Question
I have a PhoneBookDriver class that utilizes a GUI that accepts a name, address, and phone number. The code gathers these entries and uses them
I have a PhoneBookDriver class that utilizes a GUI that accepts a name, address, and phone number. The code gathers these entries and uses them to create a Person object and appends the object to a Binary Search Tree. Then the code prints the entryies in a ordered list within a text box in the GUI. The "Add" button works as intended, but I am unable to utilize the "Remove" button once it has been appended to the BST. Below is the code.
Within the remove action method, I have a simple test case, where if I add "Bob Clark" (within the GUI), then when I click remove the entire Bob Clark Person object should print to the terminal. The value, however, keeps returning as null. The BinarySearchTree class is correct. Below is my Person class and my PhoneBookDriver class.
PhoneBookDriver.java
package lab3; //Imports import javax.swing.*; import java.awt.event.ActionEvent; import java.awt.event.ActionListener; import java.io.Serializable; import java.util.Iterator; public class PhoneBookDriver implements Serializable { //GUI Parameters private JPanel mainPanel; private JPanel entryPanel; private JTextField textName; private JPanel buttonPanel; private JButton addButton; private JTextArea output; private JTextField textPhone; private JTextField textAddress; private JLabel title; private JLabel labelPhone; private JLabel labelAddress; private JButton removeButton; private JButton searchButton; private JLabel labelName; private JButton displayButton; //GUI Functionality public PhoneBookDriver() { //Initialize BST BinarySearchTreesearchTree = new BinarySearchTree<>(); //"ADD" Button addButton.addActionListener(new ActionListener() { @Override public void actionPerformed(ActionEvent e) { //new person object for each button press Person p = new Person(); //gather user input p.setName(textName.getText()); p.setAddress(textAddress.getText()); p.setPhoneNum(textPhone.getText()); searchTree.add(p.toString()); Iterator itr = searchTree.iterator(); //clear previous text output.setText(""); //print to GUI text field while (itr.hasNext()) { output.append(itr.next()); //Console Test //System.out.println(itr.next()); } } }); //"REMOVE" Button (TESTING) removeButton.addActionListener(new ActionListener() { @Override public void actionPerformed(ActionEvent e) { if (searchTree.contains("Bob Clark")); System.out.println(searchTree.getEntry("Bob Clark")); } }); //"SEARCH" Button searchButton.addActionListener(new ActionListener() { @Override public void actionPerformed(ActionEvent e) { String str = textName.getText(); if (str.isEmpty()) { str = textAddress.getText(); } if (str.isEmpty()) { str = textPhone.getText(); } output.setText(searchTree.getEntry(str).toString()); output.setText(str); } }); //"DISPLAY" Button (To revert back to main list after search) displayButton.addActionListener(new ActionListener() { @Override public void actionPerformed(ActionEvent e) { Iterator itr = searchTree.iterator(); //clear previous text output.setText(""); while (itr.hasNext()) { //print to GUI text field output.append(itr.next()); //Console Test //System.out.println(itr.next()); } } }); } //Main Method to support GUI integration public static void main(String[] args){ JFrame frame = new JFrame("PhoneBook Application"); frame.setContentPane(new PhoneBookDriver().mainPanel); frame.pack(); frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); frame.setVisible(true); } Person.java
package lab3; //Imports import java.io.*; public class Person implements Serializable{ //Initialize variables private String name; private String address; private String phoneNum; //Getters and setters public void setName(String name) { this.name = name; } public String getName() { return name; } public void setAddress(String address) { this.address = address; } public String getAddress() { return address; } public void setPhoneNum(String phoneNum) { this.phoneNum = phoneNum; } public String getPhoneNum() { return phoneNum; } @Override public String toString() { return "Name: " + getName() + " Address: " + getAddress() + " Phone Number: " + getPhoneNum() + " ************************* "; } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
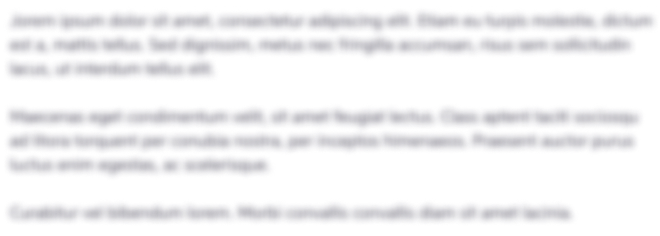
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started