
last = newNode;
count++;
}
else
{
current = first;
found = false;
while (current != nullptr && !found) //search the list
if (current->info >= newItem)
found = true;
else
{
trailCurrent = current;
current = current->link;
}
if (current == first) //Case 2
{
newNode->link = first;
first = newNode;
count++;
}
else //Case 3
{
trailCurrent->link = newNode;
newNode->link = current;
if (current == nullptr)
last = newNode;
count++;
}
}//end else
}//end insert
template
void orderedLinkedList
{
insert(newItem);
}//end insertFirst
template
void orderedLinkedList
{
insert(newItem);
}//end insertLast
template
void orderedLinkedList
{
nodeType
nodeType
bool found;
if (first == nullptr) //Case 1
cout << "Cannot delete from an empty list." << endl;
else
{
current = first;
found = false;
while (current != nullptr && !found) //search the list
if (current->info >= deleteItem)
found = true;
else
{
trailCurrent = current;
current = current->link;
}
if (current == nullptr) //Case 4
cout << "The item to be deleted is not in the "
<< "list." << endl;
else
if (current->info == deleteItem) //the item to be
//deleted is in the list
{
if (first == current) //Case 2
{
first = first->link;
if (first == nullptr)
last = nullptr;
delete current;
}
else //Case 3
{
trailCurrent->link = current->link;
if (current == last)
last = trailCurrent;
delete current;
}
count--;
}
else //Case 4
cout << "The item to be deleted is not in the "
<< "list." << endl;
}
}//end deleteNode
#endif
//---------------------------------------binaryTree.h--------------------------------------------//
//Header File Binary Search Tree
#ifndef H_binaryTree
#define H_binaryTree
#include
using namespace std;
//Definition of the Node
template
struct nodeType
{
elemType info;
nodeType
nodeType
};
//Definition of the class
template
class binaryTreeType
{
public:
const binaryTreeType
(const binaryTreeType
//Overload the assignment operator.
bool isEmpty() const;
//Function to determine whether the binary tree is empty.
//Postcondition: Returns true if the binary tree is empty;
// otherwise, returns false.
void inorderTraversal() const;
//Function to do an inorder traversal of the binary tree.
//Postcondition: Nodes are printed in inorder sequence.
void preorderTraversal() const;
//Function to do a preorder traversal of the binary tree.
//Postcondition: Nodes are printed in preorder sequence.
void postorderTraversal() const;
//Function to do a postorder traversal of the binary tree.
//Postcondition: Nodes are printed in postorder sequence.
int treeHeight() const;
//Function to determine the height of a binary tree.
//Postcondition: Returns the height of the binary tree.
int treeNodeCount() const;
//Function to determine the number of nodes in a
//binary tree.
//Postcondition: Returns the number of nodes in the
// binary tree.
int treeLeavesCount() const;
//Function to determine the number of leaves in a
//binary tree.
//Postcondition: Returns the number of leaves in the
// binary tree.
void destroyTree();
//Function to destroy the binary tree.
//Postcondition: Memory space occupied by each node
// is deallocated.
// root = nullptr;
virtual bool search(const elemType& searchItem) const = 0;
//Function to determine if searchItem is in the binary
//tree.
//Postcondition: Returns true if searchItem is found in
// the binary tree; otherwise, returns
// false.
virtual void insert(const elemType& insertItem) = 0;
//Function to insert insertItem in the binary tree.
//Postcondition: If there is no node in the binary tree
// that has the same info as insertItem, a
// node with the info insertItem is created
// and inserted in the binary search tree.
virtual void deleteNode(const elemType& deleteItem) = 0;
//Function to delete deleteItem from the binary tree
//Postcondition: If a node with the same info as
// deleteItem is found, it is deleted from
// the binary tree.
// If the binary tree is empty or
// deleteItem is not in the binary tree,
// an appropriate message is printed.
virtual void createList(elemType& newList) = 0;
binaryTreeType(const binaryTreeType
//Copy constructor
binaryTreeType();
//Default constructor
~binaryTreeType();
//Destructor
protected:
nodeType
private:
void copyTree(nodeType
nodeType
//Makes a copy of the binary tree to which
//otherTreeRoot points.
//Postcondition: The pointer copiedTreeRoot points to
// the root of the copied binary tree.
void destroy(nodeType
//Function to destroy the binary tree to which p points.
//Postcondition: Memory space occupied by each node, in
// the binary tree to which p points, is
// deallocated.
// p = nullptr;
void inorder(nodeType
//Function to do an inorder traversal of the binary
//tree to which p points.
