Question
I am pasting here the header file, implementation file, and the main file for a program. I need the complete class implementation file that includes
I am pasting here the header file, implementation file, and the main file for a program. I need the complete class implementation file that includes the methods that I am attaching. Use the main file to test if the methods are working properly. Should receive the maximum number of points (30 maximum points) if everything is working properly. Also, add inline comments to describe your logic for the completed methods.
When everything is working right the program output should look like the following:
Header File:
// FILE: sequenceDynamic.h
// CLASS PROVIDED: sequenceDynamic (part of the namespace main_savitch_4)
// There is no implementation file provided for this class since it is
// an exercise from Chapter 4 of "Data Structures and Other Objects Using C++"
//
// ALIASES and MEMBER CONSTANTS for the sequenceDynamic class:
// alias ____ value_type
// sequenceDynamic::value_type is the data type of the items in the sequenceDynamic. It
// may be any of the C++ built-in types (int, char, etc.), or a class with a
// default constructor, an assignment operator, and a copy constructor.
//
// alias ____ size_type
// sequenceDynamic::size_type is the data type of any variable that keeps track of
// how many items are in a sequenceDynamic.
//
// static const size_type DEFAULT_CAPACITY = _____
// sequenceDynamic::DEFAULT_CAPACITY is the initial capacity of a sequenceDynamic that is
// created by the default constructor.
//
// DYNAMIC MEMORY USAGE by the List
// If there is insufficient dynamic memory, then the following functions
// throw a BAD_ALLOC exception: The constructors, insert, attach.
#ifndef MAIN_SAVITCH_sequenceDynamic_H
#define MAIN_SAVITCH_sequenceDynamic_H
#include
class sequenceDynamic
{
public:
// ALIAS and MEMBER CONSTANTS
using value_type = double;
using size_type = std::size_t;
static const size_type DEFAULT_CAPACITY = 30;
// CONSTRUCTORS and DESTRUCTOR
sequenceDynamic(size_type initial_capacity = DEFAULT_CAPACITY);
sequenceDynamic(const sequenceDynamic& source);
~sequenceDynamic( );
// CONSTANT MEMBER FUNCTIONS
size_type size() const;
bool is_item() const;
value_type current() const;
// MODIFICATION MEMBER FUNCTIONS
void resize(size_type new_capacity);
void start( );
void advance( );
void insert(const value_type& entry);
void attach(const value_type& entry);
void remove_current( );
bool erase_one(const value_type& entry);
void operator =(const sequenceDynamic& source);
void operator +=(const sequenceDynamic& addend);
private:
value_type* data;
size_type used;
size_type current_index;
size_type capacity;
};
sequenceDynamic operator +(const sequenceDynamic& s1, const sequenceDynamic& s2); // added this overloaded operator
#endif
Implementation File:
// FILE: sequenceDynamic.cpp
// CLASS IMPLEMENTED: sequenceDynamic (See sequenceDynamic.h for documentation)
// INVARIANT for the sequenceDynamic ADT (i.e. verbalization of rules governing
// private member function behavior):
// 1. The number of value_types in the sequenceDynamic is in the member variable used
// (i.e. used = 0 means the sequenceDynamic is empty).
// 2. For an empty sequenceDynamic, we do not care what is stored in any of the
// array data. For a non-empty sequenceDynamic, the value_types in the sequenceDynamic are
// stored in strictly maintained order in data[0] through
// data[used]. We don't care what's in the rest of data (so don't
// need to initialize data in the constructor).
// 3. To maintain strict order, current_index is used to keep track
// of current location in data; when current_index is used-1,
// we're at the last place in the array. Also whenever current_index
// is greater than or equal to used, there is no current value_type.
// 4. capacity keeps track of the current capacity of the array pointed
// to by data; capacity starts out with the value initial_capacity
// and is changed by resize as needed (whenever used is greater than
// or equal to capacity).
#include
#include "sequenceDynamic.h"
using namespace std;
// sequenceDynamic(size_t initial_capacity = DEFAULT_CAPACITY)
// Postcondition: The sequenceDynamic has been initialized as an empty sequenceDynamic.
