I believe I have most of the code but I keep getting a "Exception in thread "main" java.lang.NumberFormatException: For input string: "Starting balance"" when I try to run the program.
Below is the question, code, and pictures:
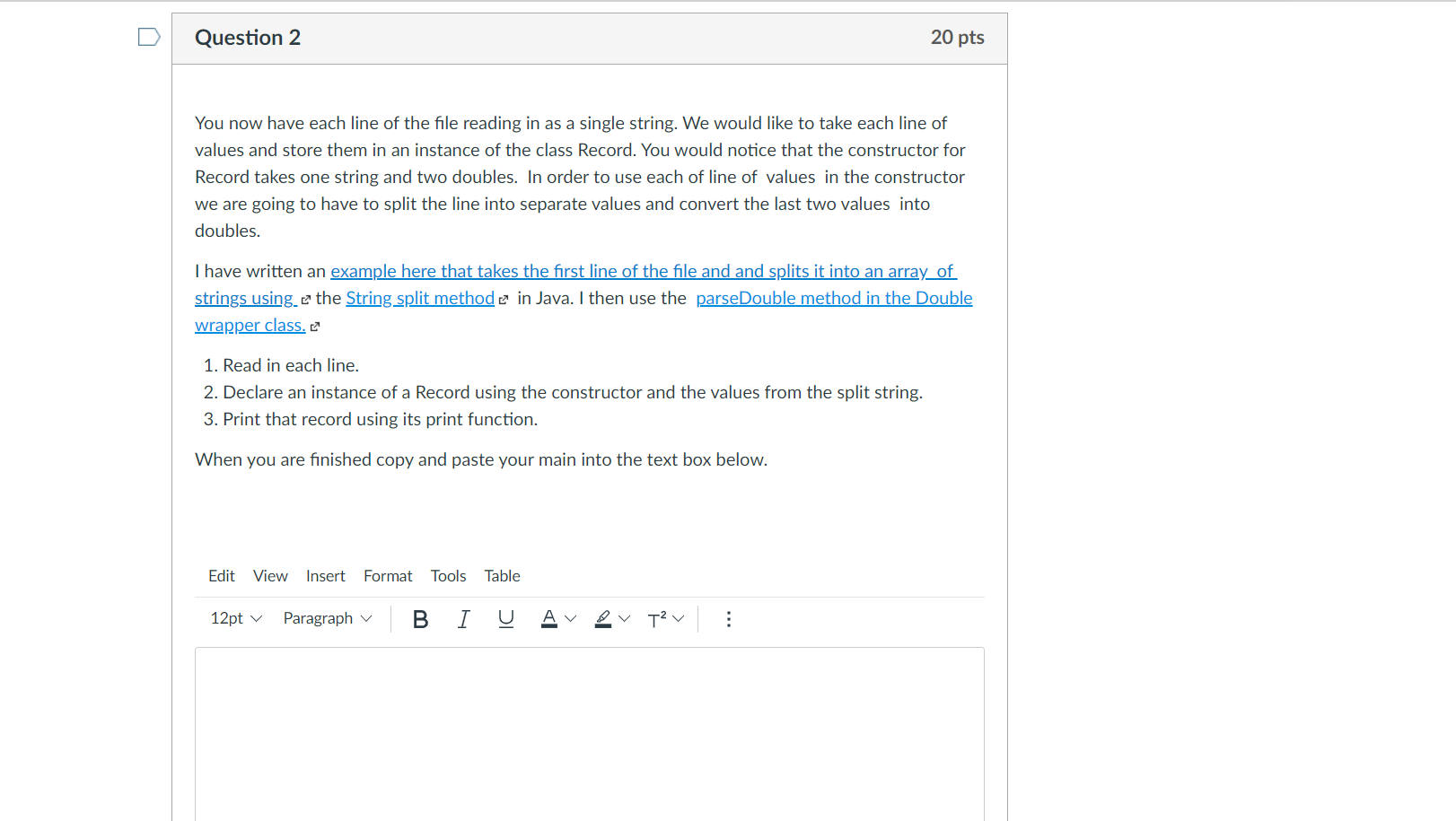
Main.java
class Main { public static void main(String[] args) { //Here is a string String data = "Jan,1137,1479"; //Using the split String function to split into substrings that are seperated by , String values[] = data.split(","); // Split returns an Array of substrings for(String item: values) // For each string item in values array System.out.print(item + " "); // print the item followed by a space System.out.println();
//Convert strings stored in second and third positions of values array to doubles double startingBalance = Double.parseDouble(values[1]); double endingBalance = Double.parseDouble(values[2]); System.out.println(startingBalance + " " + endingBalance); } }
Record.java
import java.text.DecimalFormat;
public class Record{
private String month;
private double startingBalance;
private double endingBalance;
private double amountSaved;
//Declare constructors
public Record(String monthVar, double startingBalanceVar, double endingBalanceVar)
{
//ASSIGN PARAMETERS OF CONSTRUCTOR TO ATTRIBUTES OF THE CLASS
this.month = monthVar;
this.startingBalance = startingBalanceVar;
this.endingBalance = endingBalanceVar;
this.amountSaved = monthSaving(); //Amount saved is calculated
}
//Declare setters
public void setMonth(String monthVar){
this.month = monthVar;
}
public void setStartingBalance(double startingBalanceVar){
this.startingBalance = startingBalanceVar;
}
public void setEndingBalance(double endingBalanceVar){
this.endingBalance = endingBalanceVar;
}
public void setAmountSaved(){
amountSaved = monthSaving();
}
//Declare Getters
public String getMonth(){
return this.month;
}
public double getStartingBalance(){
return this.startingBalance;
}
public double getEndingBalance(){
return this.endingBalance;
}
public double getAmountSaved(){
return this.amountSaved;
}
//Declare class methods
//Method that calculates how much money was saved
public double monthSaving(){
//CALCULATE THE AMOUNT OF MONEY SAVED FOR THE MONTH
return this.endingBalance - startingBalance;
