Question
I have a question about printing template class in c++ programing. Using Point2D class, I want to print out the template class mylist as auto
I have a question about printing template class in c++ programing. Using Point2D class, I want to print out the template class mylist as auto x:mylist, but I keep failling.
Is there any way to solve this problem without touching any classes and using Point2D print function by ostream operator <
The answer should in the form if you enter two integers, then save it as Point2D, and print is as if Point2D (2,3), lastly, it should print out multiple Point2Ds without (-1,-1).
example, enter 2 3 5 6 -1 -1 , then the answer should be in the form of ((2,3),(5,6)) <-not including (-1,-1)
Please Help me!!
My code is below, if you need more code, I will happy to post it
#include #include #include #include #include
//declaration for Node, Iterator class; template class Node; template class Iterator; class Point2D;
template class List{ public: //constructor and destructor List(); ~List(); bool isEmpty();
//use data save it to list void push_back(T new_value); //instert s at iter position Iterator insert(Iterator iter, T new_value); //erase the position Iterator erase(Iterator iter); //reverse the list -problem 12.6 void reverse(); //push_front(int) -problem 12.7 void push_front(T new_value); //swap List -problem 12.8 void swap(List& other); //get size -problem12.9-10 int get_size(); //list begin & end Iterator begin(); Iterator end(); private: Node* first; Node* last; int size; //declare iterator class as friend friend class Iterator; friend class Point2D; // friend std::ostream& operator<<(std::ostream& os, const List& list); };
template class Node{ public: //constructor Node(); Node(T new_value); private: T data; //declare two objects of node class next and previous pointer Node *next; Node *previous; //declare the Iterator and class as friend friend class Iterator; friend class List; };
template class Iterator{ public: Iterator(); void set(T new_value); T operator*() const; Iterator& operator++(); Iterator operator++(int unused); Iterator& operator--(); Iterator operator--(int unused); bool operator ==(Iterator b)const; bool operator!=(Iterator b)const; private: Node* position; List* container; //declare the List as friend friend class List; };
/**List constructor @param first; @param last; @param size1; */ template List::List(){ //initialization first=NULL; last=NULL; size=0; } template List::~List(){ delete first; delete last; delete size; } template bool List::isEmpty() { if(first == NULL && last == NULL) //if the start pointer and end pointer are NULL then the list is empty return 1; else return 0; } template void List::push_back(T new_value){ Node* new_Node=new Node(new_value); if(last==NULL){ first=new_Node; last=new_Node; } else{ new_Node->previous=last; last->next=new_Node; last=new_Node; } ++size; } /**List push_front(int n) add an data at the front of the list assign it into memory first if first==NULL -> List is empty, push_back(data) else Node data next=first and previous becomes NULL, and first=new_node */ template void List::push_front(T new_value){ Node* new_Node=new Node(new_value); if(last==NULL) { first=new_Node; last=new_Node; } else{ new_Node->next=first; new_Node->previous=NULL; first=new_Node; } ++size; } template Iterator List::insert(Iterator iter, T new_value){ if(iter.position==NULL){ push_back(new_value); return; } Node* after =iter.position; Node* before =after->previous; Node* new_Node =new Node(new_value); new_Node->next=after; new_Node->previous=before; after->previous=new_Node; if(before==NULL)first=new_Node;//insert at beginning else before->next=new_Node; ++size; } template Iterator List::erase(Iterator iter){ assert(iter.position!=NULL); Node* remove=iter.position; Node* before=remove->previous; Node* after=remove->next; if(remove==first){ first=after; } else before->next=after; if(remove==last) last=before; else after->previous=before; delete remove; Iterator r; r.position=after; r.container=this; --size; return r; } //Reversing function algorithm template void List::reverse(){ if(this->first !=NULL){ Node* center=this->first; Node* start=center->next; Node* end=NULL; while(1){ center->next=end; center->previous=start; if(start==NULL) break; end=center; center=start; start=start->next; } this->first=center; } } //swap List other with current List using swap algorithm template void List::swap(List& other){ List temp; temp.first=other.first; other.first=this->first; this->first=temp.first; } //checking the size using for loop template int List::get_size(){ Iterator iter; int count=0; for(iter=this->begin();iter!=this->end();iter++){ count++; } return count; } template std::ostream& operator<<(std::ostream& os, const List& list) { }
//check begin() template Iterator List::begin(){ Iterator iter; iter.position=first; iter.container=this; return iter; } //check end() template Iterator List::end(){ Iterator iter; iter.position=NULL; iter.container=this; return iter; }