// The insert/attach functions will work efficiently (without allocating
// new memory) until this capacity is reached.
//
sequenceDynamic::sequenceDynamic(size_type initial_capacity) {
// create sequence of initial_capacity
data = new value_type[initial_capacity];
capacity = initial_capacity;
used = 0;
current_index = 0;
}
// sequenceDynamic(const sequenceDynamic& source)
// Postcondition: The sequenceDynamic has capacity set to the sequenceDynamic source parameter.
// The sequenceDynamic object is filled with data members given by the sequenceDynamic source
// parameter.
//
sequenceDynamic::sequenceDynamic(const sequenceDynamic& source) {
// copy constructor ==>TO COMPLETE FOR LAB
}
// ~sequenceDynamic()
// Postcondition: dynamically allocated memory for data member has been released
//
sequenceDynamic::~sequenceDynamic() {
// release memory
delete [ ] data;
}
// size_type count(const value_type& target) const
// Postcondition: Return value is number of times target is in the sequenceDynamic
//
sequenceDynamic::size_type sequenceDynamic::size() const {
return used;
}
// bool is_item( ) const
// Postcondition: A true return value indicates that there is a valid
// "current" item that may be retrieved by activating the current
// member function (listed below). A false return value indicates that
// there is no valid current item.
//
bool sequenceDynamic::is_item() const {
// see if current_index is valid
return (current_index
}
// value_type current( ) const
// Precondition: is_item( ) returns true.
// Postcondition: The item returned is the current item in the sequenceDynamic.
//
sequenceDynamic::value_type sequenceDynamic::current() const {
// ensure that current_index is valid before returning value
assert(is_item());
return data[current_index];
}
// void resize(size_type new_capacity)
// Postcondition: The sequenceDynamic's current capacity is changed to the
// new_capacity (but not less that the number of items already on the
// list). The insert/attach functions will work efficiently (without
// allocating new memory) until this new capacity is reached.
//
void sequenceDynamic::resize(size_type new_capacity) {
// resize member function ==>TO COMPLETE FOR LAB
}
// void start( )
// Postcondition: The first item on the sequenceDynamic becomes the current item
// (but if the sequenceDynamic is empty, then there is no current item).
//
void sequenceDynamic::start() {
current_index = 0;
}
// void advance( )
// Precondition: is_item returns true.
// Postcondition: If the current item was already the last item in the
// sequenceDynamic, then there is no longer any current item. Otherwise, the new
// current item is the item immediately after the original current item.
//
void sequenceDynamic::advance() {
// ensure that current_index is valid
assert(is_item());
current_index++;
}
// void insert(const value_type& entry)
// Postcondition: A new copy of entry has been inserted in the sequenceDynamic
// before the current item. If there was no current item, then the new entry
// has been inserted at the front of the sequenceDynamic. In either case, the newly
// inserted item is now the current item of the sequenceDynamic.
//
void sequenceDynamic::insert(const value_type& entry) {
// insert member function ==>TO COMPLETE FOR LAB
}
// void attach(const value_type& entry)
// Postcondition: A new copy of entry has been inserted in the sequenceDynamic after
// the current item. If there was no current item, then the new entry has
// been attached to the end of the sequenceDynamic. In either case, the newly
// inserted item is now the current item of the sequenceDynamic.
//
void sequenceDynamic::attach(const value_type& entry) {
// add additional memory if at capacity
if (used >= capacity)
resize (1 + used + used/10); // Increase by 10%
// If there is no current item,
// add item at end of sequence
if (!is_item())
{
current_index = used;
data[current_index] = entry;
}
else // There is a current item
{
// so move items over to make room
for(size_type i = used; i > (current_index + 1); i--)
data[i] = data[i-1];
// insert item after current_index
data[current_index + 1] = entry;
// increment current_index
current_index++;
}
// increment number of items used
used++;
}
// void remove_current( )
// Precondition: is_item returns true.
// Postcondition: The current item has been removed from the sequenceDynamic, and the
// item after this (if there is one) is now the new current item.