}
public void print(){
System.out.println("Month:" + this.month + ", Starting bal.:$" + String.format("%.2f", this.startingBalance) + ", Ending bal.:$" + String.format("%.2f",this.endingBalance) + ", Amt. Saved:$"+ String.format("%.2f",getAmountSaved()));
}
}
MY main.java CODE
import java.io.File; // Import the File class
import java.io.FileNotFoundException; // Import this class to handle errors
import java.util.Scanner; // Import the Scanner class to read text files
import java.util.ArrayList; // import the ArrayList class
class Main {
public static void main(String[] args) {
String month;
double startingBalance;
double endingBalance;
System.out.println("Welcome");
try {
File myObj = new File("Savings.csv");
Scanner myReader = new Scanner(myObj);
while (myReader.hasNextLine()) {
String data = myReader.nextLine();
//seperate into substrings using split function
String values[] = data.split(",");
//Values of month, startingBalance and endingBalance are stored
month = values[0];
startingBalance = Double.parseDouble(values[1]);
endingBalance = Double.parseDouble(values[2]);
//Creates instance for Record
Record recordData = new Record(month, startingBalance, endingBalance);
recordData.print();
}
myReader.close();
}
catch (FileNotFoundException e) {
System.out.println("An error occurred.");
e.printStackTrace();
}
}
}
MY ERROR
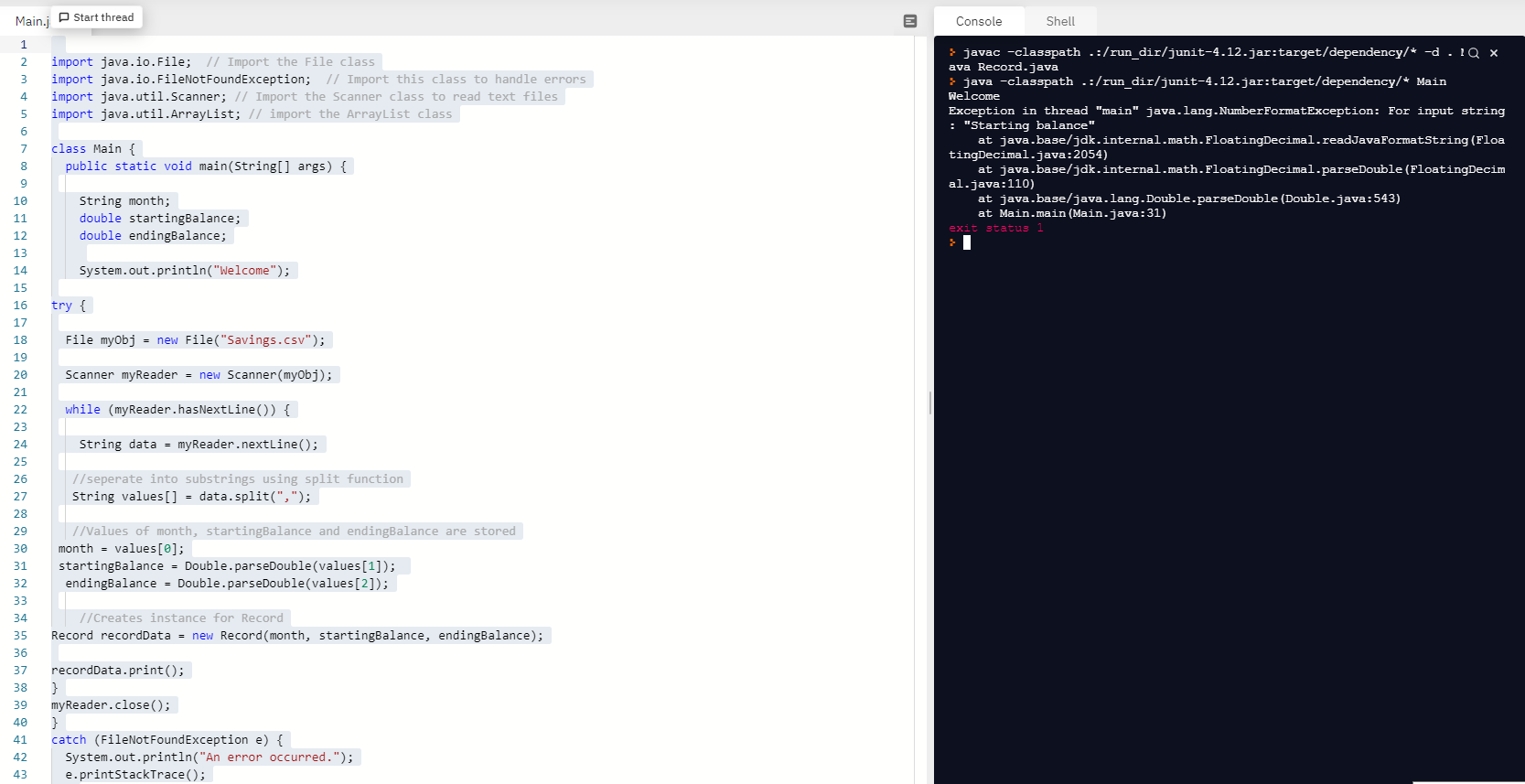
Question 2 20 pts You now have each line of the file reading in as a single string. We would like to take each line of values and store them in an instance of the class Record. You would notice that the constructor for Record takes one string and two doubles. In order to use each of line of values in the constructor we are going to have to split the line into separate values and convert the last two values into doubles. I have written an example here that takes the first line of the file and and splits it into an array of strings using the String split methode in Java. I then use the parseDouble method in the Double wrapper class. 1. Read in each line. 2. Declare an instance of a Record using the constructor and the values from the split string. 