//Postcondition: Nodes of the binary tree, to which p
// points, are printed in inorder sequence.
void preorder(nodeType
//Function to do a preorder traversal of the binary
//tree to which p points.
//Postcondition: Nodes of the binary tree, to which p
// points, are printed in preorder
// sequence.
void postorder(nodeType
//Function to do a postorder traversal of the binary
//tree to which p points.
//Postcondition: Nodes of the binary tree, to which p
// points, are printed in postorder
// sequence.
int height(nodeType
//Function to determine the height of the binary tree
//to which p points.
//Postcondition: Height of the binary tree to which
// p points is returned.
int max(int x, int y) const;
//Function to determine the larger of x and y.
//Postcondition: Returns the larger of x and y.
int nodeCount(nodeType
//Function to determine the number of nodes in
//the binary tree to which p points.
//Postcondition: The number of nodes in the binary
// tree to which p points is returned.
int leavesCount(nodeType
//Function to determine the number of leaves in
//the binary tree to which p points
//Postcondition: The number of leaves in the binary
// tree to which p points is returned.
};
//Definition of member functions
template
binaryTreeType
{
root = nullptr;
}
template
bool binaryTreeType
{
return (root == nullptr);
}
template
void binaryTreeType
{
inorder(root);
}
template
void binaryTreeType
{
preorder(root);
}
template
void binaryTreeType
{
postorder(root);
}
template
int binaryTreeType
{
return height(root);
}
template
int binaryTreeType
{
return nodeCount(root);
}
template
int binaryTreeType
{
return leavesCount(root);
}
template
void binaryTreeType
(nodeType
nodeType
{
if (otherTreeRoot == nullptr)
copiedTreeRoot = nullptr;
else
{
copiedTreeRoot = new nodeType
copiedTreeRoot->info = otherTreeRoot->info;
copyTree(copiedTreeRoot->lLink, otherTreeRoot->lLink);
copyTree(copiedTreeRoot->rLink, otherTreeRoot->rLink);
}
} //end copyTree
template
void binaryTreeType
(nodeType
{
if (p != nullptr)
{
inorder(p->lLink);
cout << p->info << " ";
inorder(p->rLink);
}
}
template
void binaryTreeType
(nodeType
{
if (p != nullptr)
{
cout << p->info << " ";
preorder(p->lLink);
preorder(p->rLink);
}
}
template
void binaryTreeType
(nodeType
{
if (p != nullptr)
{
postorder(p->lLink);
postorder(p->rLink);
cout << p->info << " ";
}
}
//Overload the assignment operator
template
const binaryTreeType
operator=(const binaryTreeType
{
if (this != &otherTree) //avoid self-copy
{
if (root != nullptr) //if the binary tree is not empty,
//destroy the binary tree
destroy(root);
if (otherTree.root == nullptr) //otherTree is empty
root = nullptr;
else
copyTree(root, otherTree.root);
}//end else
return *this;
}
template
void binaryTreeType
{
if (p != nullptr)
{
destroy(p->lLink);
destroy(p->rLink);
delete p;
p = nullptr;
}
}
template
void binaryTreeType
{
destroy(root);
}
//copy constructor
template
binaryTreeType
(const binaryTreeType
{
if (otherTree.root == nullptr) //otherTree is empty
root = nullptr;
else
copyTree(root, otherTree.root);
}
//Destructor
template
binaryTreeType
{
destroy(root);
}
template
int binaryTreeType
(nodeType
{
if (p == nullptr)
return 0;
else
return 1 + max(height(p->lLink), height(p->rLink));
}
template
int binaryTreeType
{
if (x >= y)
return x;
else
return y;
}
template
int binaryTreeType
{
cout << "Write the definition of the function nodeCount."
<< endl;
return 0;
}
template
int binaryTreeType
{
cout << "Write the definition of the function leavesCount."
<< endl;
return 0;
}
#endif
//---------------------------------------------------binarysreachtree.h------------------------------//
//Header File Binary Search Tree
#ifndef H_binarySearchTree
#define H_binarySearchTree
#include
#include "binaryTree.h"
using namespace std;
template
class bSearchTreeType : public binaryTreeType
{
public:
bool search(const elemType& searchItem) const;
//Function to determine if searchItem is in the binary
//search tree.
//Postcondition: Returns true if searchItem is found in
// the binary search tree; otherwise,
// returns false.
void insert(const elemType& insertItem);
//Function to insert insertItem in the binary search tree.