//
void sequenceDynamic::remove_current() {
// ensure that current_index is valid
assert(is_item());
// remove item at current_index by shifting items over
for(size_type i = current_index; i
data[i] = data[i+1];
// decrement number of items used
used--;
}
// bool erase_one(const value_type& entry)
// Precondition: used > 0
// Postcondition: If entry was in the sequenceDynamic,
// then one copy has been removed; otherwise the
// sequenceDynamic is unchanged. A true return value
// indicates that the first copy was removed;
// false indicates that nothing was removed.
// Current item is set to first item in sequenceDynamic.
bool sequenceDynamic::erase_one(const value_type& entry) {
// erase_one member function ==>TO COMPLETE FOR LAB
// use flag to keep track of whether item is removed
bool found = false;
return found;
}
// Overloaded = operator (as member function)
void sequenceDynamic::operator =(const sequenceDynamic& source) {
// overloaded assignment operator ==>TO COMPLETE FOR LAB
}
// Overloaded += operator (as member function)
void sequenceDynamic::operator +=(const sequenceDynamic& addend) {
// see if additional memory needed
if((used + addend.used) > capacity)
resize(used + addend.used);
// copy items from addend to current
size_type addUsed = addend.used;
for (size_type i = 0; i
data[used++] = addend.data[i];
}
// Overloaded + operator (as free function)
sequenceDynamic operator +(const sequenceDynamic& s1, const sequenceDynamic& s2) {
// create new bagDynamic with size equal to sum of both bagDynamic parameters
sequenceDynamic result(s1.size() + s2.size());
// add items from first sequenceDynamic
result += s1;
// add items from first sequenceDynamic
result += s2;
return result;
}
Main File:
// FILE: sequenceDyanmic_exam.cxx
// Written by: Michael Main (main@colorado.edu) - Oct 29, 1997
// Revised by: CREngland (england@cod.edu) 09/18/2018
// Non-interactive test program for the sequenceDynamic class using a dynamic array,
// with improved test for heap leaks.
//
// DESCRIPTION:
// Each function of this program tests part of the sequenceDynamic class, returning
// some number of points to indicate how much of the test was passed.
// A description and result of each test is printed to cout.
//
// Maximum number of points awarded by this program is determined by the
// constants POINTS[1], POINTS[2]...
#include
#include
#include
#include "sequenceDynamic.h" // Provides the sequenceDynamic class with double items.
using namespace std;
// Descriptions and points for each of the tests:
const size_t MANY_TESTS = 8;
const int POINTS[MANY_TESTS+1] = {
30, // Total points for all tests.
3, // Test 1 points
0, // Test 2 points
0, // Test 3 points
5, // Test 4 points
5, // Test 5 points
6, // Test 6 points
3, // Test 7 points
8, // Test 8 points
};
const char DESCRIPTION[MANY_TESTS+1][256] = {
"Tests for sequenceDynamic class with a dynamic array",
"Testing insert, attach, and the constant member functions",
"Testing situations where the cursor goes off the sequenceDynamic",
"Testing remove_current member function",
"Testing the resize member function",
"Testing the copy constructor",
"Testing the assignment operator",
"Testing insert/attach when current DEFAULT_CAPACITY exceeded",
"Testing erase_one member function"
};
// **************************************************************************
// bool test_basic(const sequenceDynamic& test, size_t s, bool has_cursor)
// Postcondition: A return value of true indicates:
// a. test.size() is s, and
// b. test.is_item() is has_cursor.
// Otherwise the return value is false.
// In either case, a description of the test result is printed to cout.
// **************************************************************************
bool test_basic(const sequenceDynamic& test, size_t s, bool has_cursor)
{
bool answer;
cout
cout.flush( );
answer = (test.size( ) == s);
cout
if (answer)
{
cout
cout
cout.flush( );
answer = (test.is_item( ) == has_cursor);
cout
}
return answer;
}
// **************************************************************************
// bool test_items(sequenceDynamic& test, size_t s, size_t i, double items[])
// The function determines if the test sequenceDynamic has the correct items
// Precondition: The size of the items array is at least s.
// Postcondition: A return value of true indicates that test.current()
// is equal to items[i], and after test.advance() the result of
// test.current() is items[i+1], and so on through items[s-1].
