Question
I am making a simon says game using an arduino and I found this code off of the internet that I wanted to use. I
I am making a simon says game using an arduino and I found this code off of the internet that I wanted to use. I have already tweaked it some to add another LED and I am wanting to make the game have an easy mode and a hard mode. The easy mode will happen when any other button besides red is pushed and hard mode will happen when red is pushed. I feel like its really easy to change but i cant seem to figure it out. The delay I wanted for EASY MODE is to be 10 seconds between each button pushed and HARD MODE I wanted it to be around 3-5 seconds.
// Code for Simon Says Arduino Wiring and Code Tutorial // Video found at: https://www.youtube.com/watch?v=TJiz7PT21B4
// Define all the LED and Button pins. // {RED, GREEN, YELLOW, BLUE, WHITE} int buttons[] = {3, 5, 7, 9, 11}; //The four button input pins int leds[] = {2, 4, 6, 8, 10}; // LED pins
//Keeps track of the sequence to repeat int sequence[100];
int largestIndex = 0; //the largest index in the sequence so far
int buttonPin = 2;
const int START = 0; const int PLAYEASY = 1; const int PLAYHARD = 2; const int GAMEOVER = 3;
int gameState;
int speed = 5000; boolean running = true; //
long currentMillis = 0; long previousMillis = 0;
// the setup routine runs once when you press reset: void setup() {
for(int pin=0; pin<5; pin++) { pinMode(leds[pin], OUTPUT); } for(int pin=0; pin<5; pin++) { pinMode(buttons[pin], INPUT); } Serial.begin(9600);
gameState = START; // Start the game with the start. randomSeed(analogRead(40)); // initializes the pseudo-random number generator, }
// the loop routine runs over and over again forever: void loop() { if(gameState == START) { waitToStart(); } else if(gameState == PLAYEASY) { Serial.println("Start"); showSequence(); readSequence(); } else if(gameState == PLAYHARD) { Serial.println("Start Hard Mode"); showSequenceHard(); readSequenceHard(); } else if(gameState == GAMEOVER) { Serial.println("Gameover"); blinkAll(5); gameState = START; } }
void showSequence() {
//blinkRed(2); sequence[largestIndex] = random(0,5); Serial.println("Next out"); Serial.println(sequence[largestIndex]); largestIndex++; for(int index=0; index void readSequence() { //blinkYellow(2); int positionPressed; boolean madeMistake = false; for(int index=0; index Serial.println("Pressed"); Serial.println(positionPressed); // count++; if(positionPressed == -1 | positionPressed != sequence[index]) { madeMistake = true; // Exit the loop. gameState = GAMEOVER; } } //blinkBlue(2); } // Returns the position of Button pressed (0, 1, 2, 3, or 4) or -1 if no button is pressed in the time period. int waitForButton(int delay) { int buttonPressed = -1; int input; boolean buttonBackUp = false; currentMillis = millis(); // The number of ms since the program started running previousMillis = currentMillis; // Records the point when we start spinning the loop. // Keep spinning the loop until "delay" seconds have passed. while (currentMillis - previousMillis < delay & buttonBackUp == false) { // Read the button and record when it has been pushed down. for(int pin = 0; pin < 5 & buttonBackUp == false; pin++) { if(digitalRead(buttons[pin]) == HIGH) { buttonPressed = pin; // Show the LED pushed. digitalWrite(leds[pin], HIGH); // It is possible the button is still being pushed. // This loop spins until the button is let up. while (currentMillis - previousMillis < delay & buttonBackUp == false) { input = digitalRead(buttons[pin]); if(input == LOW) { buttonBackUp = true; } currentMillis = millis(); } // Turn the LED pushed off. digitalWrite(leds[pin], LOW); // See if they took to long. if(currentMillis - previousMillis > delay) { buttonPressed = -1; // They took to long to let the button up so they lose. } } } currentMillis = millis(); } return buttonPressed; } //New code void showSequenceHard() { //blinkRed(2); sequence[largestIndex] = random(0,5); Serial.println("Next out"); Serial.println(sequence[largestIndex]); largestIndex++; for(int index=0; index void readSequenceHard() { //blinkYellow(2); int positionPressed; boolean madeMistake = false; for(int index=0; index Serial.println("Pressed"); Serial.println(positionPressed); // count++; if(positionPressed == -1 | positionPressed != sequence[index]) { madeMistake = true; // Exit the loop. gameState = GAMEOVER; } } //blinkBlue(2); } void waitToStart() { int buttonPressed = -1; allOff(); for(int pin = 0; pin < 5; pin++) { if(buttonPressed == -1) { digitalWrite(leds[pin], HIGH); buttonPressed = waitForButton(800); digitalWrite(leds[pin], LOW); } } if(buttonPressed != -1) { // A button was pushed so wait then start playing. delay(3000); largestIndex = 0; // Restart gameState = PLAYEASY; } if (buttons[0] == HIGH) { // A button was pushed so wait then start playing. delay(3000); largestIndex = 0; // Restart gameState = PLAYHARD; } } // Turns all the LEDs off. void allOff() { for(int pin = 0; pin < 5; pin++) { digitalWrite(leds[pin], LOW); } } // Turns all the LEDs on. void allOn() { for(int pin = 0; pin < 5; pin++) { digitalWrite(leds[pin], HIGH); } } // Spins a loop until "delay" milliseconds passes. // While the loop is spinning we keep looking at the value of the button to see if pushed. boolean readAnyButton(int delay) { boolean buttonDown = false; currentMillis = millis(); // The number of ms since the program started running previousMillis = currentMillis; // Records the point when we start spinning the loop. // Keep spinning the loop until "delay" seconds have passed. while (currentMillis - previousMillis < delay & buttonDown == false) { // Read the button and record when it has been pushed down. for(int pin = 0; pin < 5; pin++) { if(digitalRead(buttons[pin]) == HIGH) { buttonDown = true; } } currentMillis = millis(); } return buttonDown; } void blinkAll(int times) { for(int count = 0; count < times; count++) { allOn(); delay(300); allOff(); delay(300); } } void blinkWhite(int times) { for(int count = 0; count < times; count++) { digitalWrite(leds[4], HIGH); delay(500); digitalWrite(leds[4], LOW); delay(500); } } void blinkRed(int times) { for(int count = 0; count < times; count++) { digitalWrite(leds[0], HIGH); delay(500); digitalWrite(leds[0], LOW); delay(500); } } void blinkGreen(int times) { for(int count = 0; count < times; count++) { digitalWrite(leds[1], HIGH); delay(500); digitalWrite(leds[1], LOW); delay(500); } } void blinkYellow(int times) { for(int count = 0; count < times; count++) { digitalWrite(leds[2], HIGH); delay(500); digitalWrite(leds[2], LOW); delay(500); } } void blinkBlue(int times) { for(int count = 0; count < times; count++) { digitalWrite(leds[3], HIGH); delay(500); digitalWrite(leds[3], LOW); delay(500); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
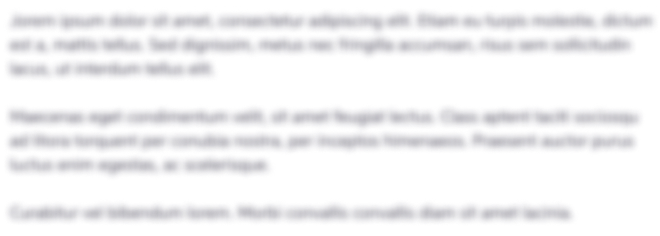
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started