Question
I am struggling with writing the program after task 5 and on. I put the code I already have down below from super class, to
I am struggling with writing the program after task 5 and on. I put the code I already have down below from super class, to subclass, to demo. If someone can model what it should look like that would be great. It's fine if you use a different program to example it; I just need to visually see it put together to understand.
Assignment:
1. Create a subclass of the class you created in Assignment 2. (10 point)
2. Create at least 1 instance field (attribute) for your subclass (10 point)
3. Create getter (accessor) and setter (mutator) methods for the new instance field (10 point)
4. Create a Method to display your data (15 point)
Create a method called display, that simply prints out the values of all instance variables of your object
It must display all the fields from the super class, along with the new field that belongs to the subclass
This method will have no parameters and not return a value
5. Create 2 Constructors
Create a default constructor (no parameters) that assigns all your instance variables to default values. It should also set the fields for the super class as well. (10 point)
Create a parameterized constructor that takes all instance variables (from both superclass and subclass) as parameters, and sets the instance variables to the values provided by the parameters (15 point)
6. Testing your program. In the main method of Demo.java:
Create an instance of your new subclass (an object) by using the default constructor.
Call all your objects setter (mutator) methods to assign values to your object (5 point)
Call the objects display method, to print out it's values (5 point)
Create another instance of your class (another object) by using the parameterized constructor (10 point)
Call the objects display method, to print out it's values
7. Write Javadoc style comments for ALL of your methods. Including the methods in your super class. (10 point)
Super Class:
/**
* Hannah Frickey
* CS108-03
* Sep 20, 2017
*/
/**
* Java SuperClass Dog
* Creates private instance fields
*
*
*/
public class Dogs {
private int numDogs;
private String colorFur;
private boolean goodDog;
/**
* default constructor
*/
public Dogs(){
numDogs = 0;
colorFur = null;
goodDog = false;
}
/**
* overload constructor, sets parameters
* @param numDogsPark
* @param dogFurColor
* @param goodDoggy
*/
public Dogs(int numDogsPark, String dogFurColor, boolean goodDoggy){
numDogs = numDogsPark;
colorFur = dogFurColor;
goodDog = goodDoggy;
}
/**
* Mutator method for int field
* @param numDogsPark
*/
public void setNum(int numDogsPark) {
numDogs = numDogsPark;
return;
}
/**
* mutator method for String field
* @param dogFurColor
*/
public void setColor(String dogFurColor){
colorFur = dogFurColor;
return;
}
/**
* mutator method for Boolean field
* @param goodDoggy
*/
public void setBehavior(boolean goodDoggy){
goodDog = goodDoggy;
return;
}
/**
* accessor for int field
* @return
*/
public int getNum(){
return numDogs;
}
/**
* accessor for String field
* @return
*/
public String getColor(){
return colorFur;
}
/**
* accessor for Boolean field
* @return
*/
public boolean getBehavior(){
return goodDog;
}
/**
* generic method to display values, will be called in Demo.java
*/
public void Display(){
System.out.println(numDogs);
System.out.println(colorFur);
System.out.println(goodDog);
}
Subclass:
public class DogsSubclass extends Dogs {
private String dogType;
public void setDogType(String coolDogType){
dogType = coolDogType;
return;
}
public DogsSubclass() {
Dogs dog1 = new Dogs();
DogsSubclass dog2 = new DogsSubclass();
dog1.setNum(getNum());
dog1.setColor(getColor());
dog1.setBehavior(getBehavior());
dog1.Display();
dog2.setNum(getNum());
dog2.setColor(getColor());
dog2.setBehavior(getBehavior());
dog2.setDogType(getDogType());
dog2.Display();
}
public void Display(){
System.out.println(dogType);
}
public String getDogType() {
return dogType;
}
}
Demo:
public class Demo {
/**
* main method. Displays a default object and an object using parameterized constructors
* @param args
*/
public static void main(String[] args) {
Dogs dog1 = new Dogs();
dog1.setNum(0);
dog1.setColor(null);
dog1.setBehavior(false);
dog1.Display();
Dogs dog2 = new Dogs(3, "red", true);
dog2.Display();
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
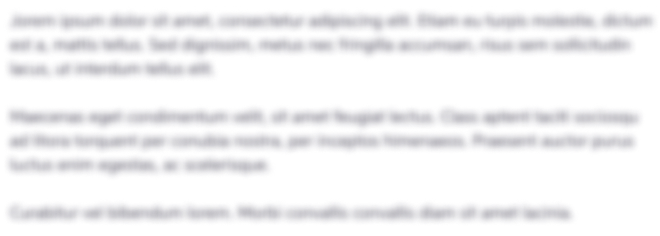
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started