Question
I did this code but when I run it , it shows me this error: Error: Could not find or load main class projectPhase2.Update **
I did this code but when I run it , it shows me this error: Error: Could not find or load main class projectPhase2.Update
** what should I do ? , or what is the error I am doing ? -- I think there is something missing but I cannot recognize it
Update Jframe :
package projectPhase2;
import java.awt.BorderLayout; import java.awt.EventQueue;
import javax.swing.JFrame; import javax.swing.JPanel; import javax.swing.border.EmptyBorder; import java.awt.Window.Type; import javax.swing.JMenuBar; import javax.swing.JMenu; import javax.swing.JSeparator; import javax.swing.JCheckBoxMenuItem; import javax.swing.JButton; import java.awt.Font; import javax.swing.JEditorPane; import javax.swing.JComboBox; import javax.swing.JPopupMenu; import java.awt.Component; import java.awt.event.MouseAdapter; import java.awt.event.MouseEvent; import javax.swing.JRadioButtonMenuItem; import javax.swing.JTree; import javax.swing.JList; import javax.swing.JMenuItem; import javax.swing.JOptionPane; import javax.swing.JScrollBar; import javax.swing.JSpinner; import javax.swing.SpinnerNumberModel; import java.awt.event.ActionListener; import java.awt.event.ActionEvent; import javax.swing.JTextField; import javax.swing.JLabel; import javax.swing.JToggleButton; import javax.swing.JRadioButton; import javax.swing.GroupLayout; import javax.swing.GroupLayout.Alignment; import java.awt.Choice;
public class Update extends JFrame {
private JPanel contentPane; private JTextField textField; private JTextField textField_1; private JTextField textField_2; private JTextField textField_3; private JTextField textField_4; private DBase db = new DBase();
/** * Launch the application. */ public static void main(String[] args) { EventQueue.invokeLater(new Runnable() { public void run() { try { Update frame = new Update(); frame.setVisible(true); } catch (Exception e) { e.printStackTrace(); } } }); }
/** * Create the frame. */ public Update() { setTitle("Update"); setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); setBounds(100, 100, 554, 537); contentPane = new JPanel(); contentPane.setToolTipText("Quantity"); contentPane.setBorder(new EmptyBorder(5, 5, 5, 5)); setContentPane(contentPane); JLabel lblQuantity = new JLabel("Item :"); lblQuantity.setBounds(10, 18, 103, 35); lblQuantity.setFont(new Font("Tahoma", Font.BOLD, 21)); JLabel lblCustomerInformation = new JLabel("Customer Information :"); lblCustomerInformation.setBounds(10, 106, 250, 26); lblCustomerInformation.setFont(new Font("Tahoma", Font.BOLD, 21)); JLabel lblOrder = new JLabel("Order :"); lblOrder.setBounds(10, 260, 92, 26); lblOrder.setFont(new Font("Tahoma", Font.BOLD, 21)); JLabel lblQuantity_1 = new JLabel("Quantity"); lblQuantity_1.setBounds(20, 61, 92, 26); JSpinner spinner = new JSpinner(); spinner.setBounds(194, 58, 59, 32); spinner.setModel(new SpinnerNumberModel(0, 0, 100, 1)); spinner.setToolTipText(""); JLabel lblEmail = new JLabel("Email"); lblEmail.setBounds(21, 146, 92, 26); textField = new JTextField(); textField.setBounds(194, 143, 250, 32); textField.setColumns(10); JLabel lblPhoneNo = new JLabel("Phone No."); lblPhoneNo.setBounds(21, 184, 116, 26); textField_1 = new JTextField(); textField_1.setBounds(194, 181, 250, 32); textField_1.setColumns(10); JLabel lblBankName = new JLabel("Bank Name"); lblBankName.setBounds(21, 220, 116, 26); textField_2 = new JTextField(); textField_2.setBounds(194, 217, 250, 32); textField_2.setColumns(10); JLabel lblDelivaryDate = new JLabel("Delivary Date"); lblDelivaryDate.setBounds(20, 298, 134, 26); textField_3 = new JTextField(); textField_3.setBounds(194, 295, 250, 32); textField_3.setColumns(10); JLabel lblAddress = new JLabel("Address"); lblAddress.setBounds(21, 340, 92, 26); textField_4 = new JTextField(); textField_4.setBounds(194, 337, 250, 32); textField_4.setColumns(10); JButton btnApply = new JButton("Apply"); btnApply.setBounds(66, 415, 177, 35); btnApply.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent arg0) { int Quantity = spinner.getComponentCount(); String Email = textField.getText(); int PhoneNo= Integer.parseInt(textField_1.getText()); String BankName= textField_2.getText(); String DelivaryDate = textField_3.getText(); String Address = textField_4.getText(); db.Update(Quantity, Email, PhoneNo, BankName, DelivaryDate, Address); System.out.println("Quantity"+""+"Email"+""+"PhoneNo"+""+"BankName"+""+"DelivaryDate"+""+"Address"); } }); JButton btnExit = new JButton("Exit"); btnExit.setBounds(326, 415, 141, 35); btnExit.