//class Node constructor template Node::Node(){ next=NULL; previous=NULL; data=0; } //constructor with integer s store it into data template Node::Node(T new_value){ data=new_value; next = NULL; previous = NULL; } //Iterator constructor template Iterator::Iterator(){ position = NULL; container = NULL; } template void Iterator::set(T new_value) { position->data = new_value; return; }
//Iterator operator * -> pointing the position template T Iterator::operator*()const { assert(position !=NULL); return position->data; }
//pre increment for operator++ template Iterator& Iterator::operator++() { assert(position!=NULL); position=position->next; return* this; } //post increment for operator++ template Iterator Iterator::operator++(int unused) { assert(position != NULL); auto clone(*this); ++(*this); return clone; } //pre increment for operator-- template Iterator& Iterator::operator--() { assert(position!=container->first); if(position==NULL) position =container->last; else position=position->previous; return* this; } //post increment for operator-- template Iterator Iterator::operator --(int unused){ auto clone(*this); --(*this); return clone; } //boolean operator to check == template bool Iterator::operator ==(Iterator b)const{ return position==b.position; } //boolean operator to check != template bool Iterator::operator!=(Iterator b)const{ return position!=b.position; } template void downsize(List& numbers){ Iterator iter; for (iter = numbers.begin(); iter != numbers.end();){ iter++; if (iter != numbers.end()) iter = numbers.erase(iter); } } template T maximum(List& numbers){ Iterator iter; iter=numbers.begin(); int max=*iter; for(;iter!=numbers.end();iter++){ if(*iter>max){ max=*iter; } } return max; } template void sort(List& l) { Iterator p1 = l.begin(); Iterator p2, index;
while(!(p1 == l.end())) { index = p1; p2 = p1; while(!(p2 == l.end())) { if(*p2 < *index) index = p2; ++p2; }
int temp = *p1; p1.set(*index); index.set(temp); ++p1; } }
template void Merge(List& numbers1, List& numbers2){ Iterator iter1; Iterator iter2; iter2 = numbers2.begin(); for (iter1 = ++numbers1.begin(); iter1 != numbers1.end(); iter1++){ if (iter2 != numbers2.end()){ numbers2.insert(iter1, *iter2); iter2++; } } for (; iter2 != numbers2.end(); iter2++){ numbers1.push_back(*iter2); } }
class Point2D {
public: // Constructors Point2D(); Point2D(double a, double b);
// Gets-rs double getx(); double gety();
// Set-rs void setx(double a); void sety(double b);
// Print functions virtual void print(); virtual void print(std::vector &point); //sort bool operator >(Point2D q) const; void sort(std::vector &point);
private: double x; double y; };
//point2D default constructors Point2D::Point2D() { x = 0; y = 0; return; } /**constructor with parameter @param a, @param b */ Point2D::Point2D(double a, double b) { x = a; y = b; return; }
//print x and y double Point2D::getx() { return x; } double Point2D::gety() { return y; } //set x=a,y=b void Point2D::setx(double a) { x = a; return; } void Point2D::sety(double b) { y = b; return; } //print x and y in user format void Point2D::print() { std::cout << "(" << x << "," << y << ")"; return; } //printing saved vector from 0 to vector size void Point2D::print(std::vector &point) { for (int i = 0; i < point.size(); i++) { point[i].print(); std::cout << " ";
} } bool Point2D::operator >(Point2D q) const{ double x1=q.getx(); double y1=q.gety(); double dis=x*x+y*y; double dist=x1*x1+y1*y1; double cos=x/dis; double cos1=x1/dist; bool op=false; if(dis>dist) op= true; else if(dis==dist){ if(y>0&&y1>0){ if(cos } else if(y>0&&y1<0)op=false; else if(y<0&&y1>0)op=true; else if(y==0){ if(x<0) op= true; } else{ if(cos>cos1)op=true; } } return op; } void Point2D::sort(std::vector &point) { int length = point.size();
for (int i = 0; i < length; ++i) { bool swapped = false; for (int j = 0; j < length - (i + 1); ++j) { if (point[j] > point[j+1]) { Point2D temp; temp.setx(point[j].getx()); temp.sety(point[j].gety()); point[j] = point[j+1]; point[j+1] = temp; swapped = true; } }
if (!swapped) break; } }
int main(){ int select1 = 0,select2=0, insert=0; List mylist; Iterator myiterator = mylist.begin(); std::cout << "Please input a set of nonnegative numbers for a list"; std::cout << " (Enter -1 when you are finished): " << std::endl;
//if select =-1 stop the loop while (select1 != -1&&select2 !=-1) { std::cin >> select1; std::cin >> select2; //since data is nonnegative set store the data if it is greater than 0 using push_back function if (select1>0||select2>0) mylist.push_back(Point2D(select1,select2)); } //printing the mylist followed by given format; std::cout << "Your list is"< for (auto x:mylist) std::cout << x << ","; std::cout <<'\b'<< ")"<
return 0;
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
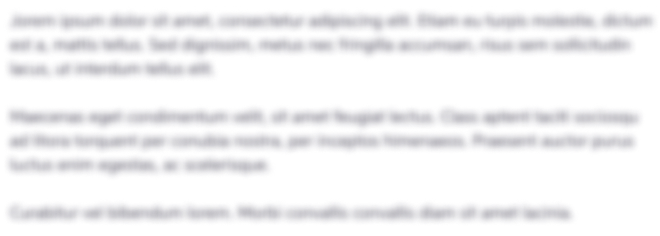
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started