Question
I am having trouble getting my code to run for week 2 of my Advanced C++ course. Here are the instructions for week 2. Use
I am having trouble getting my code to run for week 2 of my Advanced C++ course.
Here are the instructions for week 2.
Use from scratch or modify week 1 bankAccount class.The class should define and implement the following data and methods:
bankAccount
Data
accountNumber
balance
Methods
setAccountNumber
getAccountNumber
getBalance
withdraw
deposit
Constructor:
sets account number
sets account balance
Derive class checkingAccount from base class bankAccount.The checkingAccount class should define and implement the following data and methods:
checkingAccount
Data
interestRate
minimumBalance
serviceCharge
Methods
getMinimumBalance
setMinimumBalance
getInterestRate
setInterestRate
getServiceCharge
setServiceCharge
postInterest
writeCheck
withdraw
Constructor:
sets account number
sets account balance
sets minimum balance
set interest rate
sets service charge amount
NOTES:
postInterest takes the interest amount based off of the total balance and adds it to the balance (balance + balance * interestRate)
writeCheck calls withdraw
withdraw checks to make sure there is enough money in the account first and reports a warning if not.If there is enough money to make the withdraw, it then checks to see if the balance will go below the minimum amount after the withdraw.If so, it checks to see if the balance will go below zero after the withdraw and the service charge is applied.Should this occur an error is reported.If not, the balance is adjusted to reflect the withdraw and the service charge applied.
Derive class savingsAccount from base class bankAccount.The savingsAccount class should define and implement the following data and methods:
savingsAccount
Data
interestRate
Methods
getInterestRate
setInterestRate
withdraw
postInterest
Constructor:
sets account number
sets account balance
set interest rate
NOTES:
Withdraw checks to make sure there is enough money before altering the balance.If not, a warning message is printed and the balance remains unchanged.
Create a main program to test the class as follows. Use hard coded data (do not prompt the user for data, just make up values).Perform these steps in order:
1.create 2 checking accounts and 2 savings accounts
2.deposit money in each
3.post interest in each
4.print details of each
5.write checks in the checking accounts
6.withdraw from the savings accounts
7.print details of each
Here is the code I have so far:
bankAccount.h
#ifndef BANKACCOUNT_H
#define BANKACCOUNT_H
#include
#include
using namespace std;
class bankAccount
{
protected:
string accountNumber;
double balance;
public:
bankAccount();
bankAccount(string acctNum, double startBal);
~bankAccount();
double deposit(double depositAmt);
string getAccountNumber();
double getBalance();
void print();
void setAccountNumber(string acctNum);
double withdraw(double withdrawalAmt);
};
#endif // !BANKACCOUNT_H
checkingAccount.h
#ifndef CHECKINGACCOUNT_H
#define CHECKINGACCOUNT_H
#include "bankAccount.h"
#include
#include
using namespace std;
class checkingAccount :
public bankAccount
{
private:
double interestRate;
double minimumBalance;
double serviceCharge;
public:
checkingAccount();
checkingAccount(string acctNum, double startBal, double intRate, double minBalance, double srvCharge);
~checkingAccount();
double getInterestRate();
double getMinimumBalance();
double getServiceCharge();
double postInterest();
void print();
void setInterestRate(double intRate);
void setMinimumBalance(double minBalance);
void setServiceCharge(double srvCharge);
bool verifyMinimumBalance();
double withdraw(double withdrawalAmt);
void writeCheck(string recipient, double checkAmount);
};
#endif
savingsAccount.cpp
#include "savingsAccount.h"
savingsAccount::savingsAccount()
{
}
savingsAccount::savingsAccount(string acctNum, double startBal, double intRate)
{
accountNumber = acctNum;
balance = startBal;
interestRate = intRate;
}
savingsAccount::~savingsAccount()
{
}
double savingsAccount::getInterestRate()
{
return interestRate;
}
double savingsAccount::postInterest()
{
double interest = 0;
interest = bankAccount::balance * (interestRate / 100);
bankAccount::balance += interest;
return interest;
}
void savingsAccount::print()
{
cout << endl << "Your account Number:" << getAccountNumber() << endl;
cout << "Your current balance: $" << getBalance() << endl;
cout << "Your interest rate:%" << getInterestRate() << endl;
}
void savingsAccount::setInterestRate(double intRate)
{
interestRate = intRate;
}
double savingsAccount::withdraw(double withdrawalAmt)
{
//verify there's enough money in account
if ((bankAccount::balance - withdrawalAmt) < 0)
{
cout << endl << "Insufficient funds for withdrawal." << endl;
}
else
{
bankAccount::balance -= withdrawalAmt;
}
return bankAccount::balance;
}
savingsAccount.h
#ifndef SAVINGSACCOUNT_H
#define SAVINGSACCOUNT_H
#include
#include
#include "bankAccount.h"
using namespace std;
class savingsAccount :
public bankAccount
{
private:
double interestRate;
public:
savingsAccount();
savingsAccount(string acctNum, double startBal, double intRate);
~savingsAccount();
double getInterestRate();
double postInterest();
void print();
void setInterestRate(double intRate);
double withdraw(double withdrawalAmt);
};
#endif
