Question
I am trying to figure out why the program is not calculating properly: include #include #include using namespace std; ifstream infile; ofstream outfile; //Maximum number
I am trying to figure out why the program is not calculating properly:
include #include #include
using namespace std;
ifstream infile; ofstream outfile;
//Maximum number of Chocolate Types const int ARRAY_SIZE = 25;
int printReport(int ChocTypeArray[], int ChocPiecesArray[]) { //Opens output file outfile.open("ChocolateCo_Production_Results.txt");
//Print Title outfile << "======================================================================================================" << endl; outfile << "================================ O-So-Good Chocolate Company Report ==================================" << endl; outfile << "======================================================================================================" << endl;
//Print Header outfile << "Chocolate Type" << setw(25) << "Pieces" << setw(25) << "Batches" << setw(25) << "Average/Batch" << endl; outfile << "=======================================================================================================" << endl; for (int count = 0; count < ARRAY_SIZE; count++) { float avg = 0; if (ChocTypeArray[count] > 0) { avg = (float)ChocTypeArray[count] / (float)ChocPiecesArray[count]; } outfile << count + 1 << setw(35) << ChocTypeArray[count] << setw(23) << ChocPiecesArray[count] << setw(24) << avg << endl; } // Closes outout file outfile.close(); return 0; }
int main() { int ChocTypeArray[ARRAY_SIZE]; int ChocPiecesArray[ARRAY_SIZE];
//Initialize arrays for (int count = 0; count < ARRAY_SIZE; count++) { ChocTypeArray[count] = 0; ChocPiecesArray[count] = 0; }
//Try to find data file infile.open("Chocolates.txt"); if (!infile) { cout << "Failed to open Chocolates.txt. Make sure it is in the same directory as the program." << endl; return -1; }
//Read data until the end of file int _chocType, _chocPieces; while (!infile.eof()) { infile >> _chocType >> _chocPieces;
//Validates if chocType is between 1 and 25 if (_chocType < 1 || _chocType > ARRAY_SIZE) { continue; //skip } else { _chocType--; //adjust for array index ChocTypeArray[_chocType] = _chocPieces; ChocPiecesArray[_chocType]++; } }
//Print the report printReport(ChocTypeArray, ChocPiecesArray);
system("pause"); return 0; }
Here is the data sample file (first number represents the chocolate type and the second number represents the pieces for that batch)
12 1026 15 248 7 402 10 1076 16 948 14 1174 6 1137 8 719 9 952 14 1516 9 830 14 296 19 596 15 874 23 100 15 1063 16 867 6 87 11 1330 1 217 18 802 15 943 15 165 23 100 2 866 21 656 18 885 7 1221 9 1032 22 490 9 1328 12 584 11 561 14 1355 22 750 12 800 9 686 19 401 11 1121 16 389 3 664 8 676 12 692 1 844 1 994 1 847 24 1173 7 792 8 1076 19 613 14 1085 3 1107 12 891 3 1121 6 870 16 300 16 1092 24 969 12 515 6 403 11 885 7 839 14 328 1 1023 7 653 6 276 11 217 3 1288 6 1109 23 643 23 384 7 1213 6 556 4 1224 9 1271 15 1167 8 595 6 746 1 1284 18 1300 14 747 5 650 4 934 9 952 16 1408 24 1012 12 696 14 333 14 478 5 434 1 548 6 285 9 341 23 925 3 150 11 1166 5 963 8 1010 11 1272 1 587 21 866 12 152 11 1280 2 821 4 778 19 1087 15 690 11 1239 6 1267 4 361 12 704 3 560 16 1231 21 1198 6 697 3 696 6 632 16 1543 10 211 2 285 10 845 24 554 16 1105 6 1048 4 1050 16 552 3 1077 19 1333 19 669 8 1172 21 1217 2 826 18 995 7 1140 5 1084 7 1016 21 624 3 654 5 990 9 1115 23 991 3 324 8 959 16 1483 16 1036 9 694 4 817 24 247 6 477 12 1307 10 596 19 1008 1 843 12 1166 24 693 3 397 16 1000 19 368 9 1101 11 1270 15 172 8 839 12 760 6 1099 16 1119 1 673 10 742 2 858 11 1015 8 771 10 537 11 475 6 857 1 932 14 728 11 914 10 652 19 436 2 415 23 724 10 974 2 874 18 365 14 1158 1 986 7 283 21 870 10 708 2 546 7 919 15 429 9 607 19 591 16 938 2 662 6 1044 3 549 5 1012 19 1003 3 654 18 1436 1 738 14 695 14 655 10 266 11 510 6 1265 7 603 24 687 23 1014 9 