Question
I am having trouble with this problem. It keeps giving me the wrong outputs. Please help! Define a class counterType to implement a counter. Your
I am having trouble with this problem. It keeps giving me the wrong outputs. Please help!
Define a class counterType to implement a counter. Your class must have a private data member counter of type int. Define a constructor that accepts a parameter of type int and initializes the counter data member. Add functions to:
Set counter to the integer value specified by the user
Initialize counter to 0
Return the value of counter with a function named getCounter
Increment and decrement counter by one.
Print the value of counter using the print function
The value of counter must be nonnegative.
My code:
counterType.h
class counterType { private: int counter; public: counterType(); counterType(int counter); void print(); void incrementCounter(); void decrementCounter(); void setCounter(int c); int getCounter(); };
counterTypeImp.cpp
#include
counterType::counterType(int counter) { setCounter(counter); }
counterType::counterType() { counter = 0; }
void counterType::setCounter(int c) { cout << "Enter a positive number: " << endl; cin >> c; if (c > 0) { c = counter; } else { cout << "Please enter a positive number" << endl; } }
int counterType::getCounter() { return counter; }
void counterType::incrementCounter() { counter++; }
void counterType::decrementCounter() { counter--; }
void counterType::print() { cout << counter << endl; }
main.cpp (PROVIDED BY PROFESSOR)
//Main program #include
using namespace std;
int main() { counterType counter1; counterType counter2(5);
counter1.print();
cout << endl;
counter1.incrementCounter(); cout << "After Increment counter1: " << counter1.getCounter() << endl;
cout << "Counter2 = " << counter2.getCounter() << endl;
counter2.decrementCounter(); cout << "After decrement counter2 = " << counter2.getCounter() << endl;
counter1.setCounter(-6); cout << "After resetting counter1: " << counter1.getCounter() << endl;
return 0; }
The results I keep getting:
Enter a positive number: 5 0
After Increment counter1: 1 Counter2 = 4197568 After decrement counter2 = 4197567 Enter a positive number:
Step by Step Solution
There are 3 Steps involved in it
Step: 1
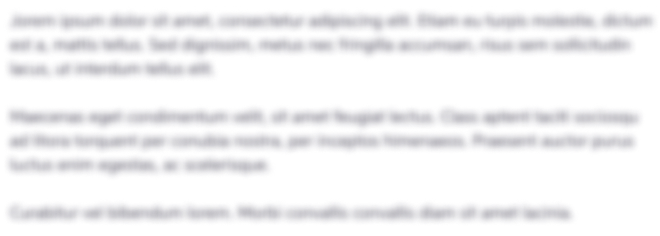
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started