Question
I dont need help with this anymore. I was able to figure it out and get the program compiling and running. Thanks for attempting to
I dont need help with this anymore. I was able to figure it out and get the program compiling and running. Thanks for attempting to help!
MAIN
#include #include
#include "Executive.cpp"
int main(int argc, char const *argv[]) {
Executive exec; exec.run("input.txt"); return 0; }
EXECUTIVE.H - OPENS FILE AND CALLS LINKEDLIST TO MAKE THE LIST
#ifndef EXECUTIVE_H #define EXECUTIVE_H #include "LinkedListExample.hpp" class Executive { private: string filename; public: Executive(); void run(string); }; #endif
EXECUTIVE.CPP
#include "Executive.h"
Executive::Executive(){ string filename;} void Executive::run(string filename){ string command, website; ifstream file; //LinkedList* websites = new LinkedList(); LinkedList* websites = new LinkedList(); file.open(filename);
while(file){ file >> command; if(command == "NAVIGATE"){ file >> website; websites->add(website); } else if(command == "BACK"){ websites->back(); } else if(command == "FORWARD"){ websites->forward(); } else if(command == "HISTORY"){ websites->print(); } command = " "; } }
LINKEDLIST.H - USES NODE.H TO POPULATE THE LIST
#ifndef LINKEDLISTEXAMPLE_H #define LINKEDLISTEXAMPLE_H
#include "node.h" using namespace std;
class LinkedList { private: int length, backUp; public: Node *head, *tail, *curPtr; LinkedList(); ~LinkedList(); void add(string); void forward(); void back(); void remove(int); void clear(); bool isEmpty() const; int getLength() const; void print(); }; #endif
LINKEDLIST.CPP
#include "LinkedListExample.h"
using namespace std;
LinkedList::LinkedList(){
this->length = 0;
this->head = NULL;
this->tail = NULL;
backUp = 1;
}
void LinkedList::add(string website){
Node *temp = new Node;
temp->data = website;
temp->prev = NULL;
temp->next = NULL;
if(head == NULL){
head = temp;
tail = temp;
temp = NULL;
curPtr = head;
}
else {
tail->prev = curPtr;
curPtr->next = temp;
curPtr = temp;
}
this->length++;
}
void LinkedList::forward(){
if (curPtr->next != NULL)
curPtr = curPtr->next;
}
void LinkedList::back(){
Node* temp = new Node;
temp = head;
for(int i = 1;i < this->length-backUp;i++){
temp = temp->next;
}
curPtr = temp;
if (curPtr->prev != NULL){
curPtr = curPtr->prev;
this->length--;
}
backUp++;
}
void LinkedList::remove(int pos){
if (pos > this->length)
{
cout << "Position does not exist";
return;
}
Node *temp = new Node;
temp = tail;
for (int i = 1; i < pos; i++)
{
temp = temp->next;
}
if (temp != NULL)
{
temp->prev->next = temp->next;
temp->next->prev = temp->prev;
}
}
int LinkedList::getLength() const{
return this->length;
}
bool LinkedList::isEmpty() const{
if(this->length == 0){
return 1;
}
else{
return 0;
}
}
void LinkedList::print(){
Node *temp = new Node;
temp = head;
cout << " Oldest" << endl;
cout << "==============" << endl;
while(temp != nullptr){
if(curPtr->data == temp->data){
cout << temp->data << " <== Current" << endl;
temp = temp->next;
}
else{
cout << temp->data << endl;
temp = temp->next;
}
}
cout << "==============" << endl;
cout << " Newest" << " " << endl;
}
void LinkedList::clear(){
}
LinkedList::~LinkedList(){
delete head;
delete tail;
std::cout << "LIST DELETED";
}
NODE.H
#ifndef NODE_H #define NODE_H
class Node { public: Node *next, *prev; std::string data; }; #endif
MAKEFILE
program: main.o Executive.o LinkedList.o
g++ -std=c++11 -g -Wall main.o Executive.o LinkedList.o -o program
main.o: main.cpp Executive.cpp
g++ -std=c++11 -g -Wall -c main.cpp
Executive.o: Executive.h Executive.cpp LinkedList.cpp
g++ -std=c++11 -g -Wall -c Executive.cpp
LinkedList.o: LinkedList.h LinkedList.cpp
g++ -std=c++11 -g -Wall -c LinkedList.cpp
clean:
rm *.o program
LIST OF ERRORS - ALL VERY SIMILIAR
