Question
I am having trouble trying to call the methods from the constructors onto the other class. My goal is to be able to get the
I am having trouble trying to call the methods from the constructors onto the other class. My goal is to be able to get the user to create their own type of hollow square For exmaple:
***
* *
* *
***
This is where I am stuck. Wonder if I went about this the wrong way.... I already got these parts in my Java files
1. One instance variable sideLength
2. Two constructs
3. Mutators and Accessors
4. fillSquare()
5. hollowSquare()
These parts I am having trouble putting onto the main method
1. Input validation. User input must be a positive digit from 1 10.
2. Input validation. User input must choose between two options only filled and hollow. You can create a menu 1 for filled and 2 for square. Or you can validate input using string operators.
3. Include user prompts that guide user input even during input validation
4. Use a do while loop. The program continues until the user decides to quit the game
My SquareGame.java class and the main TestGame.java class are below;
import java.util.Scanner;
public class SquareGame {
//static
static Scanner sc = new Scanner (System.in);
private int height;
private int sidelength;
//standard constructor
public SquareGame() {
System.out.print("Enter the height of the rectangle: ");
height = sc.nextInt();
System.out.print("Enter the width of the rectangle: ");
sidelength = sc.nextInt();
}
/** Constructor
*
* @param h The height of the square
* @param w the sidelength of the square
*/
public SquareGame(int h, int w) {
height = h;
sidelength =w;
}
/**
* method returns height accessor
* @return height
*/
public int getHeight() {
return height;
}
/**
* method returns sidelength accessor
* @return sidelength
*/
public int getSideLength() {
return sidelength;
}
/**
* height mutator
* @param h The value to store the height
*/
public void setHeight(int h) {
height = h;
}
/**
* sidelength mutator
* @param w The value to store the length
*/
public void setSideLength(int w) {
sidelength = w;
}
/**
* method that creates a hollow square of *
*/
public void hollowSquare() {
for (int x = 1; x <= height; x++)
{
// Print table row
for (int c = 1; c <= sidelength; c++)
{
if (x == 1 || c == 1 || c == sidelength || x == height) {
System.out.print("*");
}
else
System.out.print(" ");
}
System.out.println();
}
}
/**
* method that creates a filled square of *
*
*/
public void fillSquare() {
for (int x = 1; x <= height; x++) {
// Print table row
for (int c = 1; c <= sidelength; c++) {
System.out.print("*");
}
System.out.println();
}
}
}
----------------------------------------------------------------------------------------------------------------------------------------------
import java.util.Scanner;
import java.util.*;
public class TestGame {
public static void main(String[] args) {
// TODO Auto-generated method stub
Scanner userInput = new Scanner(System.in);
SquareGame.SquareGame();
System.out.println("Enter the height of the rectangle between postive numbers 1-10: ");
int height = userInput.nextInt();
System.out.println("Enter the width of the rectangle between postive numbers 1-10: ");
int sidelength = userInput.nextInt();
SquareGame Square = new SquareGame(mHeight, mSideLength);
System.out.println("Enter between 1-10: ");
int mHeight = userInput.nextInt();
System.out.println("Enter between 1-10: ");
int mSideLength = userInput.nextInt();
Square.fillSquare(mHeight,mSideLength);
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
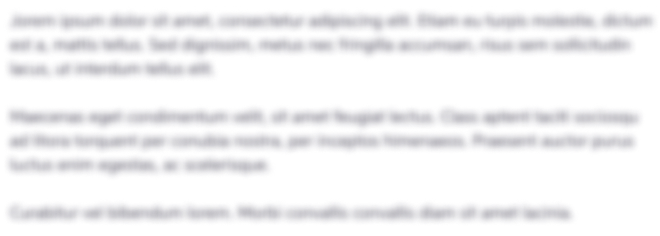
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started