// At this point, one more advance takes the cursor off the sequenceDynamic.
// If any of this fails, the return value is false.
// NOTE: The test sequenceDynamic has been changed by advancing its cursor.
// **************************************************************************
bool test_items(sequenceDynamic& test, size_t s, size_t i, double items[])
{
bool answer = true;
cout
cout
cout
cout.flush( );
while ((i
{
i++;
test.advance( );
}
if ((i != s) && !test.is_item( ))
{ // The test.is_item( ) function returns false too soon.
cout
answer = false;
}
else if (i != s)
{ // The test.current( ) function returned a wrong value.
cout
cout
answer = false;
}
else if (test.is_item( ))
{ // The test.is_item( ) function returns true after moving off the sequenceDynamic.
cout
cout
answer = false;
}
cout
return answer;
}
// **************************************************************************
// bool correct(sequenceDynamic& test, size_t s, size_t cursor_spot, double items[])
// This function determines if the sequenceDynamic (test) is "correct" according to
// these requirements:
// a. it has exactly s items.
// b. the items (starting at the front) are equal to
// items[0] ... items[s-1]
// c. if cursor_spot
// the location given by cursor_spot.
// d. if cursor_spot >= s, then test must not have a cursor.
// NOTE: The function also moves the cursor off the sequenceDynamic.
// **************************************************************************
bool correct(sequenceDynamic& test, size_t size, size_t cursor_spot, double items[])
{
bool has_cursor = (cursor_spot
// Check the sequenceDynamic's size and whether it has a cursor.
if (!test_basic(test, size, has_cursor))
{
cout
return false;
}
// If there is a cursor, check the items from cursor to end of the sequenceDynamic.
if (has_cursor && !test_items(test, size, cursor_spot, items))
{
cout
return false;
}
// Restart the cursor at the front of the sequenceDynamic and test items again.
cout
test.start( );
if (has_cursor && !test_items(test, size, 0, items))
{
cout
return false;
}
// If the code reaches here, then all tests have been passed.
cout
return true;
}
// **************************************************************************
// int test1( )
// Performs some basic tests of insert, attach, and the constant member
// functions. Returns POINTS[1] if the tests are passed. Otherwise returns 0.
// **************************************************************************
int test1( )
{
sequenceDynamic empty; // An empty sequenceDynamic
sequenceDynamic test; // A sequenceDynamic to add items to
double items1[4] = { 5, 10, 20, 30 }; // These 4 items are put in a sequenceDynamic
double items2[4] = { 10, 15, 20, 30 }; // These are put in another sequenceDynamic
// Test that the empty sequenceDynamic is really empty
cout
if (!correct(empty, 0, 0, items1)) return 0;
// Test the attach function to add something to an empty sequenceDynamic
cout
test.attach(10);
if (!correct(test, 1, 0, items2)) return 0;
// Test the insert function to add something to an empty sequenceDynamic
cout
test = empty;
test.insert(10);
if (!correct(test, 1, 0, items2)) return 0;
// Test the insert function to add an item at the front of a sequenceDynamic
cout
cout
test = empty;
test.attach(10);
test.attach(20);
test.attach(30);
test.start( );
test.insert(5);
if (!correct(test, 4, 0, items1)) return 0;
// Test the insert function to add an item in the middle of a sequenceDynamic
cout
cout
cout
test = empty;
test.attach(10);
test.attach(20);
test.attach(30);
test.start( );
test.advance( );
test.insert(15);
if (!correct(test, 4, 1, items2)) return 0;
// Test the attach function to add an item in the middle of a sequenceDynamic
cout
cout
cout
test = empty;
test.attach(10);
test.attach(20);
test.attach(30);
test.start( );
test.attach(15);
if (!correct(test, 4, 1, items2)) return 0;
// All tests have been passed
cout
return POINTS[1];
}
// **************************************************************************
// int test2( )
// Performs a test to ensure that the cursor can correctly be run off the end
// of the sequenceDynamic. Also tests that attach/insert work correctly when there is
// no cursor. Returns POINTS[2] if the tests are passed. Otherwise returns 0.
