Question
I figured out my code and it works. However, I need to develop 3 testcases to show how effective the error trapping. Make sure that
I figured out my code and it works. However, I need to develop 3 testcases to show how effective the error trapping. Make sure that you document the test cases errors.
You must indicate where you implement the error trapping structure and why.
My code is as follows:
#include
class FlCalc { public: void doCalc(double num1, double num2, char op) { switch (op) { case '+': add(num1, num2); break; case '-': subtract(num1, num2); break; case '*': multiply(num1, num2); break; case '/': divide(num1, num2); break; default: throw "Invalid operator!"; // throw an exception if the operator is invalid } } private: void add(double num1, double num2) { cout << "Addition: " << num1 + num2 << endl; } void subtract(double num1, double num2) { cout << "Subtraction: " << num1 - num2 << endl; } void multiply(double num1, double num2) { cout << "Multiplication: " << num1 * num2 << endl; } void divide(double num1, double num2) { if (num2 != 0) cout << "Division: " << num1 / num2 << endl; else throw "Error: Division by zero!"; // throw an exception if the denominator is zero } };
class FlCalc2 : public FlCalc { public: FlCalc allInOne(double num1, double num2) { FlCalc result; result.doCalc(num1, num2, '+'); result.doCalc(num1, num2, '-'); result.doCalc(num1, num2, '*'); result.doCalc(num1, num2, '/'); return result; } };
class Tools { public: void saveToFile(const string& filename, const string& content) { string test=filename; ofstream myfile(test.c_str()); if (myfile.is_open()) { myfile << content; myfile.close(); cout << "Data saved to file: " << filename << endl; } else { throw "Error: Unable to open file: " + filename; // throw an exception if the file cannot be opened } } string readFromFile(const string& filename) { string content; string test=filename; ifstream myfile(test.c_str()); if (myfile.is_open()) { string line; while (getline(myfile, line)) { content += line + " "; } myfile.close(); return content; } else { throw "Error: Unable to open file: " + filename; // throw an exception if the file cannot be opened } } };
int main() { string name; cout << "Enter name: "; getline(cin, name); string choice; while (true) { cout << " Menu: "; cout << "1. All-in-One Calculation "; cout << "2. Save to File "; cout << "3. Read from File "; cout << "4. Read Result File "; // Added menu item cout << "5. Exit "; cout << " Please choose: "; cin >> choice; try { // try block to test the code for exceptions if (choice == "1") { double num1, num2; cout << "Enter the first number: "; cin >> num1; cout << "Enter the second number: "; cin >> num2; FlCalc2 flCalc2; FlCalc result = flCalc2.allInOne(num1, num2); } else if (choice == "2") { string filename, content; cout << "Enter the filename: "; cin.ignore(); getline(cin, filename); cout << "Enter the content: "; getline(cin, content); Tools tools; tools.saveToFile(filename, content); } else if (choice == "3") { string filename; cout << "Enter the filename: "; cin.ignore(); getline(cin, filename); Tools tools; string content = tools.readFromFile(filename); if (!content.empty()) { cout << "Content of file " << filename << ": "; cout << content; } } else if (choice == "4") { string filename; cout << "Enter the result filename: "; cin.ignore(); getline(cin, filename); Tools tools; string content = tools.readFromFile(filename); if (!content.empty()) { cout << "Result content of file " << filename << ": "; cout << content; } } else if (choice == "5") { cout << name << ", Thank you for using the application. "; break; } else { throw "Invalid choice! Please try again. "; // throw an exception if the choice is invalid } } catch (const char* msg) { // catch block to handle the exceptions thrown by the try block cout << msg << endl; // print the error message } } return 0; }
With this code I have to develop three test cases to show how effective the error trapping is. I have to document the test case errors as well. Then I have to indicate where I implemented the error trapping structure and why. This all has to be done on Dev C++ program ide version 5.11. Any help is appreciated and screenshots of the test cases and error trapping would be helpful.
Thank you
Step by Step Solution
There are 3 Steps involved in it
Step: 1
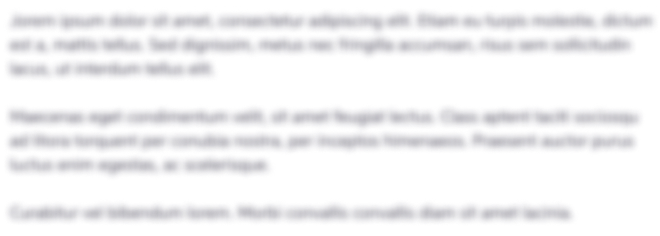
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started