Question
I am writing a Java method that accepts two integers. The first integer is the numeric value of a day of the year (1 -
I am writing a Java method that accepts two integers. The first integer is the numeric value of a day of the year (1 - 365). The second value is the year.
Without importing the Calendar class, how can I take the first integer and determine which month and which day in that month is being referenced?
For example:
If the integers given are: 107, 2018
The output should be: April 17, 2018 or 04/17/2018
This is how I've done it, but I'd like to know if there is a more efficient way:
public Date(int ddd, int yyyy){ int leap = leap(yyyy); int month = 0; int day = 0; if(ddd < 32){ month = 1; day = ddd; }//end jan if(ddd > 31 && ddd <= 59){ month = 2; day = ddd - 31; }// end feb if(ddd > 59 && ddd <= 90){ month = 3; day = ddd - 59; }//end of mar if(ddd > 90 && ddd <= 120 ){ month = 4; day = ddd - 90 - leap; }// end of apr if(ddd > 120 && ddd <= 151){ month = 5; day = ddd - 120 - leap; }// end of may if(ddd > 151 && ddd <= 181){ month = 6; day = ddd - 151 - leap; }//end of june if(ddd > 181 && ddd <= 212){ month = 7; day = ddd - 181 - leap; }//end of july if(ddd > 212 && ddd <= 243){ month = 8; day = ddd - 212 - leap; }//end of aug if(ddd > 243 && ddd <= 273){ month = 9; day = ddd - 243 - leap; }//end of sept if(ddd > 273 && ddd <= 304){ month = 10; day = ddd - 273 - leap; }//end of oct if(ddd > 304 && ddd <= 334){ month = 11; day = ddd - 304 - leap; }//end of nov if(ddd > 334){ month = 12; day = ddd - 334 - leap; }//end of dec dateString1 = month + "/" + day + "/" + yyyy; dateString2 = Months[month - 1]+" "+day+", "+yyyy; dateString3 = "The day entered day number " + ddd + " of the year " + yyyy;
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
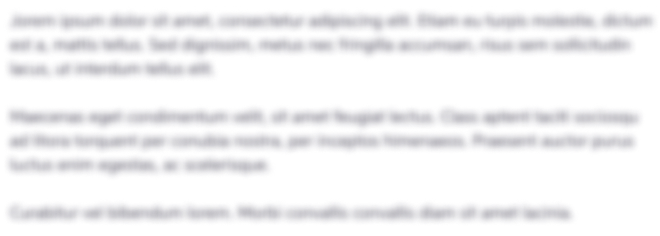
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started