// **************************************************************************
int test2( )
{
sequenceDynamic test;
size_t i;
// Put three items in the sequenceDynamic
cout
cout
cout.flush( );
test.attach(20);
test.attach(30);
test.advance( );
if (test.is_item( ))
{
cout
return -5; // 0;
}
cout
// Insert 10 at the front and run the cursor off the end again
cout
cout
cout.flush( );
test.insert(10);
test.advance( ); // advance to the 20
test.advance( ); // advance to the 30
test.advance( ); // advance right off the sequenceDynamic
if (test.is_item( ))
{
cout
return false;
}
cout
// Attach more items until the sequenceDynamic becomes full.
// Note that the first attach should attach to the end of the sequenceDynamic.
cout
cout
for (i = 4; i
test.attach(i*10);
// Test that the sequenceDynamic is correctly filled.
cout
cout
test.start( );
for (i = 1; i
{
if ((!test.is_item( )) || test.current( ) != i*10)
{
cout
return -5; // 0;
}
test.advance( );
}
if (test.is_item( ))
{
cout
return false;
}
// All tests passed
cout
return POINTS[2];
}
// **************************************************************************
// int test3( )
// Performs basic tests for the remove_current function.
// Returns POINTS[3] if the tests are passed. Returns POINTS[3] / 4 if almost
// all the tests are passed. Otherwise returns 0.
// **************************************************************************
int test3( )
{
// In the next declarations, I am declaring a sequenceDynamic called test.
// Both before and after the sequenceDynamic, I declare a small array of characters,
// and I put the character 'x' into each spot of these arrays.
// Later, if I notice that one of the x's has been changed, or if
// I notice an 'x' inside of the sequenceDynamic, then the most
// likely reason was that one of the sequenceDynamic's member functions accessed
// the sequenceDynamic's array outside of its legal indexes.
char prefix[4] = {'x', 'x', 'x', 'x'};
sequenceDynamic test;
char suffix[4] = {'x', 'x', 'x', 'x'};
// Within this function, I create several different sequenceDynamics using the
// items in these arrays:
double items1[1] = { 30 };
double items2[2] = { 10, 30 };
double items3[3] = { 10, 20, 30 };
size_t i; // for-loop control variable
char *char_ptr; // Variable to loop at each character in a sequenceDynamic's memory
// Build a sequenceDynamic with three items 10, 20, 30, and remove the middle,
// and last and then first.
cout
test.attach(10);
test.attach(30);
cout
test.insert(20);
if (!correct(test, 3, 1, items3)) return 0;
cout
test.start( );
test.advance( );
test.remove_current( );
if (!correct(test, 2, 1, items2)) return 0;
cout
cout
test.start( );
test.advance( );
test.remove_current( );
if (!correct(test, 1, 1, items2)) return 0;
cout
test.start( );
test.remove_current( );
if (!correct(test, 0, 0, items2)) return 0;
// Build a sequenceDynamic with three items 10, 20, 30, and remove the middle,
// and then first and then last.
cout
test.attach(10);
test.attach(30);
cout
test.insert(20);
if (!correct(test, 3, 1, items3)) return 0;
cout
test.start( );
test.advance( );
test.remove_current( );
if (!correct(test, 2, 1, items2)) return 0;
cout
cout
test.start( );
test.remove_current( );
if (!correct(test, 1, 0, items1)) return 0;
cout
test.start( );
test.remove_current( );
if (!correct(test, 0, 0, items1)) return 0;
// Build a sequenceDynamic with three items 10, 20, 30, and remove the first.
cout
cout
test.insert(30);
test.insert(10);
test.insert(20);
test.remove_current( );
if (!correct(test, 2, 0, items2)) return 0;
test.start( );
test.remove_current( );
test.remove_current( );
// Just for fun, fill up the sequenceDynamic, and empty it!
cout
cout
cout
cout
for (i = 0; i
test.insert(0);
for (i = 0; i
test.remove_current( );
// Make sure that the character 'x' didn't somehow get into the sequenceDynamic,
// as that would indicate that the sequenceDynamic member functions are
// copying data from before or after the sequenceDynamic into the sequenceDynamic.
