Question
I am trying to create a Tweet Manager, but I'm stuck. I found similar questions online, but all of those have errors of some sort
I am trying to create a Tweet Manager, but I'm stuck. I found similar questions online, but all of those have errors of some sort as well. My main problem is I am not sure how to save the tweets in a file named "tweets.dat". I tried to write the code for it, but it does not work. Therefore when I try to search tweets or look at the recent tweets it says there are none. The recent tweets option is supposed to show the last 5 tweets entered, and the search option is supposed to only show tweets that contain a word you entered after the question. Another issue I have is that if I enter letters when it asks "what would you like to do?" I get an error when I want it to prompt the menu again if that happens. Below I have posted the instructions for what the program is supposed to contain and accomplish along with the code I have written so far. (There are 2 things: one is Tweet.py and the other is twitter.py) I am appreciative of any help given whether that is fixing my code or writing new code that works!
Requirements Tweet Class
Write a class named Tweet and save it as a file named Tweet.py. The Tweet class should have the following data attributes:
__author To store the name of whoever is making the tweet. __text To store the tweet itself. __age To store the time when the tweet was created.
The Tweet class should also have the following methods:
__init__
get_author get_text get_age
This method should take two parameters, the author and text of the tweet. It should create __author and __text based on those parameters and it should create __age based on the current time.
Returns the value of the __author field.
Returns the value of the __text field.
This method calculates the difference between the current time and the value of the __age field. It should return a string based on the age of the tweet. For example, this method should return the following:
30s if the tweet was posted 30 seconds ago 15m if the tweet was posted 15 minutes ago 1h if the tweet was posted 1 hour, 10 minutes, and 42 seconds ago
* Note that the string returned from __get_age is only concerned with the largest unit of time. If the tweet is 0 to 59 seconds old, the value returned from __get_age will end with an s for seconds. If the tweet is some number of minutes old, it will end with an m and ignore the seconds. Likewise, if the tweet is 1 or more hours old, it will ignore both the minutes and seconds.
To work with time, youll need to use Pythons time module. The documentation for that module is available here: https://docs.python.org/3.4/library/time.html
Tweet Manager
Write a program named twitter.py. This program will have 4 functional parts:
Tweet Menu ----------------
Make a Tweet
View Recent Tweets
Search Tweets
Quit
1. Make a Tweet
If the user chooses to make a tweet, they will be asked for their name and what they would like to tweet.
Twitter limits the length of tweets to 140 characters. Likewise, your program should check the length of the users tweet and re-prompt them if it is greater than 140 characters.
Use the users input and the Tweet class to create a new Tweet object.
2. View Recent Tweets
If the user chooses to view recent tweets, then display the 5 most recently created tweets including each tweets author, text, and age.
If there are no tweets, display a message indicating so.
InfoTc 1040 Introduction to Problem Solving and Programming Final Project: Tweet Manager
3. Search Tweets
Check to make sure there are tweets available to search. If there are no tweets, display a message indicating so.
If there are tweets, ask the user what they would like to search for. Look through the tweets and display only the tweets that contain that search.
The tweets should be displayed in chronological order, starting with the most recently created tweet.
If no tweets contain the users search, display a message indicating so.
4. Quit
If the user chooses to quit, the program is to exit.
Storing, Saving, and Loading Tweets
In order to view and search through your tweets, youll need to organize them in some way. One method for doing that would be to use a list. Whenever Tweet objects are created, add them to your list.
When the program ends, we dont want to lose our tweets. Each time you run the program, it should be possible to go back and view/search past tweets. To accomplish that, well need to save and load our list of tweets.
Here are a few of our suggestions: Whenever a new Tweet object is created, add it to your list. Then serialize your list and save it to a file.
Then each time the program starts, check for that file of tweets. If it does not exist, start with a new, empty list. If it does exist, you can read that file and de-serialize it back into a list of Tweet objects.
Your tweets should be saved in a file named tweets.dat
THE CODE TO COPY (Two Separate Things!):
twitter.py
import Tweet import pickle
MAKE = 1 VIEW = 2 SEARCH = 3 QUIT = 4
FILENAME = 'tweets.dat'
def main(): try: input_file = open(FILENAME, 'rb') tweet = pickle.load(input_file) input_file.close() except: tweetlist = [] while (True): ch = display_menu() if(ch==1): tweet_ques1 = input("What is your name? ") tweet_ques2 = input("What would you like to tweet? ") print() if len(tweet_ques2)>140: print("Tweets can only be 140 characters! ") else: print(tweet_ques1 +", your tweet has been saved.") age = 0 tweet = tweet.Tweet(tweet.ques1, tweet_ques2) tweetlist.append(tweet) try: output_file = open(FILENAME, 'wb') pickle.dump(tweet, output_file) output_file.close() except: print("Your tweet could not be saved!") print() elif(ch==2): print() print("Recent Tweets") print("--------------") if len(tweetlist)==0: print("There are no recent tweets. ") for tweets in tweetlist[-5]: print(tweet.get_ques1(),"-", tweet.get_age()) print(tweet.get_ques2(), " ") elif (ch==3): match=0 tweetlist.reverse() if tweetlist==[]: print("There are no tweets to search.") print() for tweet in tweetlist: search = input("What would you like to search for? ") if(search in tweet.get_text()): match=1 if match: print("Search Results") print("---------------") print(tweet.get_ques1(),"-",tweet.get_age()) print(tweet.get_ques2()," ") elif match == 0: print("No tweets contained",search," ") elif (ch==4): print("Thank you for using Tweet Manager!") exit() def display_menu(): print("Tweet Menu") print("-----------") print("1. Make a Tweet") print("2. View Recent Tweets") print("3. Search Tweets") print("4. Quit") print() try: choice = int(input("What would you like to do? ")) if choice < MAKE or choice > QUIT: print("Please select a valid option.") print() except ValueError: print("Please enter a numeric value.")
return choice
main()
Tweet.py
import time
class Tweet:
def __init__(self, author, text):
self.__author = author
self.__text = text
self.__age = time.time()
def get_author(self):
return self.__author
def get_text(self):
return self.__text
def get_age(self):
now = time.time()
difference = now - self.__time
hours = difference // 3600
difference = difference % 3600
minutes = difference // 60
seconds = difference % 60
# Truncate units of time and convert them to strings for output
hours = str(int(hours))
minutes = str(int(minutes))
seconds = str(int(seconds))
# Return formatted units of time
return hours + ":" + minutes + ":" + seconds
Step by Step Solution
There are 3 Steps involved in it
Step: 1
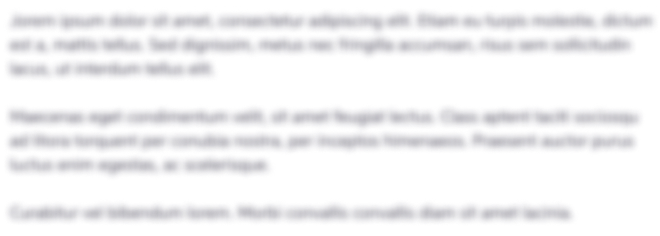
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started