Question
I am more familiar working with Java, so if I could have help with the pseudocode or actual code, that would be great. The sliding
I am more familiar working with Java, so if I could have help with the pseudocode or actual code, that would be great.
The sliding puzzle (https://en.wikipedia.org/wiki/Sliding_puzzle) is a game in which tiles are arranged on a 2D array of cells. One cell in the array is open. Moves are made by sliding one of the tiles into the open slot. the objective is to make a sequence of moves that puts the tiles in a goal state (in order). The sliding puzzle also is a great example of a problem that can be solved easily by recursion, but is hard to solve by iteration.
In this assignment, you will solve a weighted variant of the 4x4 sliding puzzle using an interative deepening search. In the normal sliding puzzle, each move has the same cost. However, in this variant of the problem, the cost of moving each piece is proportional to the value on the piece.
Iterative Deepening Search
Iterative deepening search is a recursive method to solve problems made up of a sequence of moves. The idea is to try all move sequences of length or cost 1, then all sequences of length 2, and so forth, until a solution is found. When a solution is found, the recursion unwinds, retracing the moves that were needed to solve the problem.
Input file format
The input format will be a text file which has 4 lines. giving the initial configuration of the board. The files will use Hexadecimal digits (0-9,A-F) to represent the values 1-15, and a dot (.) to represent the blank space. For example:
1 | 2 | 3 | 4 |
5 | 6 | 7 | 8 |
9 | A | B | C |
D | (.) | E | F |
The goal is to successively move squares up, down, right, or left into the blank spot until the goal configuration is reached, while incurring the least cost. For our purposes, the goal configuration is as follows:
1 | 2 | 3 | 4 |
5 | 6 | 7 | 8 |
9 | A | B | C |
D | E | F | (.) |
For the example above, the goal can be reached by moving the E piece and then F piece left one position, with a total cost of 29 (15 for moving the F, and 14 for moving the E).
Instructions
Use an iterative deepening search to solve the weighted 4x4 sliding puzzle problem. When a solution is found, print out the sequence of moves, as well as the total path cost. A move can be specified by listing which square was moved into the blank spot. For the example above, the solution to the example above could be printed as:
Path: E F
Cost: 29
Passoff
Write a program that solves the weighted 4x4 sliding puzzle using an iterative deepening search. When the program starts, it should print out your name, and state that it is a sliding puzzle solver. The user must also be able to specify the input file name. The program must read in 4x4 sliding puzzles from a file in the format described earlier. Your program must print out an optimal solution (sequence of moves, and total cost) by tracking which square is moved into the blank space at each move. In addition to the solution to the puzzle, the program must print out how many total recursive calls were made to find the solution.
(Optional) Optimize the search in some way. Some possibilities include:
-(easy) Prevent reversal moves (moving the same square twice in a row).
-(easy) Calculate a lower bound on the solution cost, and start the search there.
-(harder) Keep track of visited states, and disallow moves to the same state with higher cost.
-(harder) After implementing iterative deepening, implement an A* search to solve the puzzle.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
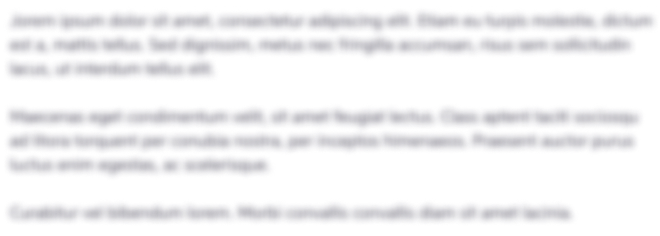
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started