3. Print that record using its print function. When you are finished copy and paste your main into the text box below. Edit View Insert Format Tools Table 12pt v Paragraph T? v Main.j Start thread Console Shell 2 3 import java.io.File; // Import the File class import java.io.FileNotFoundException; // Import this class to handle errors import java.util.Scanner; // Import the Scanner class to read text files import java.util.ArrayList; // import the ArrayList class 4 5 6 7 class Main { public static void main(String[] args) { javac -classpath .:/run_dir/junit-4.12.jar:target/dependency/* -d.IQ X ava Record.java > java -classpath . :/run_dir/junit-4.12.jar:target/dependency/* Main Welcome Exception in thread "main" java.lang.Number FormatException: For input string : "Starting balance" at java.base/jdk.internal.math.FloatingDecimal.readJavaFormatString (Floa tingDecimal.java:2054) at java.base/jdk.internal.math.FloatingDecimal.parseDouble (FloatingDecim al.java:110) at java.base/java.lang.Double.parseDouble (Double.java:543) at Main-main (Main.java:31) exit status 9 10 11 String month; double startingBalance; double endingBalance; 12 13 14 15 System.out.println("Welcome"); try { 16 17 18 File myobj = new File("Savings.csv"); 19 20 Scanner myReader = new Scanner(myObj); 21 22 while (myReader.hasNextLine()) { 23 String data = myReader.nextLine(); 24 25 26 1/ seperate into substrings using split function String values[] = data.split(","); 27 28 29 30 1/Values of month, startingBalance and endingBalance are stored month = values[@]; startingBalance = Double.parseDouble(values[1]); endingBalance - Double.parseDouble(values[2]); 31 32 33 34 // Creates instance for Record Record recordData = new Record(month, startingBalance, endingBalance); 35 36 37 38 39 40 41 recordData.print(); } my Reader.close(); } catch (FileNotFoundException e) { System.out.println("An error occurred."); e.printStackTrace(); 42 43 Question 2 20 pts You now have each line of the file reading in as a single string. We would like to take each line of values and store them in an instance of the class Record. You would notice that the constructor for Record takes one string and two doubles. In order to use each of line of values in the constructor we are going to have to split the line into separate values and convert the last two values into doubles. I have written an example here that takes the first line of the file and and splits it into an array of strings using the String split methode in Java. I then use the parseDouble method in the Double wrapper class. 