557 1 677 16 1177 24 712 9 1275 14 1188 16 311 19 581 23 237 21 1228 23 717 24 729 10 511 7 584 23 1043 21 941 23 100 2 966 23 492 5 1312 1 968 18 699 2 249 24 1062 18 1015 2 924 16 952 14 591 4 899 5 692 11 1435 7 760 2 852 15 964 21 921 7 1131 4 919 6 870 4 778 2 1507 14 213 23 713 23 861 1 595 4 623 7 794 7 880 23 1371 9 455 19 1217 16 187 6 1219 10 1135 24 365 18 906 14 576 23 700 19 444 10 11 3 817 19 1394 10 948 7 666 24 883 6 1447 7 385 1 976 9 1037 14 373 2 526 19 1148 16 1185 24 1338 11 854 5 1004 15 1212 8 281 24 1316 6 15 18 1224 19 654 14 483 4 1428 8 769 19 473 14 1185 12 691 5 1252 1 425 6 459 18 1036 15 713 6 840 9 545 12 515 4 599 1 619 6 1162 9 561 5 1105 24 453 15 456 24 644 15 324 18 808 12 860 23 307 18 552 18 568 8 256 16 1458 23 562 19 660 19 983 9 970 7 1154 21 594 9 590 15 174 3 1048 9 1079 3 536 11 1301 16 508 1 810 21 647 10 802 11 50 24 921 18 195 4 273 5 564 11 314 21 710 7 267 15 224 18 215 9 975 9 328 9 475 23 470 12 649 7 782 16 765 16 1019 9 537 4 1089 21 221 15 372 7 706 19 379 10 1015 8 1244 23 443 1 121 6 732 10 718 23 1084 12 424 23 536 11 308 7 637 18 611 19 870 24 310 15 1145 9 1005 10 672 10 59 16 1235 19 764 18 430 12 1122 8 956 23 704 1 507 8 822 3 761 2 1084 11 1066 19 439 15 859 5 494 15 498 5 1092 15 1457 15 458 18 1133 23 44 10 1215 16 856 8 942 1 321 16 663 1 463 5 1324 6 676 7 858 23 536 2 476 24 358 21 481 5 917 10 1293 4 1176 9 768 10 742 10 1294 6 608 11 237 15 1038 11 330 2 1117 4 82 21 516 16 1222 8 1347 11 922 23 1126 4 868 18 296 24 412 18 407 23 1421 4 930 23 981 2 146 3 348 16 361 18 553 24 1046 2 557 6 396 23 104 3 1197 1 892 6 218 1 865 11 644 6 471 12 938 14 759 2 180 19 739 5 1276 19 1067 7 299 10 715 19 514 7 225 5 574 14 797 18 556 23 531 21 516 15 1463 4 1264 11 1006 12 605 6 592 4 759 6 628 16 1469 8 843 16 638 23 614 24 439 19 775 9 711 8 1304 24 749 16 1143 12 829 16 1063 9 581 8 1113 10 851 14 853 19 781 10 49 16 1035 19 264 18 490 12 922 8 956 23 704 1 507 8 822 3 761 2 1084 14 1221 6 772 7 911 7 545 8 724 10 1005 7 1133 2 189 24 1212 18 1118 21 241 15 1178 3 659 24 867 4 497 8 708 18 1308 11 411 7 500 15 538 8 366 1 1185 19 989 9 759 10 144 8 1210 15 1284 5 361 23 962 5 517 8 637 15 1239 11 992 7 394 2 725 24 114 19 1518 8 1127 12 415 1 1014 15 637 9 860 3 986 21 799 24 211 4 1212 5 1348 8 530 5 1362 9 1114 10 294 8 1414 15 1010 7 987 7 608 10 923 21 837 15 325 16 1127 10 1037 12 980 10 1160 6 331 1 840 18 673 3 561 9 921 24 752 23 1123 21 757 3 722 4 996 19 415 10 650 11 239 5 813 2 349 3 484 16 70 4 596 7 753 9 716 6 1022 4 351 11 185 2 403 12 570 24 905 4 50 9 211 14 737 8 587 5 532 1 544 19 21 5 1096 11 232 1 185 24 507 3 345 16 340 3 755 15 487 7 971 2 1309 11 500 15 968 10 739 8 755 12 60 3 770 18 601 9 284 21 55 15 1144 19 1524 6 1214 7 221 19 391 23 422 9 476 1 1054 1 967 5 1149 1 794 23 1014 4 605 23 860 4 311 14 443 6 659 19 85 1 501 23 346 1 268 8 941 12 918 23 779 18 791 8 561 7 1051 24 554 8 444 14 502 12 1043 9 1080 6 771 1 1274 8 737 19 197 3 703 6 748 10 1029 14 1095 10 1141 9 924 5 962 3 192 10 963 12 521 1 1195 4 529 3 1330 7 900 21 1118 18 1118 5 1060 15 459 18 1107 24 788 16 689 5 392 18 1106 15 848 18 531 12 242 12 669 18 962 14 658 9 799 8 712 21 873 10 1191 11 577 5 499 24 817 23 569 1 529 1 1152 3 813 10 901 21 739 3 641 23 581 10 768 6 810 2 1457 8 698 14 1351 11 1081 21 589 9 848 16 1043 8 710 15 904 11 1171 10 62 16 1097 14 691 3 1183 11 1286 7 606 23 89 7 859 14 481 12 839 2 550 3 1457 3 512 12 465 14 657 21 1149 10 1340 3 765 2 948 9 395 16 662 5 961 23 1389 23 1214 5 890 8 134 22 666 22 777 23 100 22 555 3 1295 11 307 11 605 15 1064 6 1214 1 64 8 907 18 1133 9 636 3 1134 16 1101 6 313 7 791 7 1130 1 1221 21 332 24 697 6 807 2 36 2 747 16 1219 3 859 18 639 18 312 7 1079 10 1074 5 678 18 59 1 325 21 543
Step by Step Solution
There are 3 Steps involved in it
Step: 1
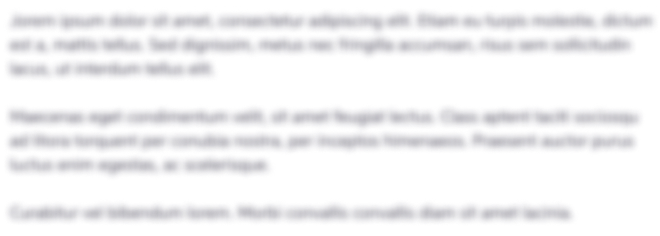
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started