Question
I am writing a project for a student management system and I'm stuck on 2 parts of it. The first part is when I add
I am writing a project for a student management system and I'm stuck on 2 parts of it. The first part is when I add multiple students into the arraylist and then go to list them in the text area it doesn't list all the students added. My second problem is saving to and loading from the csv file as neither seem to work. How do I go about fixing this?
package application;
import javafx.scene.control.Label;
import javafx.scene.control.TextField;
import javafx.scene.control.TextArea;
import java.io.IOException;
import application.student.StudentController;
import javafx.application.Application;
import javafx.application.Platform;
import javafx.event.ActionEvent;
import javafx.event.EventHandler;
import javafx.geometry.Insets;
import javafx.geometry.Pos;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.control.ButtonType;
import javafx.scene.layout.HBox;
import javafx.scene.layout.StackPane;
import javafx.scene.layout.VBox;
import javafx.stage.Stage;
import javafx.scene.control.DatePicker;
import javafx.scene.control.Alert;
import javafx.scene.control.Alert.AlertType;
public class Main extends Application {
private Label labelFName,labelLName,labelDOB,labelStudNo;
private TextField textFFName,textFLName,textFStudNo;
private TextArea textADisplay;
private Button btnAdd,btnRemove,btnList,btnLoad,btnSave,btnExit,btnYes, btnNo;
private static StudentController studCont = new StudentController();
public static void main(String[] args) {
launch(args);
}
@Override
public void start(Stage primaryStage) {
//Title
primaryStage.setTitle("MTU Student Record System");
//Label
labelFName = new Label("Forename: ");
labelLName = new Label("Surname: ");
labelDOB = new Label("Date Of Birth: ");
labelStudNo = new Label("Student Number: ");
//TextField
textFFName = new TextField();
textFLName = new TextField();
textFStudNo = new TextField();
//TextArea
textADisplay = new TextArea();
textADisplay.setEditable(false);
//DatePicker
DatePicker dateDOB = new DatePicker();
//ButtonAdd
btnAdd = new Button();
btnAdd.setText("Add");
btnAdd.setOnAction(new EventHandler() {
@Override
public void handle(ActionEvent event) {
textADisplay.clear();
// if(textFFName.getLength()<2 || textffname)
studCont.addStudentList(textFFName.getText(), textFLName.getText(), dateDOB.getValue(), textFStudNo.getText());
textADisplay.setText(textFFName.getText()+" "+textFLName.getText()+" has been added");
textFFName.clear();
textFLName.clear();
textFStudNo.clear();
dateDOB.setValue(null);
}
});
//ButtonRemove
btnRemove = new Button();
btnRemove.setText("Remove");
btnRemove.setOnAction(new EventHandler() {
@Override
public void handle(ActionEvent event) {
textADisplay.clear();
studCont.removeStudentList(textFFName.getText(), textFLName.getText(), dateDOB.getValue(), textFStudNo.getText());
textADisplay.setText(textFFName.getText()+" "+textFLName.getText()+" has been removed");
textFFName.clear();
textFLName.clear();
textFStudNo.clear();
dateDOB.setValue(null);
}
});
//ButtonList
btnList = new Button();
btnList.setText("List");
btnList.setOnAction(new EventHandler() {
@Override
public void handle(ActionEvent event) {
textADisplay.setText(studCont.getListStudent());
}
});
//ButtonLoad
btnLoad = new Button();
btnLoad.setText("Load");
btnLoad.setOnAction(new EventHandler() {
@Override
public void handle(ActionEvent event) {
try {
textADisplay.setText(studCont.loadStudentCSV());
} catch (IOException e) {
e.printStackTrace();
}
}
});
//ButtonSave
btnSave = new Button();
btnSave.setText("Save");
btnSave.setOnAction(new EventHandler() {
@Override
public void handle(ActionEvent event) {
try {
textADisplay.clear();
studCont.saveStudentCSV();
textADisplay.setText("Saved to File");
} catch (IOException e) {
textADisplay.clear();
e.printStackTrace();
textADisplay.setText("Couldn't Save to File");
}
}
});
//ButtonExit
btnExit = new Button();
btnExit.setText("Exit");
btnExit.setOnAction(new EventHandler() {
@Override
public void handle(ActionEvent event) {
Alert alert = new Alert(AlertType.CONFIRMATION);
alert.setTitle("Exit");
alert.setContentText("Do you want to save before exit?");
alert.showAndWait();
if(alert.getResult() == ButtonType.OK) {
try {
textADisplay.clear();
studCont.saveStudentCSV();
Platform.exit();
} catch (IOException e) {
textADisplay.clear();
e.printStackTrace();
textADisplay.setText("Couldn't Save to File");
}
}
else if(alert.getResult() == ButtonType.CANCEL) {
Platform.exit();
}
}
});
//HBox
HBox hbox1 = new HBox();
HBox hbox2 = new HBox();
HBox hbox3 = new HBox();
HBox hbox4 = new HBox();
HBox hbox5 = new HBox();
HBox hbox6 = new HBox();
HBox hbox7 = new HBox();
hbox1.getChildren().addAll(labelFName,textFFName);