1. Read in each line. 2. Declare an instance of a Record using the constructor and the values from the split string. 3. Print that record using its print function. When you are finished copy and paste your main into the text box below. Edit View Insert Format Tools Table 12pt v Paragraph T? v Main.j Start thread Console Shell 2 3 import java.io.File; // Import the File class import java.io.FileNotFoundException; // Import this class to handle errors import java.util.Scanner; // Import the Scanner class to read text files import java.util.ArrayList; // import the ArrayList class 4 5 6 7 class Main { public static void main(String[] args) { javac -classpath .:/run_dir/junit-4.12.jar:target/dependency/* -d.IQ X ava Record.java > java -classpath . :/run_dir/junit-4.12.jar:target/dependency/* Main Welcome Exception in thread "main" java.lang.Number FormatException: For input string : "Starting balance" at java.base/jdk.internal.math.FloatingDecimal.readJavaFormatString (Floa tingDecimal.java:2054) at java.base/jdk.internal.math.FloatingDecimal.parseDouble (FloatingDecim al.java:110) at java.base/java.lang.Double.parseDouble (Double.java:543) at Main-main (Main.java:31) exit status 9 10 11 String month; double startingBalance; double endingBalance; 12 13 14 15 System.out.println("Welcome"); try { 16 17 18 File myobj = new File("Savings.csv"); 19 20 Scanner myReader = new Scanner(myObj); 21 22 while (myReader.hasNextLine()) { 23 String data = myReader.nextLine(); 24 25 26 1/ seperate into substrings using split function String values[] = data.split(","); 27 28 29 30 1/Values of month, startingBalance and endingBalance are stored month = values[@]; startingBalance = Double.parseDouble(values[1]); endingBalance - Double.parseDouble(values[2]); 31 32 33 34 // Creates instance for Record Record recordData = new Record(month, startingBalance, endingBalance); 35 36 37 38 39 40 41 recordData.print(); } my Reader.close(); } catch (FileNotFoundException e) { System.out.println("An error occurred."); e.printStackTrace(); 42 43