//Postcondition: If there is no node in the binary search
// tree that has the same info as
// insertItem, a node with the info
// insertItem is created and inserted in the
// binary search tree.
void deleteNode(const elemType& deleteItem);
//Function to delete deleteItem from the binary search tree
//Postcondition: If a node with the same info as deleteItem
// is found, it is deleted from the binary
// search tree.
// If the binary tree is empty or deleteItem
// is not in the binary tree, an appropriate
// message is printed.
void createList(elemType& addItem);
private:
void deleteFromTree(nodeType
//Function to delete the node to which p points is
//deleted from the binary search tree.
//Postcondition: The node to which p points is deleted
// from the binary search tree.
};
template
bool bSearchTreeType
(const elemType& searchItem) const
{
nodeType
bool found = false;
if (root == nullptrptr)
cout << "Cannot search an empty tree." << endl;
else
{
current = root;
while (current != nullptrptr && !found)
{
if (current->info == searchItem)
found = true;
else if (current->info > searchItem)
current = current->lLink;
else
current = current->rLink;
}//end while
}//end else
return found;
}//end search
template
void bSearchTreeType
(const elemType& insertItem)
{
nodeType
nodeType
nodeType
newNode = new nodeType
newNode->info = insertItem;
newNode->lLink = nullptrptr;
newNode->rLink = nullptrptr;
if (root == nullptrptr)
root = newNode;
else
{
current = root;
while (current != nullptrptr)
{
trailCurrent = current;
if (current->info == insertItem)
{
cout << "The item to be inserted is already ";
cout << "in the tree -- duplicates are not "
<< "allowed." << endl;
return;
}
else if (current->info > insertItem)
current = current->lLink;
else
current = current->rLink;
}//end while
if (trailCurrent->info > insertItem)
trailCurrent->lLink = newNode;
else
trailCurrent->rLink = newNode;
}
}//end insert
template
void bSearchTreeType
(const elemType& deleteItem)
{
nodeType
nodeType
bool found = false;
if (root == nullptr)
cout << "Cannot delete from an empty tree."
<< endl;
else
{
current = root;
trailCurrent = root;
while (current != nullptr && !found)
{
if (current->info == deleteItem)
found = true;
else
{
trailCurrent = current;
if (current->info > deleteItem)
current = current->lLink;
else
current = current->rLink;
}
}//end while
if (current == nullptr)
cout << "The item to be deleted is not in the tree."
<< endl;
else if (found)
{
if (current == root)
deleteFromTree(root);
else if (trailCurrent->info > deleteItem)
deleteFromTree(trailCurrent->lLink);
else
deleteFromTree(trailCurrent->rLink);
}
else
cout << "The item to be deleted is not in the tree."
<< endl;
}
} //end deleteNode
template
void bSearchTreeType
(nodeType
{
nodeType
nodeType
nodeType
if (p == nullptr)
cout << "Error: The node to be deleted does not exist."
<< endl;
else if (p->lLink == nullptr && p->rLink == nullptr)
{
temp = p;
p = nullptr;
delete temp;
}
else if (p->lLink == nullptr)
{
temp = p;
p = temp->rLink;
delete temp;
}
else if (p->rLink == nullptr)
{
temp = p;
p = temp->lLink;
delete temp;
}
else
{
current = p->lLink;
trailCurrent = nullptr;
while (current->rLink != nullptr)
{
trailCurrent = current;
current = current->rLink;
}//end while
p->info = current->info;
if (trailCurrent == nullptr) //current did not move;
//current == p->lLink; adjust p
p->lLink = current->lLink;
else
trailCurrent->rLink = current->lLink;
delete current;
}//end else
} //end deleteFromTree
template
void bSearchTreeType
{
nodeType
nodeType
nodeType
newNode = new nodeType
newNode->info = createItem;
newNode->lLink = nullptr;
newNode->rLink = nullptr;
if (root == nullptr)
{
root = newNode;
}
}
#endif
Step by Step Solution
There are 3 Steps involved in it
Step: 1
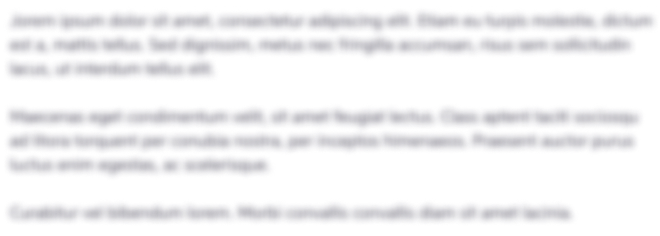
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started