hbox1.setSpacing(55);
hbox7.setAlignment(Pos.CENTER_LEFT);
hbox2.getChildren().addAll(labelLName,textFLName);
hbox2.setSpacing(61);
hbox7.setAlignment(Pos.CENTER_LEFT);
hbox3.getChildren().addAll(labelDOB,dateDOB);
hbox3.setSpacing(38);
hbox7.setAlignment(Pos.CENTER_LEFT);
hbox4.getChildren().addAll(labelStudNo,textFStudNo);
hbox4.setSpacing(19);
hbox7.setAlignment(Pos.CENTER_LEFT);
hbox5.getChildren().addAll(btnAdd,btnRemove,btnList);
hbox5.setSpacing(15);
hbox7.setAlignment(Pos.CENTER_LEFT);
hbox6.getChildren().addAll(textADisplay);
hbox7.setAlignment(Pos.CENTER);
hbox7.getChildren().addAll(btnLoad,btnSave,btnExit);
hbox7.setSpacing(15);
hbox7.setAlignment(Pos.CENTER_RIGHT);
//VBox
VBox vbox = new VBox(10);
vbox.setAlignment(Pos.CENTER);
vbox.getChildren().addAll(hbox1,hbox2,hbox3,hbox4,hbox5,hbox6,hbox7);
vbox.setPadding(new Insets(20, 20, 20, 20));
StackPane root = new StackPane();
root.getChildren().addAll(vbox);
primaryStage.setScene(new Scene(root, 500, 400));
primaryStage.show();
}
}
package application.student;
import java.time.LocalDate;
import java.util.ArrayList;
import java.util.Date;
import java.io.BufferedReader;
import java.io.File;
import java.io.FileNotFoundException;
import java.io.FileReader;
import java.io.FileWriter;
import java.io.IOException;
import java.io.PrintWriter;
public class StudentController {
private StudentList studCont;
public StudentController() {
studCont = new StudentList();
}
public void addStudentList(String fName,String lName, LocalDate dob, String studNo)
{
Student stud = new Student(fName, lName, dob, studNo);
studCont.addStudent(stud);
}
public String getListStudent()
{
String noStudents = "No Students have been added";
String allStudent="";
for (int i = 0;ireturn studCont.allStudent(i);
}
return noStudents;
}
public void removeStudentList(String fName,String lName, LocalDate dob, String studNo)
{
Student stud = new Student(fName, lName, dob, studNo);
studCont.removeStudent(stud);
}
public String saveStudentCSV() throws IOException {
String fileFound = "File Not Found";
FileWriter studentCSV = new FileWriter("StudentCSV.csv");
try {
studentCSV.append("Forename");
studentCSV.append("Surname");
studentCSV.append("Date of Birth");
studentCSV.append("Student Number");
studentCSV.append("");
for (int i = 0;i studentCSV.append(studCont.allStudent(i)+"");
}
} catch (FileNotFoundException e) {
return fileFound;
}
return fileFound;
}
public String loadStudentCSV() throws FileNotFoundException, IOException {
String line = "No input into file";
try (BufferedReader br = new BufferedReader(new FileReader("StudentCSV.csv"))) {
while ((line = br.readLine()) != null) {
return line;
}
}
return line;
}
}
package application.student;
import java.util.ArrayList;
public class StudentList {
private ArrayList studentList;
public StudentList() {
studentList = new ArrayList();
}
public void addStudent(Student student) {
studentList.add(student);
}
public void removeStudent(Student removeStudent) {
studentList.remove(removeStudent);
}
public int getSize (){
return studentList.size();
}
public String allStudent(int i) {
String allStudents ="0";
for(i=0;iallStudents = studentList.get(i).getFName()+" "+studentList.get(i).getLName()+"tt"+studentList.get(i).getDate()+"t"+studentList.get(i).getStudentNo();
return allStudents;
}
return allStudents;
}
}
package application.student;
import java.time.LocalDate;
public class Student {
/*==============================
* Instance Variables
* =============================
*/
private String fName;
private String lName;
private LocalDate dates;
private String studentNo;
/*==========================
* Constructors
==========================*/
public Student(String fName, String lName, LocalDate dob, String studentNo) {
this.fName = fName;
this.lName = lName;
this.dates = dob;
this.studentNo = studentNo;
}
public Student() {}
/*=========================
* Setters
* ========================*/
public void setFName(String fName) {
this.fName = fName;
}
public void setLName(String lName) {
this.lName = lName;
}
public void setdates(LocalDate dates) {
this.dates = dates;
}
public void setstudentNo(String studNo) {
this.studentNo = studNo;
}
/*=========================
* Getters
* ========================*/
public String getFName() {
return fName;
}
public String getLName() {
return lName;
}
public LocalDate getDate() {
return dates;
}
public String getStudentNo() {
return studentNo;
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
There are a few issues in your code that could be causing the problems you mentioned Lets go through them one by one and see how to fix them 1 Adding multiple students to the ArrayList It seems that y...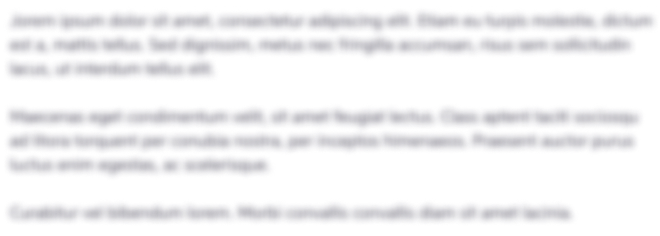
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started