g++ -std=c++11 -g -Wall -c main.cpp g++ -std=c++11 -g -Wall -c Executive.cpp g++ -std=c++11 -g -Wall -c LinkedList.cpp g++ -std=c++11 -g -Wall main.o Executive.o LinkedList.o -o program Executive.o: In function `Node::~Node()': /home/b188c785/EECS 268/Lab 2/drive-download-20180916T180232Z-001/LinkedList.cpp:7: multiple definition of `LinkedList::LinkedList()' main.o:/home/b188c785/EECS 268/Lab 2/drive-download-20180916T180232Z-001/LinkedList.cpp:7: first defined here Executive.o: In function `Node::~Node()': /home/b188c785/EECS 268/Lab 2/drive-download-20180916T180232Z-001/LinkedList.cpp:7: multiple definition of `LinkedList::LinkedList()' main.o:/home/b188c785/EECS 268/Lab 2/drive-download-20180916T180232Z-001/LinkedList.cpp:7: first defined here Executive.o: In function `std::char_traits::compare(char const*, char const*, unsigned long)': /home/b188c785/EECS 268/Lab 2/drive-download-20180916T180232Z-001/LinkedList.cpp:13: multiple definition of `LinkedList::add(std::__cxx11::basic_string, std::allocator >)' main.o:/home/b188c785/EECS 268/Lab 2/drive-download-20180916T180232Z-001/LinkedList.cpp:13: first defined here Executive.o: In function `LinkedList::forward()': /home/b188c785/EECS 268/Lab 2/drive-download-20180916T180232Z-001/LinkedList.cpp:31: multiple definition of `LinkedList::forward()' main.o:/home/b188c785/EECS 268/Lab 2/drive-download-20180916T180232Z-001/LinkedList.cpp:31: first defined here Executive.o: In function `LinkedList::back()': /home/b188c785/EECS 268/Lab 2/drive-download-20180916T180232Z-001/LinkedList.cpp:35: multiple definition of `LinkedList::back()' main.o:/home/b188c785/EECS 268/Lab 2/drive-download-20180916T180232Z-001/LinkedList.cpp:35: first defined here Executive.o: In function `LinkedList::remove(int)': /home/b188c785/EECS 268/Lab 2/drive-download-20180916T180232Z-001/LinkedList.cpp:49: multiple definition of `LinkedList::remove(int)' main.o:/home/b188c785/EECS 268/Lab 2/drive-download-20180916T180232Z-001/LinkedList.cpp:49: first defined here Executive.o: In function `LinkedList::getLength() const': /home/b188c785/EECS 268/Lab 2/drive-download-20180916T180232Z-001/LinkedList.cpp:67: multiple definition of `LinkedList::getLength() const' main.o:/home/b188c785/EECS 268/Lab 2/drive-download-20180916T180232Z-001/LinkedList.cpp:67: first defined here Executive.o: In function `LinkedList::isEmpty() const': /home/b188c785/EECS 268/Lab 2/drive-download-20180916T180232Z-001/LinkedList.cpp:70: multiple definition of `LinkedList::isEmpty() const' main.o:/home/b188c785/EECS 268/Lab 2/drive-download-20180916T180232Z-001/LinkedList.cpp:70: first defined here Executive.o: In function `LinkedList::print()': /home/b188c785/EECS 268/Lab 2/drive-download-20180916T180232Z-001/LinkedList.cpp:78: multiple definition of `LinkedList::print()' main.o:/home/b188c785/EECS 268/Lab 2/drive-download-20180916T180232Z-001/LinkedList.cpp:78: first defined here Executive.o: In function `LinkedList::clear()': /home/b188c785/EECS 268/Lab 2/drive-download-20180916T180232Z-001/LinkedList.cpp:98: multiple definition of `LinkedList::clear()' main.o:/home/b188c785/EECS 268/Lab 2/drive-download-20180916T180232Z-001/LinkedList.cpp:98: first defined here Executive.o: In function `LinkedList::~LinkedList()': /home/b188c785/EECS 268/Lab 2/drive-download-20180916T180232Z-001/LinkedList.cpp:101: multiple definition of `LinkedList::~LinkedList()' main.o:/home/b188c785/EECS 268/Lab 2/drive-download-20180916T180232Z-001/LinkedList.cpp:101: first defined here Executive.o: In function `LinkedList::~LinkedList()': /home/b188c785/EECS 268/Lab 2/drive-download-20180916T180232Z-001/LinkedList.cpp:101: multiple definition of `LinkedList::~LinkedList()' main.o:/home/b188c785/EECS 268/Lab 2/drive-download-20180916T180232Z-001/LinkedList.cpp:101: first defined here Executive.o: In function `Executive::Executive()': /home/b188c785/EECS 268/Lab 2/drive-download-20180916T180232Z-001/Executive.cpp:5: multiple definition of `Executive::Executive()' main.o:/home/b188c785/EECS 268/Lab 2/drive-download-20180916T180232Z-001/Executive.cpp:5: first defined here Executive.o: In function `Executive::Executive()': /home/b188c785/EECS 268/Lab 2/drive-download-20180916T180232Z-001/Executive.cpp:5: multiple definition of `Executive::Executive()' main.o:/home/b188c785/EECS 268/Lab 2/drive-download-20180916T180232Z-001/Executive.cpp:5: first defined here Executive.o: In function `Executive::run(std::__cxx11::basic_string, std::allocator >)': /home/b188c785/EECS 268/Lab 