Answered step by step
Verified Expert Solution
Question
1 Approved Answer
I am working on a coding project, and I have successfully passed all the tests, however, when I attempted to submit my project, 3 new
I am working on a coding project, and I have successfully passed all the tests, however, when I attempted to submit my project, 3 new tests were added and I can't seem to pass them. There is no description for the test because we are to figure that out ourselves, however, they do have names on the submission website:
FAILED TESTS
testPriorGuesses1K2 (0.0/10.0)
testPriorGuesses1K5 (0.0/10.0)
testPriorGuesses1K9 (0.0/10.0)
Please attempt to fix the code so that it passes those 3 tests.
/**
* * An Array-based implementation of the Guess-A-Number game * */ public class ArrayGame { // stores the next number to guess private int guess; // TODO: declare additional data members, such as arrays that store // prior guesses, eliminated candidates etc. // NOTE: only primitive type arrays are allowed, such as int[], boolean[] etc. // You MAY NOT use any Collection type (such as ArrayList) provided by Java. private int permutations = 9*10*10*10; private boolean[] guessArray; private int guessCount; private boolean gameOver; private int highMatches; private int knownDigits; private int lastGuess; /******************************************************** * NOTE: you are allowed to add new methods if necessary, * but DO NOT remove any provided method, otherwise your * code will fail the JUnit tests! * Also DO NOT create any new Java files, as they will * be ignored by the autograder! *******************************************************/ // ArrayGame constructor method public ArrayGame() { lastGuess = 0; highMatches = 0; knownDigits = 0; guess = 1000; gameOver = false; guessCount = 0; guessArray = new boolean[permutations]; for (int i=0; i < permutations; i++) guessArray[i] = false; } // Resets data members and game state so we can play again public void reset() { lastGuess = 0; knownDigits = 0; highMatches = 0; guess = 1000; gameOver = false; guessCount = 0; guessArray = new boolean[permutations]; for (int i=0; i < permutations; i++) guessArray[i] = false; } // Returns true if n is a prior guess; false otherwise. public boolean isPriorGuess(int n) { return guessArray[n-1000]; } // Returns the number of guesses so far. public int numGuesses() { return guessCount; } /** * Returns the number of matches between integers a and b. * You can assume that both are 4-digits long (i.e. between 1000 and 9999). * The return value must be between 0 and 4. * * A match is the same digit at the same location. For example: * 1234 and 4321 have 0 match; * 1234 and 1114 have 2 matches (1 and 4); * 1000 and 9000 have 3 matches (three 0's). */ public static int numMatches(int a, int b) { // DO NOT remove the static qualifier int matchCount = 0; String aStr = Integer.toString(a); String bStr = Integer.toString(b); for (int i=0; i < 4; i++) matchCount += (aStr.charAt(i) == bStr.charAt(i)) ? 1 : 0; return matchCount; } /** * Returns true if the game is over; false otherwise. * The game is over if the number has been correctly guessed * or if all candidates have been eliminated. */ public boolean isOver() { return gameOver; } // Returns the guess number and adds it to the list of prior guesses. public int getGuess() { // TODO: add guess to the list of prior guesses. guessCount++; guessArray[guess-1000] = true; return guess; } /** * Updates guess based on the number of matches of the previous guess. * If nmatches is 4, the previous guess is correct and the game is over. * Check project description for implementation details. * * Returns true if the update has no error; false if all candidates * have been eliminated (indicating a state of error); */ public boolean updateGuess(int nmatches) { // Error if ((nmatches - highMatches) <= -2) return false; if (nmatches == 4) { // Done gameOver = true; return true; // Victory } highMatches = Integer.max(nmatches, highMatches); if (nmatches == 0) { // All Digits invalid guess += 1111; return true; } if (nmatches < highMatches) { knownDigits += 1; guess = lastGuess; } lastGuess = guess; guess += Math.pow(10, knownDigits); return true; } // Returns the list of guesses so far as an integer array. // The size of the array must be the number of prior guesses. // Returns null if there has been no prior guess public int[] priorGuesses() { if (guessCount == 0) return null; int guessHistory[] = new int[guessCount]; int index = 0; for (int i=0; i < guessCount; i++) { if (guessArray[i]) { guessHistory[index] = i + 1000; index++; } } return guessHistory; } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
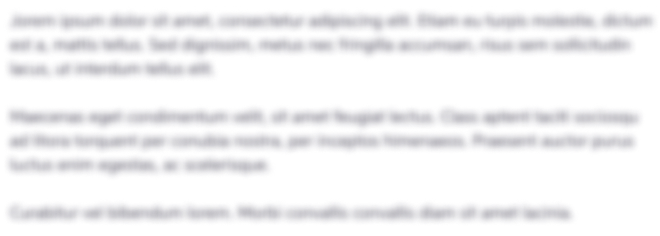
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started