Question
I am working on this code and keep getting errors like ERROR: Syntax error: Encountered at line 1, column 169. and ERROR: Table/View
I am working on this code and keep getting errors like
"ERROR: Syntax error: Encountered "
and
"ERROR: Table/View 'STUDENT' does not exist. ERROR: Table/View 'STUDENT' does not exist. ERROR: Table/View 'STUDENT' does not exist."
I do not know why this isn't working. Here is my code:
DTCCDatabase.java
import java.sql.Connection; import java.sql.DriverManager; import java.sql.SQLException; import java.sql.Statement;
/** * This program creates the CoffeeDB database. */ public class DTCCDatabase { public static void main(String[] args) { // Create a named constant for the URL. // NOTE: This value is specific for Java DB. final String DTCC_URL = "jdbc:derby:DTCCDatabase;create=true";
try { // Create a connection to the database. Connection conn = DriverManager.getConnection(DTCC_URL);
// If the DB already exists, drop the tables. dropTables(conn);
// Build the Coffee table and insert records. buildStudentTable(conn);
// Close the connection. conn.close(); System.out.println("DB creation success!"); } catch (Exception ex) { System.out.println("ERROR: " + ex.getMessage()); } } // end main /** * The buildCoffeeTable method creates the * Coffee table and adds some rows to it. */ public static void buildStudentTable(Connection conn) { try { // Get a Statement object. Statement stmt = conn.createStatement();
// Create the table. stmt.execute( "CREATE TABLE STUDENTS (Student_ID CHAR(25) NOT NULL PRIMARY KEY, " + "LastName CHAR(20) NOT NULL," + "FirstName CHAR(15) NOT NULL," + "PlanOfStudy CHAR(25) NOT NULL," + "GPA Double NOT NULL" );
// Insert row #1. stmt.execute( "INSERT INTO STUDENT VALUES (899090111, 'Rothlisberger', 'Ben', 'CIT', 3.7)" );
// Insert row #2. stmt.execute( "INSERT INTO STUDENT VALUES (129020202, 'Manning', 'Peyton', 'Computer Programming', 3.8)" );
// Insert row #3. stmt.execute( "INSERT INTO STUDENT VALUES (890101030, 'Brady', 'Tom', 'Accounting', 3.4 )" ); stmt.execute( "INSERT INTO STUDENT VALUES (980191919, 'Rodgers', 'Aaron', 'Networking', 3.2 )" ); stmt.execute( "INSERT INTO STUDENT VALUES (807223230, 'Manning', 'Eli', 'Securities', 3.7 )" );
System.out.println("Student table created."); } catch (SQLException ex) { System.out.println("ERROR: " + ex.getMessage()); } } // end build /** * The dropTables method drops any existing * in case the database already exists. */ public static void dropTables(Connection conn) { System.out.println("Checking for existing table.");
try { // Get a Statement object. Statement stmt = conn.createStatement();;
try { // Drop the Coffee table. stmt.execute("DROP TABLE Coffee"); System.out.println("Coffee table dropped."); } catch(SQLException ex) { // No need to report an error. // The table simply did not exist. } } catch(SQLException ex) { System.out.println("ERROR: " + ex.getMessage()); ex.printStackTrace(); } } // end drop tables } // end class
DTCCViewer.java
// Needed for JDBC classes import java.sql.Connection; import java.sql.DriverManager; import java.sql.ResultSet; import java.sql.ResultSetMetaData; import java.sql.SQLException; import java.sql.Statement;
public class DTCCViewer {
public static void main(String[] args) { // Create a named constant for the URL. // NOTE: This value is specific for Java DB. final String DTCC_URL = "jdbc:derby:DTCCDatabase;create=true";
try { // Create a connection to the database. Connection conn = DriverManager.getConnection(DTCC_URL);
// view data viewStudentTable(conn); // modify record modStudentTable(conn); viewStudentTable(conn); // Close the connection. conn.close();
} catch (Exception ex) { System.out.println("ERROR: " + ex.getMessage()); }
} // end main
public static void viewStudentTable(Connection conn) { //create var for result set ResultSet resultset = null;
try { // Get a Statement object. Statement stmt = conn.createStatement();
// View the table. resultset = stmt.executeQuery("SELECT * FROM Student");
// process results ResultSetMetaData metaData = resultset.getMetaData(); System.out.println("Data from Student Table:");
int numberOfColumns = metaData.getColumnCount(); // for loop to field names for (int i = 1; i <= numberOfColumns; i++){ System.out.printf("%s\t", metaData.getColumnName(i)); } System.out.println(); // while loop to display data while (resultset.next()){ for (int i = 1; i <= numberOfColumns; i++){ System.out.printf("%s\t", resultset.getObject(i)); } System.out.println(); }
} catch (SQLException ex) { System.out.println("ERROR: " + ex.getMessage()); } }
public static void modStudentTable(Connection conn) { //create var for result set ResultSet resultset = null;
try { // Get a Statement object. Statement stmt = conn.createStatement();
// update record stmt.executeUpdate("UPDATE Student SET PlanOfStudy = 'WebTechnologies' WHERE FirstName = 'Tom'"); System.out.println("Record updated");
} catch (SQLException ex) { System.out.println("ERROR: " + ex.getMessage()); } }
} // end class
Please help!
Step by Step Solution
There are 3 Steps involved in it
Step: 1
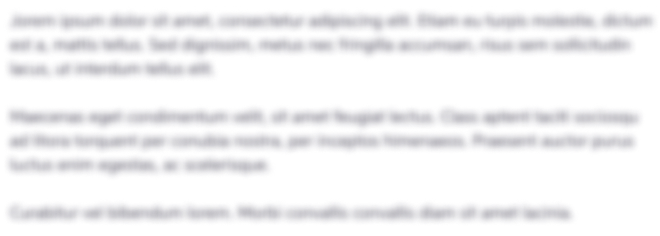
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started