Question
I am writing a program for a vending machine and I have all of the code. The only problem lies with the purchase method within
I am writing a program for a vending machine and I have all of the code. The only problem lies with the purchase method within the VendingMachine class.
Here is my code:
Main class (Ignore the code that is commented out):
public class Main {
/** * @param args the command line arguments */ public static void main(String[] args) throws FileNotFoundException { File f = new File("candy.txt"); Scanner sc = new Scanner(f);
String[] candyNames; int numNames = sc.nextInt(); sc.nextLine(); candyNames = new String[numNames]; for (int i = 0; i < numNames; i++) { candyNames[i] = sc.nextLine(); System.out.println("Adding " + candyNames[i]);
} // Random r = new Random(); // //System.out.println(candyNames[r.nextInt(numNames)]); // Scanner kb = new Scanner(System.in); // System.out.println("Press ENTER for candy, or type 'quit'"); // String command = kb.nextLine(); // while (!command.equals("quit")) { // Candy c = new Candy(candyNames[r.nextInt(numNames)], r.nextFloat(), r.nextInt(4)+1 ); // System.out.println(c); // command = kb.nextLine(); // // }
Scanner keyboard = new Scanner(System.in); System.out.println("How many shelves in the vending machine?"); int shelves = keyboard.nextInt(); System.out.println("How many slots on each shelf?"); int slotsPerShelf = keyboard.nextInt(); keyboard.nextLine(); VendingMachine vm = new VendingMachine(shelves, slotsPerShelf); vm.load(candyNames); vm.display(); System.out.println("What shelf do you want?"); int shelfSelection = keyboard.nextInt(); keyboard.nextLine(); System.out.println("What slot you want?"); int slotSelection = keyboard.nextInt(); keyboard.nextLine(); vm.purchase(shelfSelection, slotSelection); vm.display(); }
}
Candy Class:
public class Candy { private String name; private float price; private int ounces; private DecimalFormat df = new DecimalFormat("0.00"); public Candy(String n, float p, int o) { name = n; price = p; ounces = o; } public String toString() { return "Names: " + name + " Price: " + df.format(price) + " Ounces: " + ounces; } public String getName(){ return name; } }
VendingMachine Class:
public class VendingMachine {
private int shelves; private int slotsPerShelf; private Candy[][] slots;
public VendingMachine(int sh, int sl) { shelves = sh; slotsPerShelf = sl; slots = new Candy[shelves][slotsPerShelf];
}
public void load(String[] names) { Random r = new Random(); for (int i = 0; i < shelves; i++) { for (int j = 0; j < slotsPerShelf; j++) { slots[i][j] = new Candy(names[r.nextInt(names.length)], r.nextFloat(), r.nextInt(4) + 1); } } } public void display() { System.out.print(" "); for (int j = 0; j < slots[0].length; j++) { System.out.printf("|%25d|", j); } System.out.println(); for (int i = 0; i < shelves; i++) { System.out.printf("%2d", i);
for (int j = 0; j < slotsPerShelf; j++) { if (slots[i][j] == null) { System.out.printf("|%25s|", ""); } else { System.out.printf("|%25s|", slots[i][j].getName()); } } System.out.println(); } System.out.println(); } public void purchase (int r, int s) { if (r >= 0 && r < shelves && s >= 0 && s <= slotsPerShelf) { System.out.println("Your purchase: " + slots[r][s]); slots[r][s] = null; } else System.out.println("Invalid selection"); } }
The problem within the purchase method is that I need the program to print "Invalid Selection" if the user enters in a negative number for the number of shelves and slots within the machine, rather than the program just crashing. Thank you for your time.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
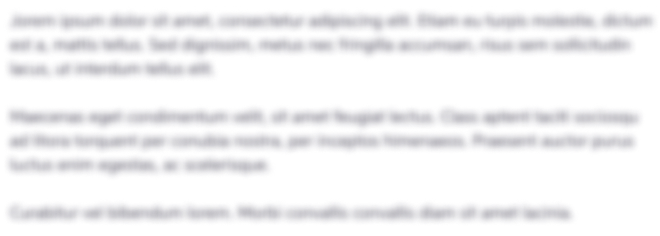
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started