Question
I am trying to get this C program to work and it is not at the moment. Can someone help me out. Write a C
I am trying to get this C program to work and it is not at the moment. Can someone help me out.
Write a C program using multiple functions that will calculate the gross pay for a set of employees.
See the last lecture note this week for a template you can use if you feel you need something to start with. However, feel free to implement this assignment that way you see fit, the template is just there if you need it. There are multiple ways to effectively implement this assignment.
The program determines the overtime hours (anything over 40 hours), the gross pay and then outputs a table in the following format shown below. Column alignment, leading zeros in Clock number, and zero suppression in float fields is important. Use 1.5 as the overtime pay factor.
#include
/* constants */
#define NUM_EMPL 5
#define OVERTIME_RATE 1.5f
#define STD_WORK_WEEK 40.0f
/* function prototypes */
float getHours (long int clockNumber);
void printHeader (void);
void printEmp (long int clockNumber, float wageRate, float hours,
float overtimeHrs, float grossPay);
/* TODO: Add other function prototypes here as needed */
int main()
{
/* Variable Declarations */
long int clockNumber[NUM_EMPL] = {98401,526488,765349,34645,127615}; /* ID */
float grossPay[NUM_EMPL]; /* gross pay */
float hours[NUM_EMPL]; /* hours worked in a given week */
int i; /* loop and array index */
float overtimeHrs[NUM_EMPL]; /* overtime hours */
float wageRate[NUM_EMPL] = {10.60,9.75,10.50,12.25,8.35}; /* hourly wage rate */
/* process each employee */
for (i = 0; i < NUM_EMPL; ++i)
{
/* Read in the hours for an employee */
hours[i] = getHours (clockNumber[i]);
/* TODO: Function call to calculate overtime */
/* TODO: Function call to calculate gross pay */
}
/* print the header info */
printHeader();
/* print out each employee */
for (i = 0; i < NUM_EMPL; ++i)
{
/* Print all the employees - call by reference */
printEmp (clockNumber[i], wageRate[i], hours[i],
overtimeHrs[i], grossPay[i]);
} /* for */
return (0);
}
//**************************************************************
// Function: getHours
//
// Purpose: Obtains input from user, the number of hours worked
// per employee and stores the result in a local variable
// that is passed back to the calling function.
//
// Parameters: clockNumber - The unique employee ID
//
// Returns: hoursWorked - hours worked in a given week
//
//**************************************************************
float getHours (long int clockNumber)
{
float hoursWorked; /* hours worked in a given week */
/* Read in hours for employee */
printf(" Enter hours worked by emp # %06li: ", clockNumber);
scanf ("%f", &hoursWorked);
/* return hours back to the calling function */
return (hoursWorked);
}
//**************************************************************
// Function: printHeader
//
// Purpose: Prints the initial table header information.
//
// Parameters: none
//
// Returns: void
//
//**************************************************************
void printHeader (void)
{
printf (" *** Pay Calculator *** ");
/* print the table header */
printf(" Clock# Wage Hours OT Gross ");
printf("------------------------------------------------ ");
}
//*************************************************************
// Function: printEmp
//
// Purpose: Prints out all the information for an employee
// in a nice and orderly table format.
//
// Parameters:
//
// clockNumber - unique employee ID
// wageRate - hourly wage rate
// hours - Hours worked for the week
// overtimeHrs - overtime hours worked in a week
// grossPay - gross pay for the week
//
// Returns: void
//
//**************************************************************
void printEmp (long int clockNumber, float wageRate, float hours,
float overtimeHrs, float grossPay)
{
/* print the employee */
/* TODO: add code to print out each employee one at a time */
}
/* TODO: Add other functions here as needed */
/* ... remember your comment block headers for each function */
Step by Step Solution
There are 3 Steps involved in it
Step: 1
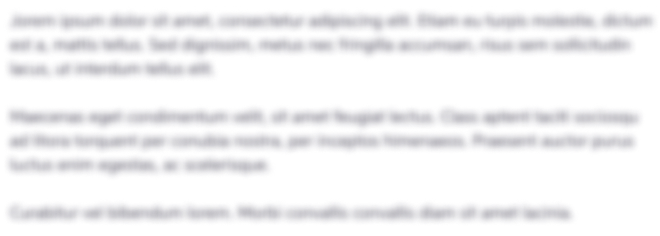
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started