Question
I am working on an visual basic program that takes a user input of scores that are entered and then adds them to an array
I am working on an visual basic program that takes a user input of scores that are entered and then adds them to an array list but i am trying to figure out how to remove the current count variable that i have declared and use the array list to retrieve the count from that. Another thing that i cant seem to figure out is to throw an error when the user enteres a number Less than 0. Finally when the user clicks display button they should see a sorted list in a messagebox
/////////////////////////////////////////This is what i have in the form designer
private void InitializeComponent() { this.lblScore = new System.Windows.Forms.Label(); this.lblScoreTotal = new System.Windows.Forms.Label(); this.lblScoreCount = new System.Windows.Forms.Label(); this.lblAverage = new System.Windows.Forms.Label(); this.btnDisplay = new System.Windows.Forms.Button(); this.btnClearScores = new System.Windows.Forms.Button(); this.btnExit = new System.Windows.Forms.Button(); this.btnAdd = new System.Windows.Forms.Button(); this.txtScore = new System.Windows.Forms.TextBox(); this.txtScoreTotal = new System.Windows.Forms.TextBox(); this.txtScoreCount = new System.Windows.Forms.TextBox(); this.txtAverage = new System.Windows.Forms.TextBox(); this.SuspendLayout(); // // lblScore // this.lblScore.AutoSize = true; this.lblScore.Location = new System.Drawing.Point(12, 27); this.lblScore.Name = "lblScore"; this.lblScore.Size = new System.Drawing.Size(38, 13); this.lblScore.TabIndex = 0; this.lblScore.Text = "Score:"; // // lblScoreTotal // this.lblScoreTotal.AutoSize = true; this.lblScoreTotal.Location = new System.Drawing.Point(12, 56); this.lblScoreTotal.Name = "lblScoreTotal"; this.lblScoreTotal.Size = new System.Drawing.Size(65, 13); this.lblScoreTotal.TabIndex = 1; this.lblScoreTotal.Text = "Score Total:"; // // lblScoreCount // this.lblScoreCount.AutoSize = true; this.lblScoreCount.Location = new System.Drawing.Point(12, 83); this.lblScoreCount.Name = "lblScoreCount"; this.lblScoreCount.Size = new System.Drawing.Size(69, 13); this.lblScoreCount.TabIndex = 2; this.lblScoreCount.Text = "Score Count:"; // // lblAverage // this.lblAverage.AutoSize = true; this.lblAverage.Location = new System.Drawing.Point(12, 111); this.lblAverage.Name = "lblAverage"; this.lblAverage.Size = new System.Drawing.Size(50, 13); this.lblAverage.TabIndex = 3; this.lblAverage.Text = "Average:"; // // btnDisplay // this.btnDisplay.Location = new System.Drawing.Point(29, 148); this.btnDisplay.Name = "btnDisplay"; this.btnDisplay.Size = new System.Drawing.Size(91, 23); this.btnDisplay.TabIndex = 2; this.btnDisplay.Text = "&Display Scores"; this.btnDisplay.UseVisualStyleBackColor = true; this.btnDisplay.Click += new System.EventHandler(this.btnDisplay_Click); // // btnClearScores // this.btnClearScores.Location = new System.Drawing.Point(136, 148); this.btnClearScores.Name = "btnClearScores"; this.btnClearScores.Size = new System.Drawing.Size(91, 23); this.btnClearScores.TabIndex = 3; this.btnClearScores.Text = "&Clear Scores"; this.btnClearScores.UseVisualStyleBackColor = true; this.btnClearScores.Click += new System.EventHandler(this.btnClearScores_Click); // // btnExit // this.btnExit.DialogResult = System.Windows.Forms.DialogResult.Cancel; this.btnExit.Location = new System.Drawing.Point(152, 177); this.btnExit.Name = "btnExit"; this.btnExit.Size = new System.Drawing.Size(75, 23); this.btnExit.TabIndex = 4; this.btnExit.Text = "E&xit"; this.btnExit.UseVisualStyleBackColor = true; this.btnExit.Click += new System.EventHandler(this.btnExit_Click); // // btnAdd // this.btnAdd.Location = new System.Drawing.Point(159, 22); this.btnAdd.Name = "btnAdd"; this.btnAdd.Size = new System.Drawing.Size(68, 23); this.btnAdd.TabIndex = 1; this.btnAdd.Text = "&Add"; this.btnAdd.UseVisualStyleBackColor = true; this.btnAdd.Click += new System.EventHandler(this.btnAdd_Click); // // txtScore // this.txtScore.Location = new System.Drawing.Point(90, 24); this.txtScore.Name = "txtScore"; this.txtScore.Size = new System.Drawing.Size(63, 20); this.txtScore.TabIndex = 0; // // txtScoreTotal // this.txtScoreTotal.Location = new System.Drawing.Point(90, 53); this.txtScoreTotal.Name = "txtScoreTotal"; this.txtScoreTotal.ReadOnly = true; this.txtScoreTotal.Size = new System.Drawing.Size(63, 20); this.txtScoreTotal.TabIndex = 9; // // txtScoreCount // this.txtScoreCount.Location = new System.Drawing.Point(90, 80); this.txtScoreCount.Name = "txtScoreCount"; this.txtScoreCount.ReadOnly = true; this.txtScoreCount.Size = new System.Drawing.Size(63, 20); this.txtScoreCount.TabIndex = 10; // // txtAverage // this.txtAverage.Location = new System.Drawing.Point(90, 108); this.txtAverage.Name = "txtAverage"; this.txtAverage.ReadOnly = true; this.txtAverage.Size = new System.Drawing.Size(63, 20); this.txtAverage.TabIndex = 11; // // frmScoreCalculatorList // this.AcceptButton = this.btnAdd; this.AutoScaleDimensions = new System.Drawing.SizeF(6F, 13F); this.AutoScaleMode = System.Windows.Forms.AutoScaleMode.Font; this.CancelButton = this.btnExit; this.ClientSize = new System.Drawing.Size(244, 223); this.Controls.Add(this.txtAverage); this.Controls.Add(this.txtScoreCount); this.Controls.Add(this.txtScoreTotal); this.Controls.Add(this.txtScore); this.Controls.Add(this.btnAdd); this.Controls.Add(this.btnExit); this.Controls.Add(this.btnClearScores); this.Controls.Add(this.btnDisplay); this.Controls.Add(this.lblAverage); this.Controls.Add(this.lblScoreCount); this.Controls.Add(this.lblScoreTotal); this.Controls.Add(this.lblScore); this.Name = "frmScoreCalculatorList"; this.StartPosition = System.Windows.Forms.FormStartPosition.CenterScreen; this.Text = "Score Calculator"; this.ResumeLayout(false); this.PerformLayout();
}
/////////////////////////////////////////////////This is the code that i have so far
public partial class frmScoreCalculatorList : Form { public frmScoreCalculatorList() { InitializeComponent(); } int total = 0; int count = 0; List
private void btnAdd_Click(object sender, EventArgs e) { int score = Convert.ToInt32(txtScore.Text); myData.Add(score); total += score; count += 1; int average = total / count; txtScoreTotal.Text = total.ToString(); txtScoreCount.Text = count.ToString(); txtAverage.Text = average.ToString(); txtScore.Focus(); }
private void btnDisplay_Click(object sender, EventArgs e) { myData.Sort(); string scoresString = ""; foreach (int i in myData) if (i != 0) { scoresString += i.ToString() + " "; } MessageBox.Show(scoresString, "Sorted Scores"); txtScore.Focus(); }
private void btnClearScores_Click(object sender, EventArgs e) { total = 0; txtScore.Text = ""; txtScoreTotal.Text = ""; txtScoreCount.Text = ""; txtAverage.Text = ""; txtScore.Focus(); myData = new List
private void btnExit_Click(object sender, EventArgs e) { this.Close(); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
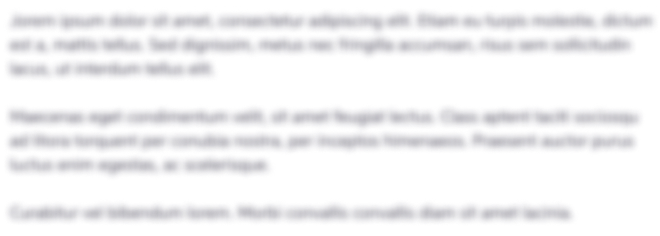
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started