char_ptr = (char *) &test;
for (i = 0; i
if (char_ptr[i] == 'x')
{
cout
return POINTS[3] / 4;
}
// Make sure that the prefix and suffix arrays still have four
// x's each. Otherwise one of the sequenceDynamic operations wrote outside of
// the legal boundaries of its array.
for (i = 0; i
if ((suffix[i] != 'x') || (prefix[i] != 'x'))
{
cout
return POINTS[3] / 4;
}
// All tests passed
cout
return POINTS[3];
}
// **************************************************************************
// int test4( )
// Performs some tests of resize.
// Returns POINTS[4] if the tests are passed. Otherwise returns 0.
// **************************************************************************
int test4( )
{
sequenceDynamic test;
size_t i;
char bytes[sizeof(sequenceDynamic)];
char newbytes[sizeof(sequenceDynamic)];
size_t mismatches;
cout
cout
cout
test.resize(2*test.DEFAULT_CAPACITY);
test.attach(0);
memcpy(bytes, (char *) &test, sizeof(sequenceDynamic));
// At this point, I should be able to insert 2*DEFAULT_CAPACITY-1
// more items without calling resize again. Therefore, at most 1 byte
// of the object will change (the least significant byte of used).
for (i = 1; i
test.attach(i);
test.start( );
memcpy(newbytes, (char *) &test, sizeof(sequenceDynamic));
for (i = 0; i
{
if (test.current( ) != i)
{
cout
return 0;
}
test.advance( );
}
test.start( );
mismatches = 0;
for (i = 0; i
if (bytes[i] != newbytes[i])
mismatches++;
if (mismatches > 1)
{
cout
return 0;
}
else
cout
cout
cout
cout
memcpy(bytes, (char *) &test, sizeof(sequenceDynamic));
test.resize(1);
mismatches = 0;
for (i = 0; i
if (bytes[i] != newbytes[i])
mismatches++;
if (mismatches > 0)
{
cout
return 0;
}
else
cout
// All tests passed
cout
return POINTS[4];
}
// **************************************************************************
// int test5( )
// Performs some tests of the copy constructor.
// Returns POINTS[5] if the tests are passed. Otherwise returns 0.
// **************************************************************************
int test5( )
{
sequenceDynamic original; // A sequenceDynamic that we'll copy.
double items[2*original.DEFAULT_CAPACITY];
size_t i;
// Set up the items array to conatin 1...2*DEFAULT_CAPACITY.
for (i = 1; i
items[i-1] = i;
// Test copying of an empty sequenceDynamic. After the copying, we change the original.
cout
sequenceDynamic copy1(original);
original.attach(1); // Changes the original sequenceDynamic, but not the copy.
if (!correct(copy1, 0, 0, items)) return 0;
// Test copying of a sequenceDynamic with current item at the tail.
cout
for (i=2; i
original.attach(i);
sequenceDynamic copy2(original);
original.start( );
original.advance( );
original.remove_current( ); // Removes 2 from the original, but not the copy.
if (!correct
(copy2, 2*original.DEFAULT_CAPACITY, 2*original.DEFAULT_CAPACITY-1, items)
)
return 0;
// Test copying of a sequenceDynamic with cursor near the middle.
cout
original.insert(2);
for (i = 1; i
original.advance( );
// Cursor is now at location [DEFAULT_CAPACITY] (counting [0] as the first spot).
sequenceDynamic copy3(original);
original.start( );
original.advance( );
original.remove_current( ); // Removes 2 from the original, but not the copy.
if (!correct
(copy3, 2*original.DEFAULT_CAPACITY, original.DEFAULT_CAPACITY, items)
)
return 0;
// Test copying of a sequenceDynamic with cursor at the front.
cout
original.insert(2);
original.start( );
// Cursor is now at the front.
sequenceDynamic copy4(original);
original.start( );
original.advance( );
original.remove_current( ); // Removes 2 from the original, but not the copy.
if (!correct
(copy4, 2*original.DEFAULT_CAPACITY, 0, items)
)
return 0;
// Test copying of a sequenceDynamic with no current item.
cout
original.insert(2);
while (original.is_item( ))
original.advance( );
// There is now no current item.
sequenceDynamic copy5(original);
original.start( );
original.advance( );
original.remove_current( ); // Removes 2 from the original, but not the copy.
if (!correct
(copy5, 2*original.DEFAULT_CAPACITY, 2*original.DEFAULT_CAPACITY, items)
)
return 0;
// All tests passed
cout
return POINTS[5];
}
// **************************************************************************
// int test6( )
// Performs some tests of the assignment operator.