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent arg0) { dispose(); } }); contentPane.setLayout(null); contentPane.add(lblQuantity); contentPane.add(lblCustomerInformation); contentPane.add(lblOrder); contentPane.add(lblQuantity_1); contentPane.add(spinner); contentPane.add(lblEmail); contentPane.add(textField); contentPane.add(lblPhoneNo); contentPane.add(textField_1); contentPane.add(lblBankName); contentPane.add(textField_2); contentPane.add(lblDelivaryDate); contentPane.add(textField_3); contentPane.add(lblAddress); contentPane.add(textField_4); contentPane.add(btnApply); contentPane.add(btnExit); } private static void addPopup(Component component, final JPopupMenu popup) { component.addMouseListener(new MouseAdapter() { public void mousePressed(MouseEvent e) { if (e.isPopupTrigger()) { showMenu(e); } } public void mouseReleased(MouseEvent e) { if (e.isPopupTrigger()) { showMenu(e); } } private void showMenu(MouseEvent e) { popup.show(e.getComponent(), e.getX(), e.getY()); } }); } }
DBase class :
package projectPhase2;
import java.sql.Connection; import java.sql.Date; import java.sql.DriverManager; import java.sql.PreparedStatement; import java.sql.ResultSet; import java.sql.SQLException; import java.sql.Statement; import java.util.ArrayList; import java.util.Locale;
public class DBase { private Connection conn; private Statement stmt; public DBase() { super(); try { conn = DriverManager.getConnection("jdbc:oracle:thin:@coestudb.qu.edu.qa:1521/STUD.qu.edu.qa", "aa1300228","aa1300228"); stmt = conn.createStatement(); } catch (SQLException e) { // TODO Auto-generated catch block e.printStackTrace(); } } public void insertCustomer(int phoneNumber,String BankName,String Name, String Email){ String sql = "insert into customerrr values(?,?,?,?)"; try { PreparedStatement st = conn.prepareStatement(sql); st.setInt(1, phoneNumber);st.setString(2, BankName);st.setString(3, Name);st.setString(4, Email); st.executeUpdate(); st.close(); } catch (SQLException e) { // TODO Auto-generated catch block e.printStackTrace(); } } public void insertCustInfo(int phoneNumber, int AccountNumber){ String sql = "insert into CustomerInfo values(?,?)"; try { PreparedStatement st = conn.prepareStatement(sql); st.setInt(1, phoneNumber);st.setInt(2, AccountNumber); st.executeUpdate(); st.close(); } catch (SQLException e) { // TODO Auto-generated catch block e.printStackTrace(); } } public void insertOnlineRetail(int CustomerID, int phoneNumber){ String sql = "insert into OnlineRetail values(?,?)"; try { PreparedStatement st = conn.prepareStatement(sql); st.setInt(1, CustomerID);st.setInt(2, phoneNumber); st.executeUpdate(); st.close(); } catch (SQLException e) { // TODO Auto-generated catch block e.printStackTrace(); } } public void insertPurcahse(int CustomerID, int ItemNumber, int Quantity){ String sql = "insert into Purcahse values(?,?,?)"; try { PreparedStatement st = conn.prepareStatement(sql); st.setInt(1, CustomerID);st.setInt(2, ItemNumber);st.setInt(3, Quantity); st.executeUpdate(); st.close(); } catch (SQLException e) { // TODO Auto-generated catch block e.printStackTrace(); } } // updating an order public void Update(int Quantity, String Email, int PhoneNo, String bankName, String delivaryDate, String address) { String sql = " update values ( ?,?,?,?,?,?) "; try { PreparedStatement st = conn.prepareStatement(sql); st.setInt(1, Quantity); st.setString(2, Email); st.setInt(3, PhoneNo); st.setString(4, bankName); st.setString(5, delivaryDate); st.setString(6, address); st.executeUpdate(); st.close(); } catch (SQLException e) { // TODO Auto-generated catch block e.printStackTrace(); }
} public ArrayList getAllItems(){ ArrayList temp = new ArrayList<>() ; try { Statement st = conn.createStatement(); ResultSet rs = st.executeQuery("select * from Itemm"); while (rs.next()) temp.add(rs.getInt(1)+" "+rs.getInt(2)); } catch (SQLException e) { // TODO Auto-generated catch block e.printStackTrace(); } return temp; } public void close(){ try { conn.close(); stmt.close(); } catch (SQLException e) { // TODO Auto-generated catch block e.printStackTrace(); } } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
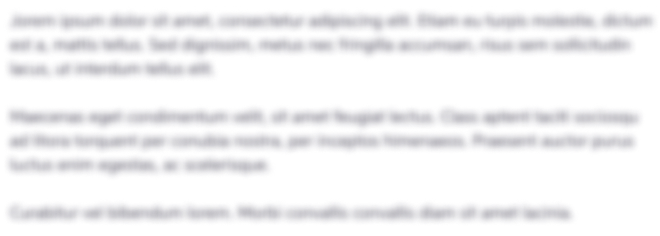
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started