2/drive-download-20180916T180232Z-001/Executive.cpp:8: multiple definition of `Executive::run(std::__cxx11::basic_string, std::allocator >)' main.o:/home/b188c785/EECS 268/Lab 2/drive-download-20180916T180232Z-001/Executive.cpp:8: first defined here LinkedList.o: In function `Node::~Node()': /home/b188c785/EECS 268/Lab 2/drive-download-20180916T180232Z-001/LinkedList.cpp:7: multiple definition of `LinkedList::LinkedList()' main.o:/home/b188c785/EECS 268/Lab 2/drive-download-20180916T180232Z-001/LinkedList.cpp:7: first defined here LinkedList.o: In function `Node::~Node()': /home/b188c785/EECS 268/Lab 2/drive-download-20180916T180232Z-001/LinkedList.cpp:7: multiple definition of `LinkedList::LinkedList()' main.o:/home/b188c785/EECS 268/Lab 2/drive-download-20180916T180232Z-001/LinkedList.cpp:7: first defined here LinkedList.o: In function `std::char_traits::compare(char const*, char const*, unsigned long)': /home/b188c785/EECS 268/Lab 2/drive-download-20180916T180232Z-001/LinkedList.cpp:13: multiple definition of `LinkedList::add(std::__cxx11::basic_string, std::allocator >)' main.o:/home/b188c785/EECS 268/Lab 2/drive-download-20180916T180232Z-001/LinkedList.cpp:13: first defined here LinkedList.o: In function `LinkedList::forward()': /home/b188c785/EECS 268/Lab 2/drive-download-20180916T180232Z-001/LinkedList.cpp:31: multiple definition of `LinkedList::forward()' main.o:/home/b188c785/EECS 268/Lab 2/drive-download-20180916T180232Z-001/LinkedList.cpp:31: first defined here LinkedList.o: In function `LinkedList::back()': /home/b188c785/EECS 268/Lab 2/drive-download-20180916T180232Z-001/LinkedList.cpp:35: multiple definition of `LinkedList::back()' main.o:/home/b188c785/EECS 268/Lab 2/drive-download-20180916T180232Z-001/LinkedList.cpp:35: first defined here LinkedList.o: In function `LinkedList::remove(int)': /home/b188c785/EECS 268/Lab 2/drive-download-20180916T180232Z-001/LinkedList.cpp:49: multiple definition of `LinkedList::remove(int)' main.o:/home/b188c785/EECS 268/Lab 2/drive-download-20180916T180232Z-001/LinkedList.cpp:49: first defined here LinkedList.o: In function `LinkedList::getLength() const': /home/b188c785/EECS 268/Lab 2/drive-download-20180916T180232Z-001/LinkedList.cpp:67: multiple definition of `LinkedList::getLength() const' main.o:/home/b188c785/EECS 268/Lab 2/drive-download-20180916T180232Z-001/LinkedList.cpp:67: first defined here LinkedList.o: In function `LinkedList::isEmpty() const': /home/b188c785/EECS 268/Lab 2/drive-download-20180916T180232Z-001/LinkedList.cpp:70: multiple definition of `LinkedList::isEmpty() const' main.o:/home/b188c785/EECS 268/Lab 2/drive-download-20180916T180232Z-001/LinkedList.cpp:70: first defined here LinkedList.o: In function `LinkedList::print()': /home/b188c785/EECS 268/Lab 2/drive-download-20180916T180232Z-001/LinkedList.cpp:78: multiple definition of `LinkedList::print()' main.o:/home/b188c785/EECS 268/Lab 2/drive-download-20180916T180232Z-001/LinkedList.cpp:78: first defined here LinkedList.o: In function `LinkedList::clear()': /home/b188c785/EECS 268/Lab 2/drive-download-20180916T180232Z-001/LinkedList.cpp:98: multiple definition of `LinkedList::clear()' main.o:/home/b188c785/EECS 268/Lab 2/drive-download-20180916T180232Z-001/LinkedList.cpp:98: first defined here LinkedList.o: In function `LinkedList::~LinkedList()': /home/b188c785/EECS 268/Lab 2/drive-download-20180916T180232Z-001/LinkedList.cpp:101: multiple definition of `LinkedList::~LinkedList()' main.o:/home/b188c785/EECS 268/Lab 2/drive-download-20180916T180232Z-001/LinkedList.cpp:101: first defined here LinkedList.o: In function `LinkedList::~LinkedList()': /home/b188c785/EECS 268/Lab 2/drive-download-20180916T180232Z-001/LinkedList.cpp:101: multiple definition of `LinkedList::~LinkedList()' main.o:/home/b188c785/EECS 268/Lab 2/drive-download-20180916T180232Z-001/LinkedList.cpp:101: first defined here collect2: error: ld returned 1 exit status makefile:2: recipe for target 'program' failed make: *** [program] Error 1
Step by Step Solution
There are 3 Steps involved in it
Step: 1
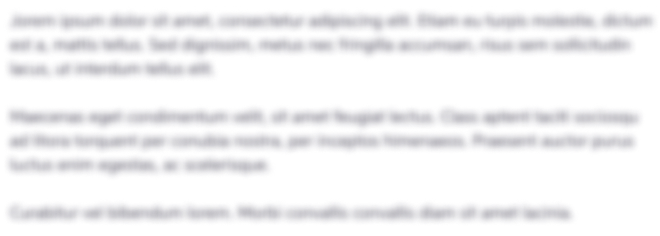
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started