// Returns POINTS[6] if the tests are passed. Otherwise returns 0.
// **************************************************************************
int test6( )
{
sequenceDynamic original; // A sequenceDynamic that we'll copy.
double items[2*original.DEFAULT_CAPACITY];
size_t i;
// Set up the items array to conatin 1...2*DEFAULT_CAPACITY.
for (i = 1; i
items[i-1] = i;
// Test copying of an empty sequenceDynamic. After the copying, we change the original.
cout
sequenceDynamic copy1;
copy1 = original;
original.attach(1); // Changes the original sequenceDynamic, but not the copy.
if (!correct(copy1, 0, 0, items)) return 0;
// Test copying of a sequenceDynamic with current item at the tail.
cout
for (i=2; i
original.attach(i);
sequenceDynamic copy2;
copy2 = original;
original.start( );
original.advance( );
original.remove_current( ); // Removes 2 from the original, but not the copy.
if (!correct
(copy2, 2*original.DEFAULT_CAPACITY, 2*original.DEFAULT_CAPACITY-1, items)
)
return 0;
// Test copying of a sequenceDynamic with cursor near the middle.
cout
original.insert(2);
for (i = 1; i
original.advance( );
// Cursor is now at location [DEFAULT_CAPACITY] (counting [0] as the first spot).
sequenceDynamic copy3;
copy3 = original;
original.start( );
original.advance( );
original.remove_current( ); // Removes 2 from the original, but not the copy.
if (!correct
(copy3, 2*original.DEFAULT_CAPACITY, original.DEFAULT_CAPACITY, items)
)
return 0;
// Test copying of a sequenceDynamic with cursor at the front.
cout
original.insert(2);
original.start( );
// Cursor is now at the front.
sequenceDynamic copy4;
copy4 = original;
original.start( );
original.advance( );
original.remove_current( ); // Removes 2 from the original, but not the copy.
if (!correct
(copy4, 2*original.DEFAULT_CAPACITY, 0, items)
)
return 0;
// Test copying of a sequenceDynamic with no current item.
cout
original.insert(2);
while (original.is_item( ))
original.advance( );
// There is now no current item.
sequenceDynamic copy5;
copy5 = original;
original.start( );
original.advance( );
original.remove_current( ); // Removes 2 from the original, but not the copy.
if (!correct
(copy5, 2*original.DEFAULT_CAPACITY, 2*original.DEFAULT_CAPACITY, items)
)
return 0;
cout
original.insert(2);
original = original;
if (!correct
(original, 2*original.DEFAULT_CAPACITY, 1, items)
)
return 0;
// All tests passed
cout
return POINTS[6];
}
// **************************************************************************
// int test7( )
// Performs some basic tests of insert and attach for the case where the
// current capacity has been reached.
// Returns POINTS[7] if the tests are passed. Otherwise returns 0.
// **************************************************************************
int test7( )
{
sequenceDynamic testa, testi;
double items[2*testa.DEFAULT_CAPACITY];
size_t i;
// Set up the items array to conatin 1...2*DEFAULT_CAPACITY.
for (i = 1; i
items[i-1] = i;
cout
cout
for (i = 1; i
testa.attach(i);
if (!correct
(testa, 2*testa.DEFAULT_CAPACITY, 2*testa.DEFAULT_CAPACITY-1, items)
)
return 0;
cout
cout
for (i = 2*testi.DEFAULT_CAPACITY; i >= 1; i--)
testi.insert(i);
if (!correct
(testi, 2*testi.DEFAULT_CAPACITY, 0, items)
)
return 0;
// All tests passed
cout
return POINTS[7];
}
// **************************************************************************
// int test8( )
// Performs a test to ensure that the erase_one(...) correctly removes
// an item of the sequenceDynamic. Returns POINTS[8] if the tests are passed.
