Question
I am writing a c++ program that has the user input a city or partial city name and then the program will search through a
I am writing a c++ program that has the user input a city or partial city name and then the program will search through a text file, personnel_addresses.txt, containing a list of names and the city that person lives and the program will store all the names of people that live in a city that contains the string entered by the user in a vector. So if the user enters the city name "Wax" the program will read through the text file and store all the names of people that live in that city in a vector. Then it will loop through the the vector and search for each name in another text file, personnel_salaries.txt, and it will then output the name of the person and their salary separated by a colon. Program works great! No bugs or anything but it is just really inefficient right now and I was just wanting to see if there was a more efficient way to write this program so it gets all the data faster.
# include # include # include # include using namespace std; int main() { fstream name_cities; fstream salaries; string line; string name; string sal; string user_input; string city; vector name_vector; ofstream data; string person; string income; data.open("data.txt"); name_cities.open("personnel_addresses.txt"); salaries.open("personnel_salaries.txt"); cout << "Enter a string for a city:" << endl; cin >> user_input; if (name_cities) { while (getline(name_cities, line)) { city = (line.substr(line.find("|") + 1, line.back())); if (city.find(user_input) != std::string::npos) { name = line.substr(0, line.find("|")); name_vector.push_back(name); } } } for (vector::iterator t = name_vector.begin(); t != name_vector.end(); ++t) { while (getline(salaries, sal)) { person = sal.substr(0, sal.find("|"));
if (person == *t) { income = (sal.substr(sal.find("|") + 1, sal.back())); cout << person << ":" << income << endl; data << person << ":" << income << endl; } } salaries.clear(); salaries.seekg(0, ios::beg); } data.close(); name_cities.close(); salaries.close();
system("pause"); return 0; }
Portion of text files:
personnel_addresses:
apheth Edwards|Albany Evan Taylor|Wax Olivia Grimme|Wax Alfonso McNeil|Somerset Allyson Schroeder|Washington April Tomes|Lancaster Laura Hancock|Louisville Robert Joyce|Remington HamedHaribHamed Yates|Somerset Robin Snoke|Remington David Bash|Versailles Boyko Michels|Washington
personnel_salaries:
Jessica Collett|59789 Mitchell Long|68985 Khumbo Benedict|53385 Adam Kandibanda|67671 Lloyd Wathen|98564 Daniel Towery|36588 Suzanne Siferd|97528 James Gudger|67113 Laken Ferguson|61260 Lauren Canter|57304 Jordan McMillan|67088 Angela Padgett|58771 Shilpi Caldwell|94080 Kathryn Kolles|73990
Step by Step Solution
There are 3 Steps involved in it
Step: 1
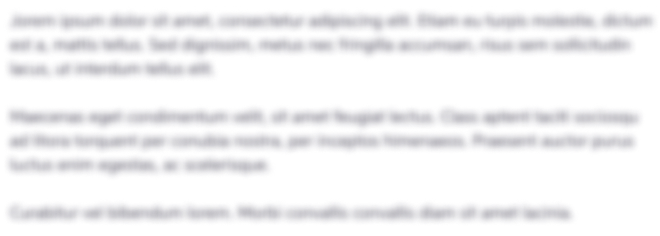
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started