Question
I have a Time class ( as below ) now I want to write a failing test: write a test method that will call our
I have a Time class ( as below )
now I want to write a failing test: write a test method that will call our getMilliseconds(String time) method and expect some valid result. For example, if we enter a time 12:05:05:05, we expect 5. Note that our failing test must still compile so we need a stub for getMilliseconds in our Time class.
and then write code to make the test pass
and take note : What else should we add to this method? What about checking the length of the String?
Time class in eclipse IDE
package time;
import java.util.Scanner; import javax.swing.JOptionPane;
public class Time { public static void main(String[] args) { try { String time = JOptionPane.showInputDialog(null, "Enter a time in the format hh:mm:ss", "Enter Time", JOptionPane.QUESTION_MESSAGE); int totalSeconds = getTotalSeconds(time); JOptionPane.showMessageDialog(null, totalSeconds, "Total Seconds", JOptionPane.INFORMATION_MESSAGE); } catch(StringIndexOutOfBoundsException e) { JOptionPane.showMessageDialog(null, "You entered the time in the wrong format. " + "Please enter the time in the form hh:mm:ss", "Invalid Time", JOptionPane.ERROR_MESSAGE); } catch(NumberFormatException e) { JOptionPane.showMessageDialog(null, "You entered an invalid time. Please enter numbers only.", "Invalid Time", JOptionPane.ERROR_MESSAGE); } catch(Exception e) { System.out.println("An unexpected Exception occurred"); } }
public static int getTotalSeconds(String time)throws NumberFormatException, StringIndexOutOfBoundsException { int hours = getTotalHours(time); //we will eventually multiply the hours by 3600 + the minutes by 60 + the seconds int minutes = getTotalMinutes (time); int seconds = getSeconds(time); return hours * 3600 + minutes * 60 + seconds; } public static int getSeconds(String time) throws NumberFormatException, StringIndexOutOfBoundsException { return Integer.parseInt(time.substring(6,8)); }
public static int getTotalMinutes(String time) throws NumberFormatException, StringIndexOutOfBoundsException { if (time.length()>8) { throw new NumberFormatException("your time was too long!"); } return Integer.parseInt(time.substring(3,5)); }
public static int getTotalHours(String time)throws NumberFormatException, StringIndexOutOfBoundsException { return Integer.parseInt(time.substring(0,2)); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
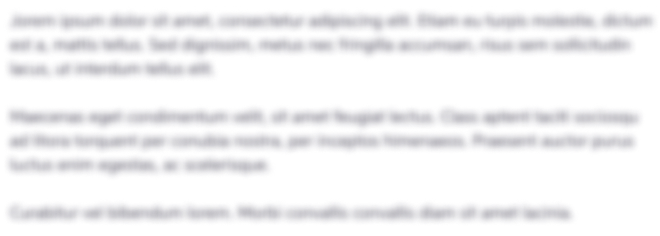
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started