// Otherwise returns 0.
// **************************************************************************
int test8()
{
sequenceDynamic testDup, testUnique;
size_t i, j;
// array of duplicate items
int items3[3] = { 10, 10, 10 };
size_t arrSize3 = sizeof(items3) / sizeof(int);
// array of unique items
int items5[5] = { 10, 20, 30, 40, 50 };
size_t arrSize5 = sizeof(items5) / sizeof(int);
// put items in the sequenceDynamic of duplicates
cout
for (i = 0; i
cout
testDup.attach(items3[i]);
}
cout
// try to erase item not found
cout
if (testDup.erase_one(100)) {
if (testDup.size() != arrSize3) {
cout
return 0;
}
}
cout
// remove items from sequenceDynamic of duplicates
cout
for (i = 0; i
if (testDup.erase_one(items3[i])) {
// position cursor at beginning
testDup.start();
// check size after removed
if (testDup.size() + 1 != arrSize3 - i) {
cout
return 0;
}
cout
// check that all remaining items are in sequence
for (j = i + 1; j
if (testDup.current() != items3[j]) {
cout
return 0;
}
else
testDup.advance();
}
}
}
cout
// put items in the sequenceDynamic of unique items
cout
for (i = 0; i
cout
testUnique.attach(items5[i]);
}
cout
// remove all items from non-duplicate array
cout
for (i = 0; i
if (testUnique.erase_one(items5[i])) {
// position cursor at beginning
testUnique.start();
// check size after removed
if (testUnique.size() + 1 != arrSize5 - i) {
cout
return 0;
}
cout
// check that all remaining items are in sequence
for (j = i + 1; j
if (testUnique.current() != items5[j]) {
cout
return 0;
}
else
testUnique.advance();
}
}
}
cout
// All tests passed
cout
return POINTS[8];
}
int run_a_test(int number, const char message[], int test_function( ), int max)
{
int result;
cout
cout
result = test_function( );
if (result > 0)
{
cout
cout
}
else
cout
cout
return result;
}
// **************************************************************************
// int main( )
// The main program calls all tests and prints the sum of all points
// earned from the tests.
// **************************************************************************
int main( )
{
int stuPoints[MANY_TESTS+1], sum = 0;
cout
// run tests and identify points awarded
stuPoints[1] = run_a_test(1, DESCRIPTION[1], test1, POINTS[1]);
stuPoints[2] = run_a_test(2, DESCRIPTION[2], test2, POINTS[2]);
stuPoints[3] = run_a_test(3, DESCRIPTION[3], test3, POINTS[3]);
stuPoints[4] = run_a_test(4, DESCRIPTION[4], test4, POINTS[4]);
stuPoints[5] = run_a_test(5, DESCRIPTION[5], test5, POINTS[5]);
stuPoints[6] = run_a_test(6, DESCRIPTION[6], test6, POINTS[6]);
stuPoints[7] = run_a_test(7, DESCRIPTION[7], test7, POINTS[7]);
stuPoints[8] = run_a_test(8, DESCRIPTION[8], test8, POINTS[8]);
// accumulate points
for (int i = 1; i
sum += stuPoints[i];
// output testing results
cout TEST RESULTS
cout
cout
cout
cout POINT SUMMARY
for (int i = 1; i
cout
return EXIT_SUCCESS;
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
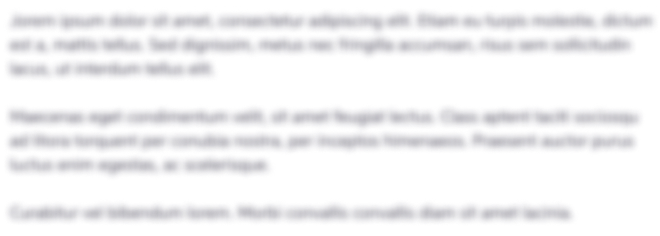
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started