Question
I am using github to do it. My task is to render and to get it to show in the HTML file. (cube.html) I need
I am using github to do it. My task is to render and to get it to show in the HTML file. (cube.html)
I need it converted into HTML
Consider modeling a cube. We want our cube to have the following properties: centered at the origin (0, 0, 0) the length of any edge to be 1.0 units That information should suggest what the vertex positions of the cube should be. Next, you'll need to determine the faces of the cube. A recommendation would be to model each face as two independent triangles using indexed rendering. That specification should tell you how many indices are required in your index list. For each triangle, make sure the vertex winding (the right-hand rule discussion from class). The deliverables for this project will be: the list of vertex positions that you would use to initialize the this.positions.values array in an object. the list of indices that would render the triangles composing the cubes that you would use to initialize the values in this.indices.values. (A test program framework will be uploaded in the next day to allow you to concentrate on the modeling aspect, and not be concerned with the rendering at the moment. More details to follow soon). This assignment was locked Mar 10 at 11:59pm. Now that you've modeled your cube, let's render it and make sure you have the triangle face winding correct (recall, faces are front facing if the order of their vertex positions are counter-clockwise). As discussed, here's a framework for testing and submitting your modeled cube. First, here's an implementation of a Cube class that you should add to your Common directory. In this file, add in your vertex positions and the index list to render the cube as independent triangles. There are two comments in the file that begin with // Insert your ... hereto show where you should add your code. Cone.jsView in a new window Next, create a directory (if you haven't already) for Assignment-4, and place the following two files in that directory. cube.htmlView in a new window cube.jsView in a new window You do not need to modify these files; they're complete, including shaders and handling uniform variables. You should just be able to open cube.html in your web browser and see your handiwork. To help you know that you have the vertex winding right, the fragment shader uses a new WebGL variable named gl_FrontFacing which indicated if the primitive you've drawn is front facing or not. Front facing primitives will be rendered in magenta, while back-facing ones will be rendered in red. You don't want to see any red. Some things to look at these programs for, since you'll need to do this for the next assignments: review how we schedule additional calls to render() to do animation check out how we increment a motion variable and use it to do animation (this assignment doesn't use a matrix stack, since there's only a single object, so don't be surprised).
Once you're satisfied with your cube implementation, commit it to your Git repository, and submit a screen shot to let me know you're done. const DefaultNumSides = 8; function Cone( gl, numSides, vertexShaderId, fragmentShaderId ) { // Initialize the shader pipeline for this object using either shader ids // declared in the application's HTML header, or use the default names. // var vertShdr = vertexShaderId || "Cone-vertex-shader"; var fragShdr = fragmentShaderId || "Cone-fragment-shader"; this.program = initShaders(gl, vertShdr, fragShdr); if ( this.program
First, add the apex vertex onto the index list // indices.push(n + 1); // Next, we need to append the rest of the vertices for the permieter of the disk. // However, the cone's perimeter vertices need to be reversed since it's effectively a // reflection of the bottom disk. // indices = indices.concat( indices.slice(1,n+2).reverse() ); this.positions.buffer = gl.createBuffer(); gl.bindBuffer( gl.ARRAY_BUFFER, this.positions.buffer ); gl.bufferData( gl.ARRAY_BUFFER, new Float32Array(positions), gl.STATIC_DRAW ); this.indices.buffer = gl.createBuffer(); gl.bindBuffer( gl.ELEMENT_ARRAY_BUFFER, this.indices.buffer ); gl.bufferData( gl.ELEMENT_ARRAY_BUFFER, new Uint16Array(indices), gl.STATIC_DRAW ); this.positions.attributeLoc = gl.getAttribLocation( this.program, "vPosition" ); gl.enableVertexAttribArray( this.positions.attributeLoc ); this.render = function () { gl.useProgram( this.program ); gl.bindBuffer( gl.ARRAY_BUFFER, this.positions.buffer ); gl.vertexAttribPointer( this.positions.attributeLoc, this.positions.numComponents, gl.FLOAT, gl.FALSE, 0, 0 ); gl.bindBuffer( gl.ELEMENT_ARRAY_BUFFER, this.indices.buffer ); // Draw the cone's base // gl.drawElements( gl.POINTS, this.indices.count, gl.UNSIGNED_SHORT, 0 ); // Draw the cone's top // var offset = this.indices.count * 2 /* sizeof(UNSIGNED_INT) */; gl.drawElements( gl.POINTS, this.indices.count, gl.UNSIGNED_SHORT, offset ); } }; var cube = undefined; var gl = undefined; var angle = 0; function init() { var canvas = document.getElementById( "webgl-canvas" ); gl = WebGLUtils.setupWebGL( canvas ); if ( !gl ) { alert("Unable to setup WebGL"); return; } gl.clearColor( 0.8, 0.8, 0.8, 1.0 ); gl.enable( gl.DEPTH_TEST ); cube = new Cube(); render(); } function render() { gl.clear( gl.COLOR_BUFFER_BIT | gl.DEPTH_BUFFER_BIT ); angle += 2.0; // degrees cube.MV = rotate( angle, [1, 1, 0] ); cube.render(); requestAnimationFrame( render ); // schedule another call to render() } window.onload = init; Show original message
How did you get this step by step below?
Step by Step Solution
There are 3 Steps involved in it
Step: 1
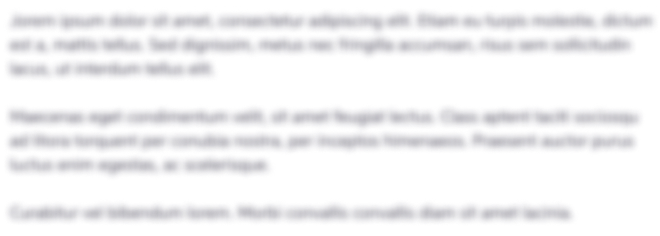
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started