checkingAccount.cpp
#include "checkingAccount.h"
checkingAccount::checkingAccount()
{
}
checkingAccount::checkingAccount(string acctNum, double startBal, double intRate, double minBalance, double srvCharge)
{
bankAccount::accountNumber = acctNum;
bankAccount::balance = startBal;
interestRate = intRate;
minimumBalance = minBalance;
serviceCharge = srvCharge;
}
checkingAccount::~checkingAccount()
{
}
double checkingAccount::getInterestRate()
{
return interestRate;
}
double checkingAccount::getMinimumBalance()
{
return minimumBalance;
}
double checkingAccount::getServiceCharge()
{
return serviceCharge;
}
// Multiplies balance times interest rate (as percentage) divided by 100, adds interest to balance,
// and returns interest gained
double checkingAccount::postInterest()
{
if (balance <= 0)
{
return 0;
}
double interest = 0;
interest = bankAccount::balance * (interestRate / 100);
bankAccount::balance += interest;
return interest;
}
void checkingAccount::print()
{
cout << endl << "Your account Number:" << getAccountNumber() << endl;
cout << "Your current balance: $" << getBalance() << endl;
cout << "Your interest rate:%" << getInterestRate() << endl;
cout << "Your minimum balance: $" << getMinimumBalance() << endl;
cout << "Your service charge:$" << getServiceCharge() << endl;
}
void checkingAccount::setInterestRate(double intRate)
{
interestRate = intRate;
}
void checkingAccount::setMinimumBalance(double minBalance)
{
minimumBalance = minBalance;
}
void checkingAccount::setServiceCharge(double srvCharge)
{
serviceCharge = srvCharge;
}
// returns true if balance is below minimum balance
bool checkingAccount::verifyMinimumBalance()
{
bool balanceBelow = false;
if (bankAccount::balance < minimumBalance)
balanceBelow = true;
return balanceBelow;
}
double checkingAccount::withdraw(double withdrawalAmt)
{
//check if enough balance
if ((bankAccount::balance - withdrawalAmt) < 0)
{
cout << endl << "This will overdraw your account! Can not withdraw." << endl;
}
else
{
bankAccount::balance -= withdrawalAmt;
if (verifyMinimumBalance())
{
balance -= getServiceCharge();
cout << "You've dropped below your minimum balacnce!" << endl;
cout << "You've been charged a fee of $" << getServiceCharge() << endl;
}
}
return bankAccount::balance;
}
void checkingAccount::writeCheck(string recipient, double checkAmount)
{
if ((balance - checkAmount) < 0)
{
cout << "Insufficient funds" << endl;
return;
}
withdraw(checkAmount);
//check min balance and charge if below min balance
if (verifyMinimumBalance())
{
balance -= getServiceCharge();
cout << "You've dropped below your minimum balacnce!" << endl;
cout << "You've been charged a fee of $" << getServiceCharge();
}
cout << endl << "Check sent to " << recipient << " for $" << checkAmount << endl;
cout << "Your current balance is $" << getBalance() << endl;
}
bankAccount.cpp
#include "bankAccount.h"
bankAccount::bankAccount()
{
}
bankAccount::bankAccount(string acctNum, double startBal)
{
accountNumber = acctNum;
balance = startBal;
}
bankAccount::~bankAccount()
{
}
double bankAccount::deposit(double depositAmt)
{
balance += depositAmt;
return balance;
}
string bankAccount::getAccountNumber()
{
return accountNumber;
}
double bankAccount::getBalance()
{
return balance;
}
void bankAccount::print()
{
cout << "Your account Number:" << getAccountNumber() << endl;
cout << "Your current balance: $" << getBalance() << endl;
}
void bankAccount::setAccountNumber(string acctNum)
{
accountNumber = acctNum;
}
double bankAccount::withdraw(double withdrawalAmt)
{
balance -= withdrawalAmt;
return balance;
}
main.cpp
//main.cpp
#include
#include "bankAccount.h"
using namespace std;
// Initialize static member "nextaccountnumber" of class bankAccount
int bankAccount::nextaccountnumber = 1000;
//Declare a const
const int CUSTOMER_COUNT = 10;
//main function
int main()
{
//Declaring an array of 10 components of type bankAccount.
bankAccount myaccounts[CUSTOMER_COUNT];
//getting customer information from user
for(int index = 0; index < CUSTOMER_COUNT; index++){
cout << " Enter customer " << index + 1 << " information: " << endl;
//get customer name
string holdername;
cout << "Enter account holder name: ";
getline(cin, holdername);
//Get account type
string accounttype;
do
{
//checcking for valid input
cout << "Enter account type (savings or checkings): ";
getline(cin, accounttype);
if(accounttype == "savings" || accounttype == "checkings"){
break;
}
cout << "Invalid account type! Please try again." << endl;
}while(true);
//get account balance
cout << "Enter initial account balance: ";
double balance;
cin >> balance;
//get interest rate
cout << "Enter interest rate: ";
double interestrate;
cin >> interestrate;
//creating object and adding it to array
myaccounts[index] = bankAccount(holdername, accounttype, balance, interestrate);
cin.ignore();
}
//Iterating the array and printing all the customer information
cout << " Printing all customers information " << endl;
for(int index = 0; index < CUSTOMER_COUNT; index++){
//using print() method to display account information
myaccounts[index].print();
}
return 0;
}
I can't get it to run and I am not sure where I am going wrong. Please assist and explain.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
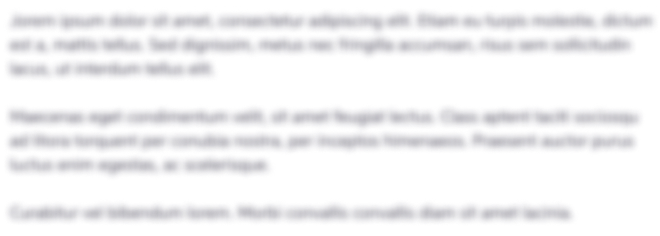
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started