Question
I am working in visual studios and need to write this program in C++. The game of matchsticks is a two-player game played with 21
I am working in visual studios and need to write this program in C++.
The game of matchsticks is a two-player game played with 21 matchsticks (or any other object). Players take turns removing matchsticks from a centralized pile. On any given turn, a player can remove 1, 2, or 3 matchsticks. Turns pass from player to player. The winner of the game is the player who takes the last matchstick. It is against the rules to put matchsticks back into a pile, or skip a turn.
Hint: If you get to go first and know the "algorithm" you can always win. To "see" the algorithm look carefully at the number of matchsticks that remain after each move.
The Secret: Whenever there is a chance, adjust the remaining number of sticks so that number is evenly divisible by 4. If you do, you will win. If you don't, the opponent's next move will use this strategy, and then you cannot win.
Program:
For this programming assignment, you will create a computerized game of matchsticks that allows for a human player and a simulated computer player. The simulated computer play should exhibit perfect play. Your game should have the following options.
1) Allow a choice of which player goes first.
2) Allow changing of the number of matchsticks with which the players begin the game from the default value of 21.
Requirements:
"Functionalize" your program as much as possible, that is, decompose the program into reasonable modules (functions). Main should pretty much consist of variable declarations, overall control structure, and function invocations. Anything substantial should be relegated to a function, either void or non-void, as appropriate. Remember to comment your functions to indicate their purpose within the program.
Verify that the user is making a legal move. In other words, your program should check that the number of matchsticks requested by the user is between 1 and 3 and does not exceed the number of matchsticks remaining.
Use at least one void function that passes back more than one parameter by reference in the construction of your program.
The game should allow the player to start a new game after the game is complete. At the end of each game, the program should output the total number of games won so far by the user and by the computer along with the players winning percentage.
You may not use any global variables in the development of your game.
Test your program thoroughly!
Bonus:
Frequently, a computerized opponent that plays optimally is not a lot of fun for the human player. Modify your program so that there is an additional AI that plays randomly. Incorporate a menu to allow the user to select the level of difficulty.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
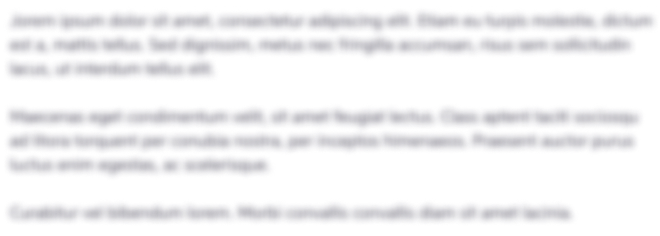
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started